Adapting Code Reviews for Evolving Software Development
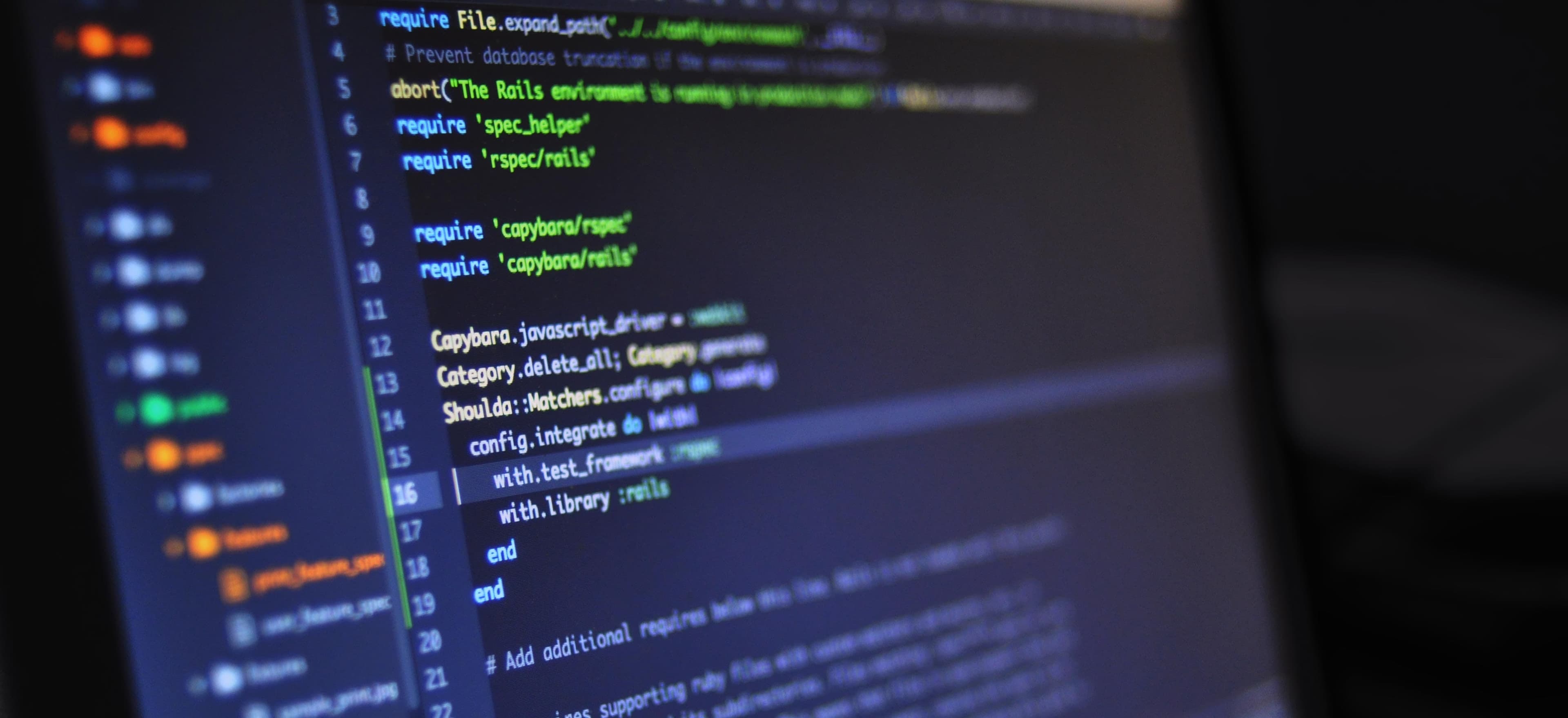
- Published on
Adapting Code Reviews for Evolving Software Development
In the fast-paced world of software development, adaptability is key. This applies not just to the code we write, but also to the processes and practices we employ. One such practice is code reviews, which play a crucial role in ensuring the quality, maintainability, and scalability of the codebase. However, as software development methodologies and tools evolve, so too must the way we approach and conduct code reviews. In this article, we'll explore how code reviews can be adapted to meet the needs of modern software development.
The Role of Code Reviews
Before delving into the adaptations, let's first discuss the fundamental role of code reviews in the software development lifecycle. Code reviews are a systematic examination of source code, often performed by one's peers, with the goal of identifying and addressing issues before they become entrenched in the codebase. They help in maintaining code quality, spreading knowledge across the team, and preventing the introduction of bugs and technical debt.
Embracing Continuous Integration and Code Review
In the era of continuous integration and continuous delivery (CI/CD), the role of code reviews has evolved. Traditionally, code reviews were seen as a gatekeeper to catch issues before merging code into the main branch. While this is still an important aspect, code reviews in the context of CI/CD should also focus on ensuring that the changes integrate well with the existing codebase and do not disrupt the continuous delivery pipeline.
Exemplary Code Snippet:
public class OrderService {
public void processOrder(Order order) {
// Processing logic here
}
}
In the above example, a simple OrderService
class is shown. Before the code review, one might consider checks for potential issues such as error handling, input validation, and adherence to coding standards. However, in the context of CI/CD, the review should also ensure that this change does not introduce performance bottlenecks or compatibility issues with other parts of the system.
Leveraging Automation and Tools
To adapt code reviews to modern software development, leveraging automation and tools is crucial. Static code analysis tools, such as SonarQube or Checkstyle, can automatically check for adherence to coding standards, potential bugs, and code smells. Integrating these tools into the CI/CD pipeline not only automates part of the review process but also promotes consistency in code quality across the codebase.
Exemplary Code Snippet:
public class Customer {
private String firstName;
private String lastName;
// Getters and setters
}
In the code snippet above, it's important to ensure that naming conventions are followed, and that there are no potential null pointer exceptions. By leveraging automated tools, these checks can be performed consistently and efficiently, freeing up human reviewers to focus on more complex issues such as architectural alignment and design decisions.
Cultivating a Positive Code Review Culture
Adapting code reviews for evolving software development also involves cultivating a positive code review culture within the team. This entails promoting constructive feedback, knowledge sharing, and a growth mindset. A positive code review culture not only improves the quality of the codebase but also fosters a sense of collaboration and continuous improvement within the team.
Exemplary Code Snippet:
public class ProductService {
public List<Product> getProductsByCategory(String category) {
// Implementation here
}
}
In the context of cultivating a positive code review culture, the focus should not only be on identifying issues, but also on acknowledging and highlighting good practices and design decisions. Feedback should be framed in a constructive manner, with an emphasis on collective learning and improvement.
Final Considerations
As software development practices continue to evolve, so must the way we approach code reviews. By embracing continuous integration, leveraging automation and tools, and cultivating a positive code review culture, teams can adapt code reviews to meet the needs of modern software development. The goal remains the same – ensuring the quality, maintainability, and scalability of the codebase – but the approach evolves to align with the pace and demands of the ever-changing software development landscape.
So, next time you embark on a code review, remember to consider the broader context of evolving software development and adapt your approach accordingly. After all, just as code evolves, so should the practices that uphold its quality and integrity.
To delve deeper into the world of modern code reviews, check out A Guide to Effective Code Reviews and The Role of Code Quality in Software Development.