Enhancing User Engagement with Vuforia SDK
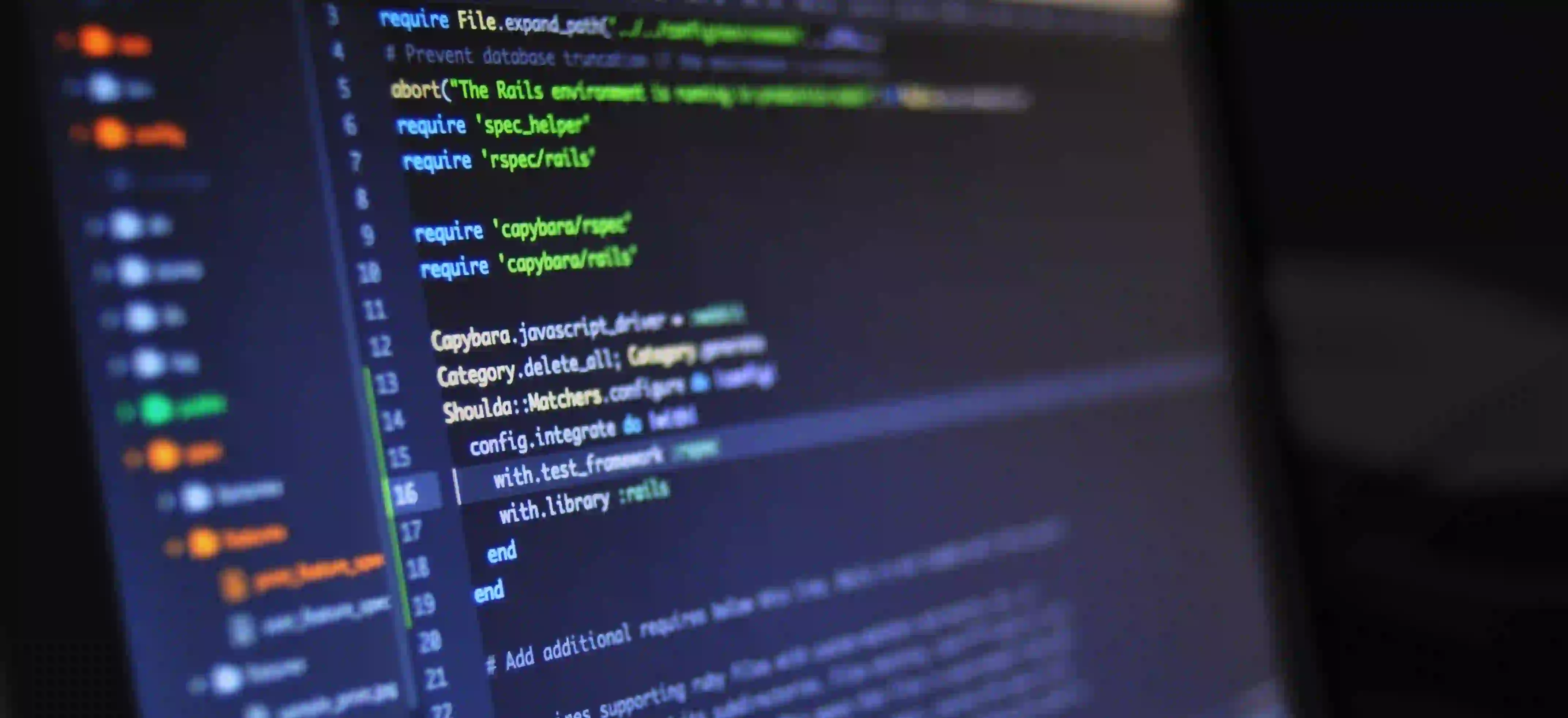
Enhancing User Engagement with Vuforia SDK
In today's tech-driven world, user engagement is a key factor in the success of any application. Augmented Reality (AR) has gained significant traction in recent years and has proven to be an effective tool for enhancing user engagement. With the Vuforia Software Development Kit (SDK), developers can easily integrate AR capabilities into their Java applications.
What is Vuforia SDK?
Vuforia is a popular AR development platform that provides developers with the tools and resources to create engaging AR experiences. It supports various platforms, including Android and iOS, and offers robust features such as image recognition, 3D object recognition, and environment recognition.
Getting Started with Vuforia SDK in Java
To begin integrating Vuforia SDK into a Java application, you'll need to follow a series of steps that include setting up the development environment, integrating the Vuforia SDK, and implementing AR features.
Setting Up the Development Environment
Before diving into the code, ensure that you have Java Development Kit (JDK) installed on your system. You can download and install the latest version of JDK from the official Oracle website.
Next, you'll need to set up your preferred Integrated Development Environment (IDE) for Java development. Popular choices include IntelliJ IDEA, Eclipse, and NetBeans. Choose the IDE that best suits your workflow and install it on your system.
Integrating Vuforia SDK into a Java Project
Once your development environment is set up, you can proceed to integrate the Vuforia SDK into your Java project. You can add the Vuforia SDK as a dependency in your project using a build automation tool such as Maven or Gradle. Here's a Maven example of adding Vuforia SDK as a dependency in your pom.xml
file:
<dependencies>
<dependency>
<groupId>com.vuforia</groupId>
<artifactId>vuforia-sdk</artifactId>
<version>9.8.7</version>
</dependency>
</dependencies>
In this example, we're adding the Vuforia SDK dependency with version 9.8.7 to our Maven project. This allows us to access the Vuforia SDK features and functionality within our Java application.
Implementing AR Features with Vuforia SDK
Now that the Vuforia SDK is integrated into your Java project, you can start implementing AR features to enhance user engagement. One of the most common use cases is marker-based AR, where the application recognizes and augments specific images or objects.
Let's take a look at a simple Java code snippet that demonstrates how to initialize the Vuforia SDK and load a target image for marker-based AR:
import com.vuforia.Vuforia;
public class ARManager {
private static final String VUFORIA_ACCESS_KEY = "your-vuforia-access-key";
public void initVuforia() {
Vuforia.setInitParameters(this, VUFORIA_ACCESS_KEY);
Vuforia.init();
}
public void loadTargetImage(String targetImageFile) {
// Load the target image for marker-based AR
// Code to load the target image
}
}
In this example, we initialize the Vuforia SDK with the provided access key and load a target image for marker-based AR. This sets the foundation for creating an immersive AR experience within your Java application.
Best Practices for User Engagement with Vuforia SDK
To maximize user engagement using Vuforia SDK in your Java application, consider the following best practices:
-
Intuitive User Interface: Design an intuitive and user-friendly interface that seamlessly integrates AR elements for a cohesive user experience.
-
Interactive AR Content: Implement interactive AR content, such as virtual buttons or 3D animations, to encourage user interaction and exploration.
-
Performance Optimization: Ensure smooth performance by optimizing AR content rendering and minimizing latency for a seamless user experience.
-
Guided Onboarding: Provide user guidance and onboarding tutorials to familiarize users with the AR features and functionalities of your application.
-
Social Sharing: Enable users to share their AR experiences on social media platforms, fostering engagement and user-generated content.
Wrapping Up
Incorporating Vuforia SDK into your Java application opens up a world of possibilities for enhancing user engagement through immersive AR experiences. By following best practices and leveraging the powerful features of Vuforia SDK, you can create compelling AR applications that captivate and delight users.
Start exploring the potential of Vuforia SDK in Java development, and elevate your application's user engagement to new heights. Happy coding!