Wrestling with the Complexity of New Parallelism APIs
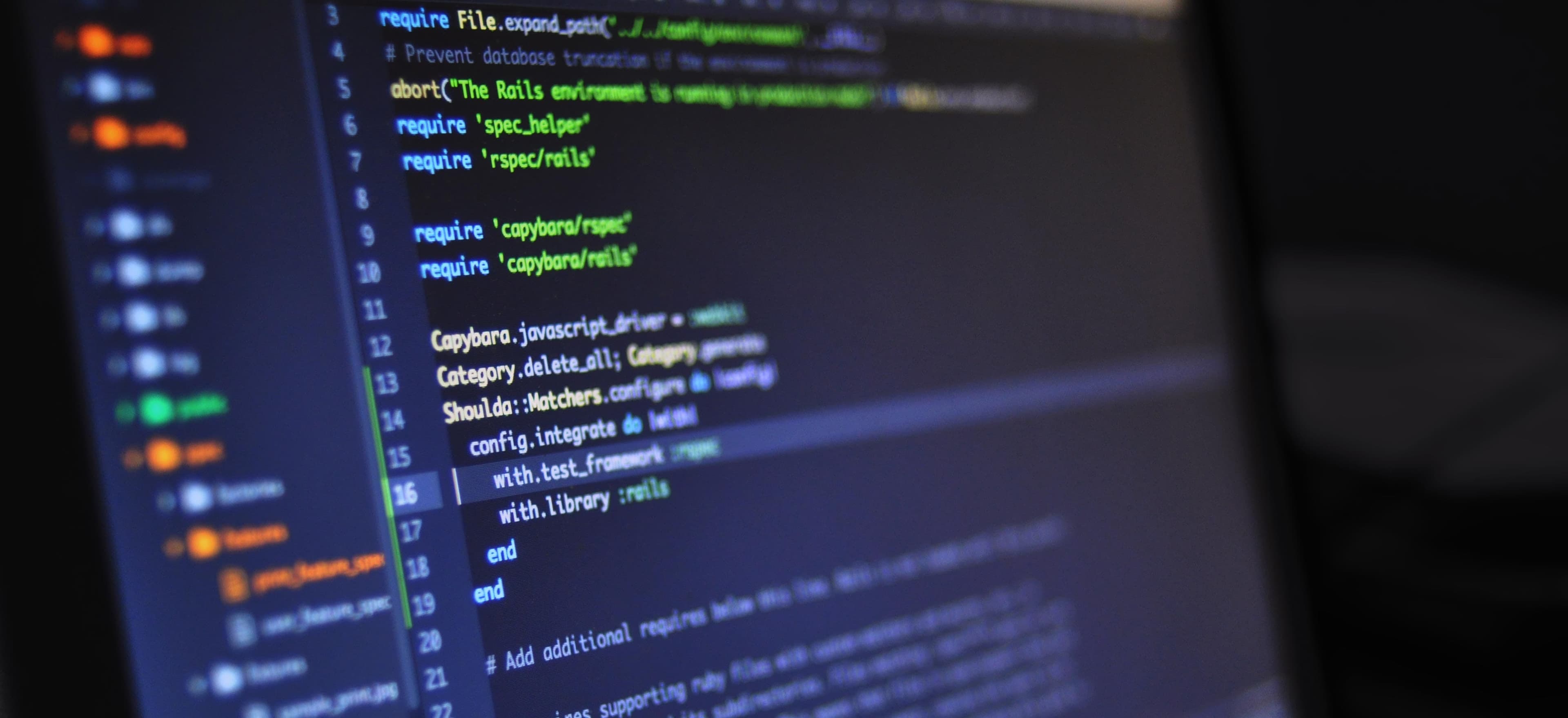
- Published on
Exploring the Complexity of Java’s New Parallelism APIs
In recent years, Java has evolved to meet the increasing demands for parallelism and concurrency in modern software development. With the introduction of new parallelism APIs, such as CompletableFuture and Executor framework, developers now have more powerful tools at their disposal. In this article, we will delve into the complexity of these new APIs and discuss how they can be effectively utilized in Java programming.
The Evolution of Parallelism in Java
Java has come a long way since the early days of multi-threading with the introduction of the java.util.concurrent
package. This package provided essential building blocks for concurrent programming, such as ExecutorService
, Future
, and ThreadPoolExecutor
. While these abstractions were powerful, they required developers to manage threads explicitly, leading to complex and error-prone code.
To address these challenges, Java 8 introduced the CompletableFuture API, which simplified asynchronous programming by allowing developers to compose and combine asynchronous operations. This was a significant step forward in the evolution of parallelism in Java, making it easier to write concurrent code without delving into the low-level details of thread management.
Understanding CompletableFuture
CompletableFuture
is a powerful class that represents a future result of an asynchronous computation. It allows developers to chain asynchronous operations together, define error handling, and orchestrate parallel computations with ease. Let's take a look at a simple example to illustrate the power of CompletableFuture
.
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello")
.thenApplyAsync(result -> result + " World")
.thenAcceptAsync(System.out::println);
future.join();
}
}
In this example, we create a CompletableFuture
using the supplyAsync
method, which performs a computation asynchronously. We then chain two more operations using the thenApplyAsync
and thenAcceptAsync
methods, which modify the result and print it to the console, respectively. Finally, we use the join
method to wait for the asynchronous computation to complete.
The beauty of CompletableFuture
lies in its ability to express complex asynchronous workflows in a fluent and composable manner. It simplifies the orchestration of parallel tasks and the handling of their results, making concurrent programming more approachable for developers.
Leveraging the Executor Framework
While CompletableFuture
provides a high-level abstraction for asynchronous programming, it relies on the underlying Executor framework for executing tasks in parallel. The Executor framework manages the execution of tasks on worker threads, abstracting away the complexities of thread creation and management.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.CompletableFuture;
public class ExecutorFrameworkExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> performTask(1), executor);
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> performTask(2), executor);
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(future1, future2);
combinedFuture.join();
executor.shutdown();
}
public static void performTask(int taskId) {
// Perform the task
}
}
In this example, we create an ExecutorService
with a fixed pool of two threads using Executors.newFixedThreadPool
. We then use CompletableFuture.runAsync
to execute two tasks asynchronously using the executor. Finally, we combine the two futures using CompletableFuture.allOf
to wait for both tasks to complete.
By leveraging the Executor framework in conjunction with CompletableFuture
, developers can control the execution context of asynchronous tasks and fine-tune the parallelism of their applications.
Navigating the Complexity
While the new parallelism APIs in Java provide powerful abstractions for concurrent programming, they also introduce complexity that developers must navigate. Understanding the intricacies of these APIs is crucial to harnessing their full potential and building reliable, high-performance applications.
One of the key challenges developers face is managing the lifecycle of asynchronous computations. It's essential to handle exceptions, timeouts, and resource cleanup properly to prevent resource leaks and ensure the stability of the application. Additionally, coordinating multiple asynchronous tasks and handling dependencies between them requires careful design and orchestration.
Furthermore, tuning the parallelism of asynchronous operations to match the characteristics of the underlying hardware is non-trivial. Oversaturating the system with too many concurrent tasks can lead to contention and degrade performance, while underutilizing available resources leaves potential performance gains untapped.
Best Practices for Utilizing Parallelism APIs
To effectively utilize the new parallelism APIs in Java, developers should adhere to best practices that promote maintainability, performance, and reliability.
1. Embrace Functional Composition
The functional programming capabilities of Java play a crucial role in simplifying the composition of asynchronous operations. Leveraging lambdas and method references makes it easier to express complex workflows concisely and clearly.
2. Use Custom Executors for Specialized Workloads
The Executor framework allows developers to create custom executors tailored to specific types of tasks. By designing executors with appropriate thread pool configurations, task scheduling policies, and thread factory settings, developers can optimize the performance of their asynchronous computations.
3. Handle Errors Explicitly
Proper error handling is essential in asynchronous programming. Whether it's propagating exceptions, recovering from failures, or composing fallback strategies, comprehensive error handling ensures the resilience of parallel computations.
4. Profile and Tune for Performance
Profiling the application's parallel execution and tuning the parallelism level based on empirical data is critical for achieving optimal performance. It's essential to strike a balance between the degree of parallelism and the characteristics of the workload to maximize throughput and minimize latency.
In Conclusion, Here is What Matters
The new parallelism APIs in Java, such as CompletableFuture and the Executor framework, have ushered in a new era of concurrent programming. While these APIs offer powerful abstractions for orchestrating parallel tasks, they also present challenges that require careful consideration and expertise to overcome.
By mastering the complexities of these APIs and adhering to best practices, developers can unlock the full potential of parallelism in Java and build resilient, high-performance applications that meet the demands of modern computing.
In conclusion, Java's new parallelism APIs empower developers to embrace the complexities of concurrent programming and wield the tools of parallelism with confidence and efficacy.
For further reading, refer to the official Java documentation on CompletableFuture and the Executor framework. Happy coding!
By incorporating the latest parallelism APIs in Java, developers can build high-performance applications that meet the demands of modern computing. If you want to learn more about Java and its capabilities, feel free to [Contact Us] for further information.