Optimizing Tower Placement for Effective Defense
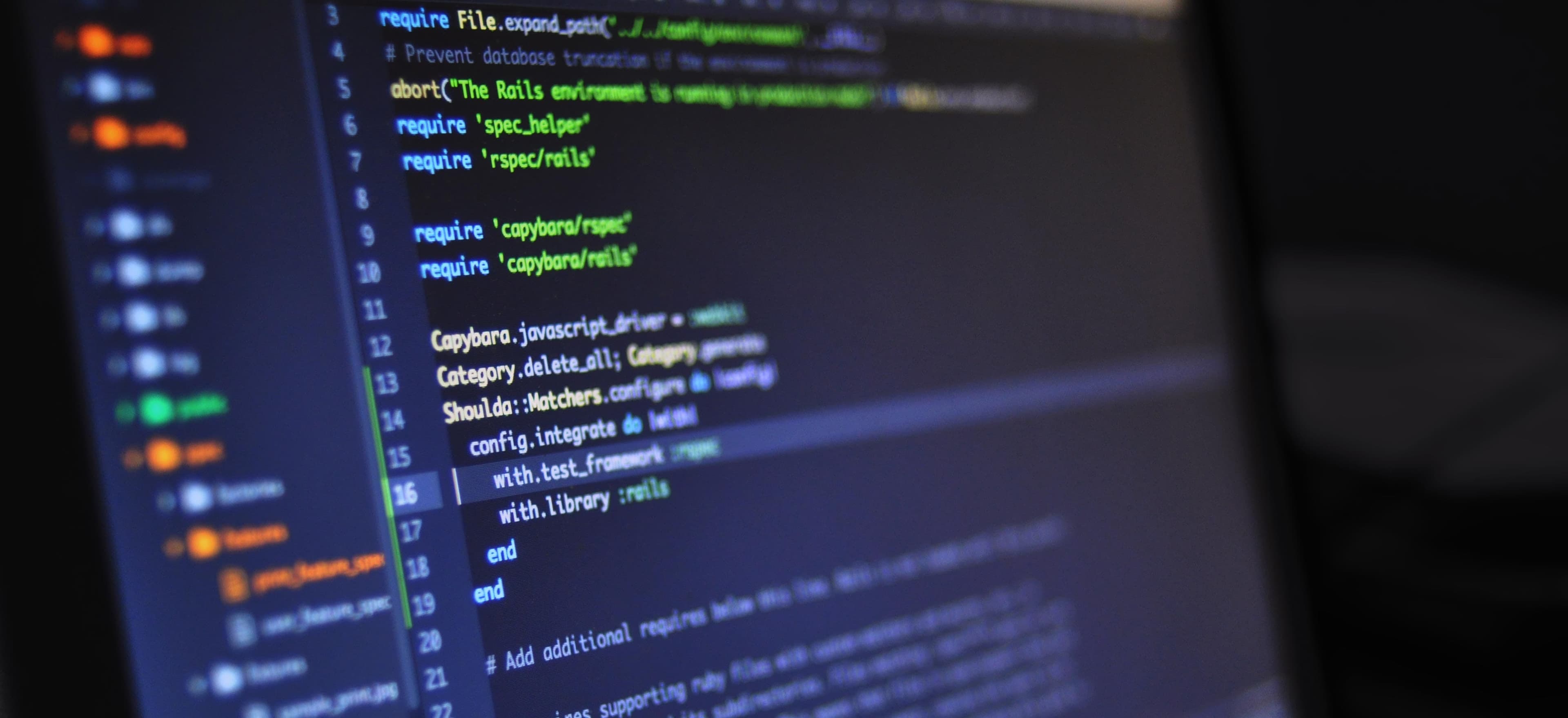
- Published on
When it comes to game development and design, Java is a powerful tool that can be used to implement various features and mechanics. One popular game genre where Java is frequently utilized is tower defense games. These games challenge players to strategically place defensive structures, such as towers, in order to stop waves of enemies from reaching their goal. In this blog post, we will delve into the process of optimizing tower placement for effective defense in Java-based tower defense games.
Understanding the Importance of Tower Placement
In a tower defense game, the placement of defensive towers is crucial to the player's success. Well-placed towers can efficiently target and eliminate incoming enemies, while poorly placed towers may allow enemies to slip through the defenses. Therefore, optimizing tower placement is a key aspect of creating an engaging and challenging gameplay experience.
The Role of Java in Tower Defense Game Development
Java offers a robust set of features for game development, including efficient data structures, object-oriented programming capabilities, and a vast collection of libraries. These features make Java an excellent choice for implementing the mechanics of a tower defense game.
Designing a Tower Placement Algorithm
To optimize tower placement, we need to design an algorithm that can intelligently identify the most strategic locations to position defensive towers. When implementing this algorithm in Java, we can leverage various data structures, algorithms, and design patterns to achieve a balance between computational efficiency and strategic decision-making.
Utilizing Pathfinding Algorithms
One approach to optimizing tower placement is to employ pathfinding algorithms to predict the routes that enemies will take as they progress through the game level. By analyzing potential pathing options, we can identify key choke points or intersections where the placement of towers would be most effective.
// Example of utilizing A* pathfinding algorithm to identify optimal tower placement locations
public List<Point> findOptimalTowerLocations(GameMap map, List<Enemy> enemies) {
AStarPathfinder pathfinder = new AStarPathfinder(map);
List<Point> optimalLocations = new ArrayList<>();
for (Enemy enemy : enemies) {
// Calculate the path the enemy will take
List<Point> enemyPath = pathfinder.calculatePath(enemy.getCurrentPosition(), enemy.getTargetPosition());
// Analyze the enemy path and identify potential tower placement locations
// Add the optimal locations to the list
}
return optimalLocations;
}
In the provided code snippet, we demonstrate how the A* pathfinding algorithm can be utilized to calculate the optimal locations for tower placement based on the predicted paths of enemies.
Considering Enemy Behavior and Targeting
Another factor to consider when optimizing tower placement is the behavior and targeting priorities of the enemies. By analyzing the attributes of different enemy types, such as speed, armor, and resistances, we can tailor our tower placement strategy to effectively counter these variations.
// Example of considering enemy attributes to optimize tower placement
public void optimizeTowerPlacement(List<Enemy> enemies, List<Tower> towers) {
for (Enemy enemy : enemies) {
// Analyze enemy attributes and vulnerabilities
// Deploy towers with effective targeting against specific enemy attributes
}
}
In this example, we illustrate how the characteristics of the enemies can inform the placement of towers with specialized targeting capabilities, such as slowing down fast enemies or dealing increased damage to heavily armored foes.
Leveraging Data Structures for Spatial Analysis
When optimizing tower placement, spatial analysis of the game level is essential for identifying strategic positions. Java provides a wide range of data structures, such as grids, trees, and graphs, which can be utilized to efficiently represent and analyze the spatial layout of the game environment.
// Example of using a grid data structure for spatial analysis in tower placement optimization
public void analyzeSpatialLayout(GameMap map) {
Grid<Cell> spatialGrid = new Grid<>(map.getWidth(), map.getHeight());
// Populate the grid with information about the terrain, paths, and obstacles
// Analyze the spatial data to identify key locations for tower placement
}
In this code snippet, we showcase how a grid data structure can be used to perform spatial analysis of the game map, enabling the identification of optimal tower placement locations based on the terrain and enemy pathing.
Closing Remarks
Optimizing tower placement for effective defense in Java-based tower defense games involves a multifaceted approach that combines pathfinding algorithms, enemy behavior analysis, and spatial data structures. By leveraging the features of Java for game development, we can create sophisticated algorithms and systems to enhance the strategic depth and challenge of tower defense gameplay.
In the dynamic world of game development, Java continues to be a versatile and powerful language for implementing engaging and immersive gaming experiences. As developers, we have the opportunity to push the boundaries of tower defense game design by employing advanced optimization techniques and leveraging the capabilities of Java to create compelling and strategic gameplay.
For further exploration of Java game development and optimization, consider diving into Java Gaming Resources and Algorithms in Java for a comprehensive understanding of the subject matter.