Overloading Restrictions in Java 8 Method References
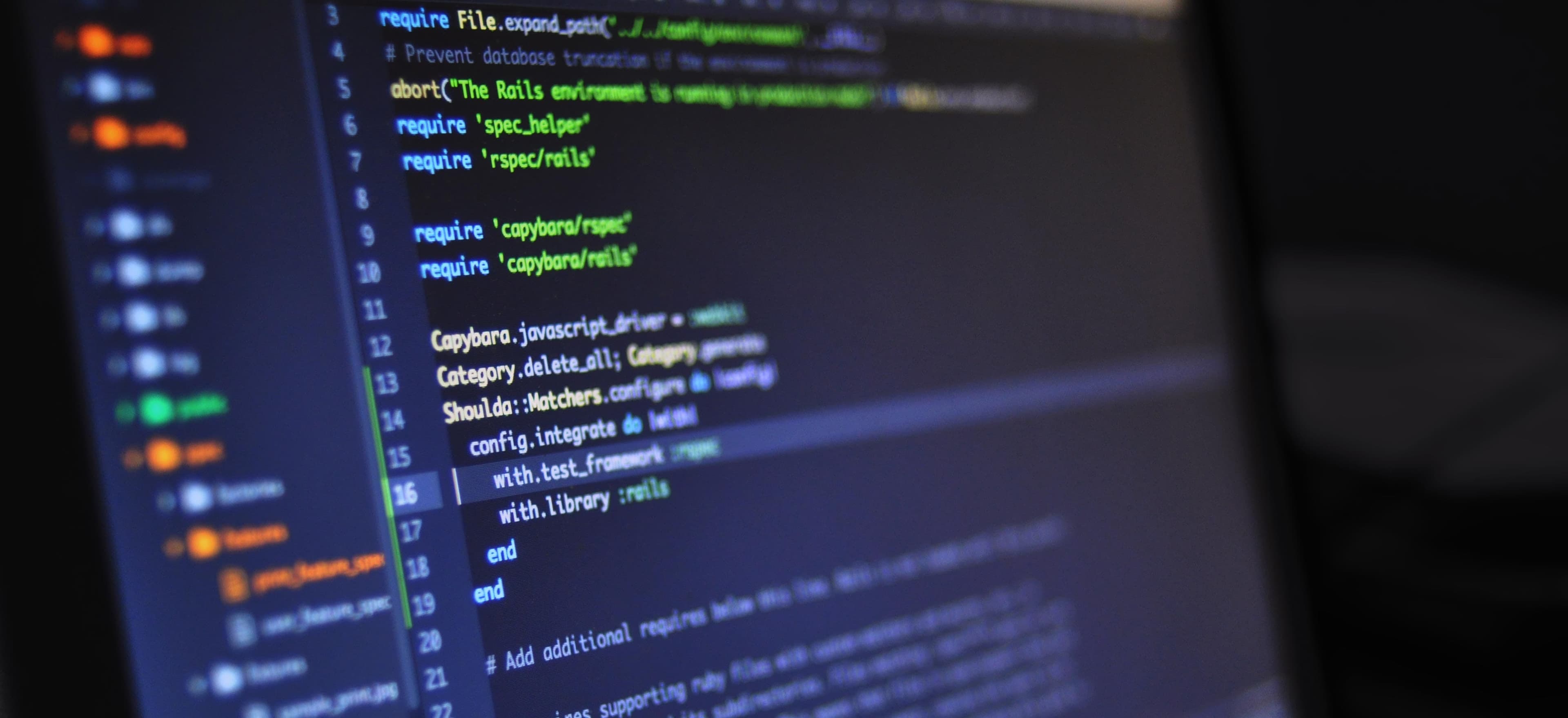
- Published on
Understanding Overloading Restrictions in Java 8 Method References
When working with Java 8 and above, method references provide a concise way to refer to methods or constructors for functional interfaces. However, there are certain restrictions and considerations when it comes to overloading methods and using method references. In this post, we'll delve into the limitations and best practices for using method references in Java 8, focusing on overloading restrictions.
What are Method References in Java 8?
Method references allow you to refer directly to a method or constructor by its name. They make the code more concise and readable by providing a way to replace lambda expressions that call a single method. There are four main types of method references in Java 8:
- Reference to a static method
- Reference to an instance method of a particular object
- Reference to an instance method of an arbitrary object of a particular type
- Reference to a constructor
For example, here's a simple static method reference:
// Static method
Function<String, Integer> converter = Integer::parseInt;
In this example, Integer::parseInt
refers to the static method parseInt
of the Integer
class.
Overloading Restrictions in Method References
One of the key considerations when using method references in Java 8 is understanding the restrictions related to overloading. In Java, overloading refers to the ability to define multiple methods in the same class with the same name but different parameter lists.
When using method references, these overloading restrictions come into play, particularly when referencing overloaded methods. Let's explore these restrictions in detail.
Ambiguity Due to Overloading
Consider a class Calculator
with overloaded add
methods:
class Calculator {
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
}
Now, let's assume we want to use a method reference to refer to one of the overloaded add
methods. Due to the overloading of add
, when trying to reference it, the compiler won't be able to determine which version of the method to reference based solely on the functional interface's signature.
Attempting to create a functional interface and refer to the overloaded add
method with method reference would result in a compilation error due to ambiguity.
Resolving Ambiguity
To resolve the ambiguity caused by overloading when using method references, you can explicitly provide a lambda expression instead of a method reference. By using a lambda expression, you can specify the exact method with its parameter list, removing the ambiguity that arises from overloading.
Calculator calculator = new Calculator();
// Reference to the int add method
BiFunction<Integer, Integer, Integer> addInt = calculator::add;
// Reference to the double add method
DoubleBinaryOperator addDouble = (a, b) -> calculator.add(a, b);
Explicitly specifying the desired method with its parameter types in the lambda expression avoids the ambiguity caused by method overloading.
Best Practices for Overloaded Methods and Method References
When dealing with overloaded methods and method references, it's important to consider the following best practices:
-
Use explicit lambda expressions: When dealing with overloaded methods, explicitly specify the method and its parameter list using a lambda expression to avoid ambiguity.
-
Rename methods: If possible, consider renaming overloaded methods to have distinct names. This can help alleviate the ambiguity when using method references.
-
Document the intent: If renaming the methods is not feasible, document the intended usage of method references in the code to make it clear which overloaded method is being referred to.
Lessons Learned
Method references in Java 8 provide a convenient way to refer to methods or constructors for functional interfaces. However, when dealing with overloaded methods, it's crucial to understand the restrictions and best practices to avoid ambiguity and compilation errors.
By using explicit lambda expressions, resolving ambiguity through method renaming, or documenting the intended usage, you can effectively work with method references and overloaded methods in Java 8.
Understanding the overloading restrictions in Java 8 method references empowers developers to write clean, unambiguous code that leverages the power of functional programming in Java.
For further in-depth understanding, you can refer to the official Java documentation on method references.
In conclusion, method references in Java 8 are a powerful feature for functional programming, but being aware of their limitations, especially when dealing with overloaded methods, is essential for writing robust and maintainable code.
Stay tuned for more insights into the nuances of Java programming!