Streamlining Code with Polymorphism and Composition
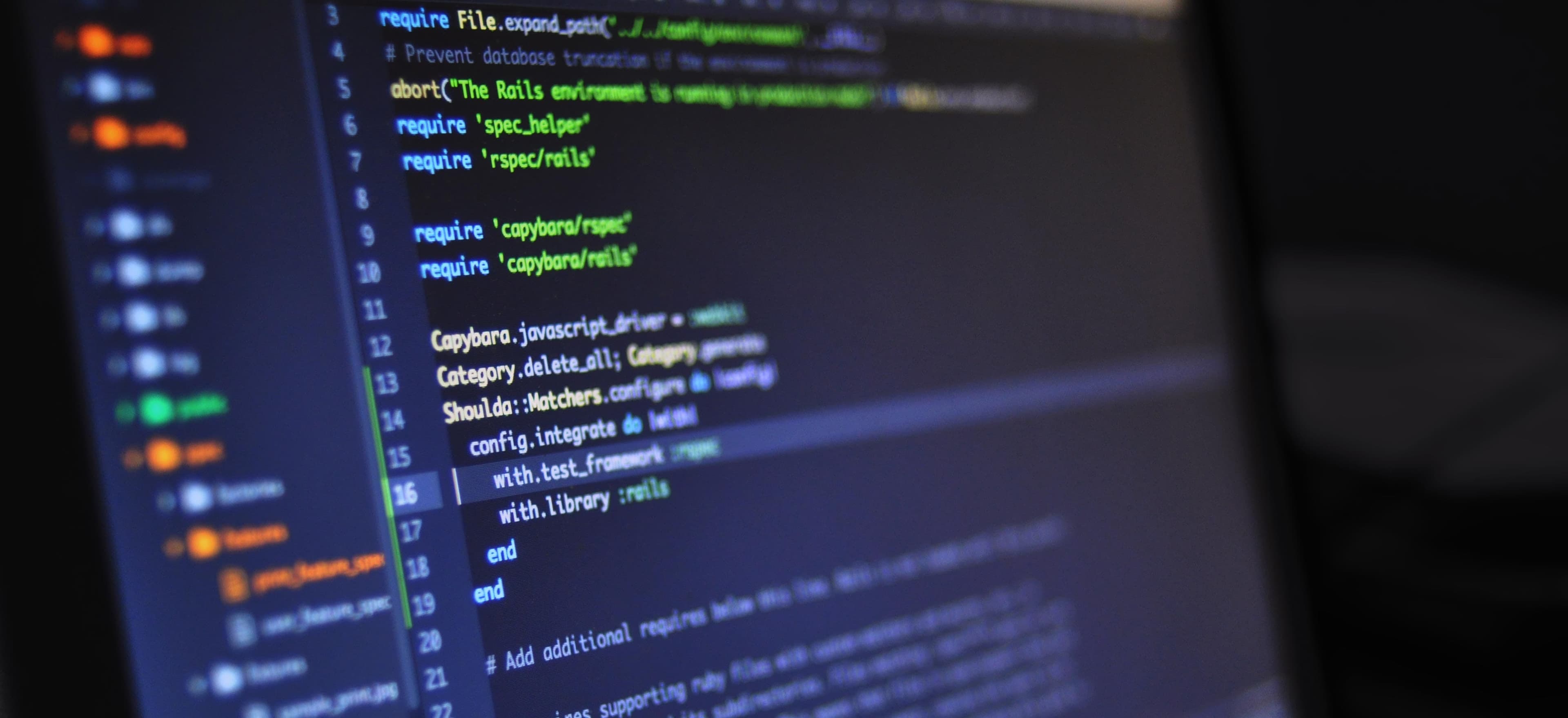
- Published on
Streamlining Code with Polymorphism and Composition in Java
In the world of Java programming, writing clean, efficient, and maintainable code is crucial. One way to achieve these goals is through the use of polymorphism and composition. These object-oriented programming concepts allow for flexible and modular code, making it easier to extend and maintain applications. In this article, we will explore how polymorphism and composition can help streamline your Java code, making it more robust and scalable.
Understanding Polymorphism
Polymorphism, a fundamental principle of object-oriented programming, allows objects of different classes to be treated as objects of a common superclass. This means that a variable of a supertype can refer to a subtype object.
Consider the following example:
public interface Animal {
void makeSound();
}
public class Dog implements Animal {
@Override
public void makeSound() {
System.out.println("Woof!");
}
}
public class Cat implements Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog();
Animal cat = new Cat();
dog.makeSound(); // Output: Woof!
cat.makeSound(); // Output: Meow!
}
}
In the example above, the Animal
interface defines a common method makeSound
. The Dog
and Cat
classes implement this interface and provide their specific implementations of the makeSound
method. We can then refer to both Dog
and Cat
objects using the Animal
type, enabling us to write generic code that can work with any Animal
subtype without knowing the specific subtype at compile time.
This powerful capability of polymorphism allows for code that is easier to maintain and extend. You can add new subclasses without modifying existing code that uses the supertype. Polymorphism encourages loose coupling, as the client code only needs to know about the supertype and is not affected by changes in the subtype.
Leveraging Composition
Composition is another essential concept in object-oriented programming, where objects are composed of other objects. It promotes code reusability and flexibility by allowing classes to be constructed from a combination of other classes.
Let's take a look at an example to understand composition better:
public class Engine {
public void start() {
System.out.println("Engine started");
}
}
public class Car {
private Engine engine;
public Car() {
this.engine = new Engine();
}
public void startCar() {
engine.start();
System.out.println("Car started");
}
}
In the above example, the Car
class has a composition relationship with the Engine
class. Instead of inheriting the behavior of the Engine
, the Car
class encapsulates an instance of the Engine
and delegates the start
functionality to it. This way, the Car
class can utilize the functionalities offered by the Engine
class without being tightly coupled to it.
By using composition, you can create modular and maintainable code. It allows for more flexibility in defining the behavior of a class and promotes code reusability by enabling the reuse of existing classes within new classes.
Streamlining Code with Polymorphism and Composition
Now that we understand the concepts of polymorphism and composition, let's see how they can be combined to streamline our Java code. Consider the scenario where we have different types of vehicles, each with an engine, and we want to define the start
behavior for each vehicle type.
We can start by creating an interface Vehicle
:
public interface Vehicle {
void start();
}
Next, we can create specific vehicle classes that implement the Vehicle
interface and utilize composition to encapsulate the engine functionality:
public class Car implements Vehicle {
private Engine engine;
public Car() {
this.engine = new Engine();
}
@Override
public void start() {
engine.start();
System.out.println("Car started");
}
}
public class Motorcycle implements Vehicle {
private Engine engine;
public Motorcycle() {
this.engine = new Engine();
}
@Override
public void start() {
engine.start();
System.out.println("Motorcycle started");
}
}
In the Car
and Motorcycle
classes, we use composition to encapsulate the Engine
functionality and implement the start
method specific to each vehicle type. This approach allows us to reuse the Engine
functionality without duplicating code and enables us to define and extend behaviors for different vehicle types independently.
Now, let's create a simple application to test our implementations:
public class Main {
public static void main(String[] args) {
Vehicle car = new Car();
Vehicle motorcycle = new Motorcycle();
car.start(); // Output: Engine started
// Car started
motorcycle.start(); // Output: Engine started
// Motorcycle started
}
}
As seen in the example above, the Vehicle
interface enables us to write generic code to start any type of vehicle without knowing the specific vehicle type at compile time. We can now easily add new vehicle types by creating classes that implement the Vehicle
interface, without modifying the existing code.
By combining polymorphism and composition, we've created a flexible and maintainable design that allows us to extend our codebase with new vehicle types and behaviors. This approach promotes code reuse, reduces duplication, and encourages the creation of cohesive, loosely coupled components.
Closing Remarks
In this article, we've explored how polymorphism and composition can be leveraged to streamline Java code. By using polymorphism, we can write generic code that can work with objects of different subtypes, promoting code flexibility and maintainability. Composition allows us to construct classes from a combination of other classes, leading to modular and reusable code.
As a Java developer, understanding and applying these principles can significantly enhance the quality of your codebase. By designing with polymorphism and composition in mind, you can create scalable, extensible, and maintainable Java applications that are easier to develop and manage.
Start practicing polymorphism and composition in your Java projects, and witness the transformation of your code into a more efficient and robust software solution.
Happy coding!