Troubleshooting Connection Leaks in Tomcat-JDBC Pool
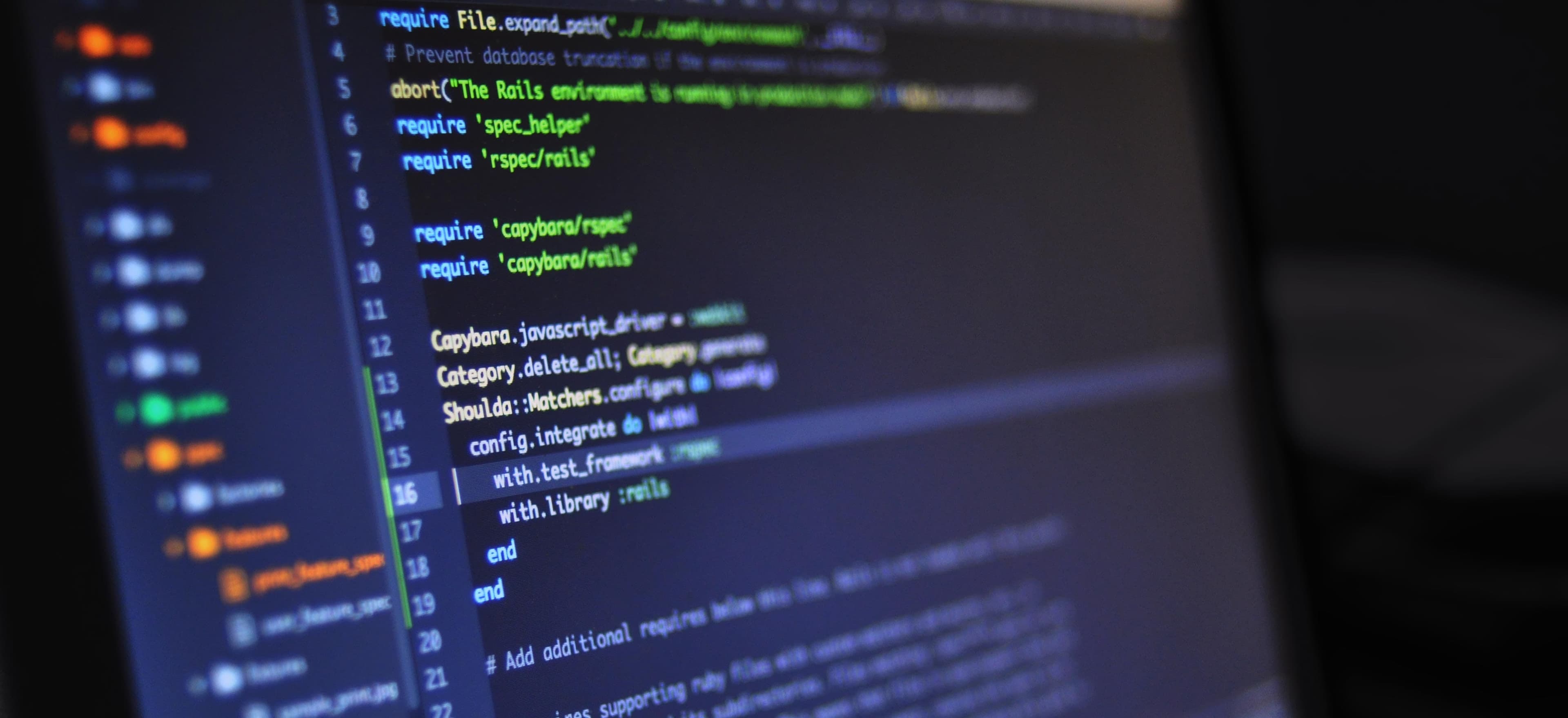
- Published on
Troubleshooting Connection Leaks in Tomcat-JDBC Pool
When using a connection pool in a Java application, connection leaks can be a common and frustrating issue to encounter. Connection leaks occur when a database connection is not properly closed after its intended use, which can lead to a depletion of available connections in the pool and ultimately result in application downtime. In this article, we will explore how to identify and troubleshoot connection leaks in the Tomcat-JDBC connection pool, offering insights into identifying the root cause and addressing the issue effectively.
Understanding Connection Leaks
Before diving into troubleshooting, it's crucial to understand how connection leaks can occur within a Tomcat-JDBC connection pool. When a connection is obtained from the pool for database operations, it's imperative to close the connection explicitly once it's no longer needed. Failure to do so can lead to leaks, where connections are not returned to the pool for reuse, causing it to eventually run out of available connections.
Identifying Connection Leaks
Monitoring Connection Usage
One of the first steps in identifying connection leaks is to monitor the usage of connections within the application. This can be achieved by enabling logging for connection open and close events in the Tomcat-JDBC pool.
import org.apache.juli.logging.Log;
import org.apache.juli.logging.LogFactory;
public class CustomDataSource extends org.apache.tomcat.jdbc.pool.DataSource {
private static Log log = LogFactory.getLog(CustomDataSource.class);
@Override
public void createConnectionPool() throws SQLException {
super.createConnectionPool();
this.getPoolProperties().setJdbcInterceptors(
"org.apache.tomcat.jdbc.pool.interceptor.ConnectionState;"+
"org.apache.tomcat.jdbc.pool.interceptor.StatementFinalizer");
}
@Override
public void close() {
log.info("Closing custom data source");
super.close();
}
}
In the above code snippet, we subclass the DataSource
from Tomcat-JDBC and override the createConnectionPool
method to add JdbcInterceptors
for monitoring connection states. By logging these events, we can track the open and close actions on connections.
Analyzing Thread Dumps
Thread dumps can provide valuable insights into the state of threads and their associated database connections. By analyzing thread dumps when the application is experiencing connection leaks, we can identify which threads are holding onto connections without releasing them.
Resolving Connection Leaks
Reviewing Code for Proper Connection Handling
It's essential to review the application code where database connections are used. Ensure that each connection retrieval is followed by a proper try-finally block to guarantee closure, or better yet, utilize Java's try-with-resources statement for automatic resource management.
try (Connection conn = dataSource.getConnection()) {
// Perform database operations
} catch (SQLException ex) {
// Handle exceptions
}
By adopting the try-with-resources statement, connections will be automatically closed, mitigating the risk of potential leaks.
Implementing Connection Leak Detection
Tomcat-JDBC provides a built-in feature to detect connection leaks by setting the logAbandoned
and removeAbandoned
properties in the connection pool configuration.
<Resource name="jdbc/yourDB" auth="Container" type="javax.sql.DataSource"
maxActive="100" maxIdle="30" maxWait="10000"
username="user" password="password" driverClassName="com.mysql.jdbc.Driver"
url="jdbc:mysql://localhost:3306/yourDB"
logAbandoned="true" removeAbandoned="true"
removeAbandonedTimeout="60" />
With logAbandoned
set to true
, any connections that are not properly closed will be logged, allowing for easier identification of potential leaks. Additionally, setting removeAbandoned
to true
with a defined removeAbandonedTimeout
will automatically remove leaked connections after the specified time, preventing them from causing further issues.
Utilizing Connection Health Validation
Another approach to prevent connection leaks is to utilize connection health validation. Tomcat-JDBC supports the validationQuery
property, which can be used to execute a validation query on connections to ensure their health before returning them to the pool.
<Resource name="jdbc/yourDB" auth="Container" type="javax.sql.DataSource"
maxActive="100" maxIdle="30" maxWait="10000"
username="user" password="password" driverClassName="com.mysql.jdbc.Driver"
url="jdbc:mysql://localhost:3306/yourDB"
validationQuery="SELECT 1" testOnBorrow="true" />
By setting testOnBorrow
to true
, the validation query will be executed when a connection is borrowed from the pool, allowing for the detection of unhealthy connections before they are used, thereby reducing the likelihood of leaks.
Lessons Learned
Connection leaks in a Tomcat-JDBC connection pool can have detrimental effects on the stability and performance of a Java application. By understanding how to identify and troubleshoot connection leaks, as well as implementing best practices for connection handling and pool configuration, developers can effectively mitigate and prevent such issues, leading to a more robust and reliable application.
In closing, proactive monitoring, thorough code review, and leveraging the features provided by Tomcat-JDBC are essential steps in addressing connection leaks and maintaining a healthy connection pool environment for Java applications.
To delve deeper into connection pool management with Tomcat-JDBC, consider reviewing the official Tomcat-JDBC documentation and this insightful article on Java connection pooling best practices.