Optimizing Database Performance with Hibernate Sorting
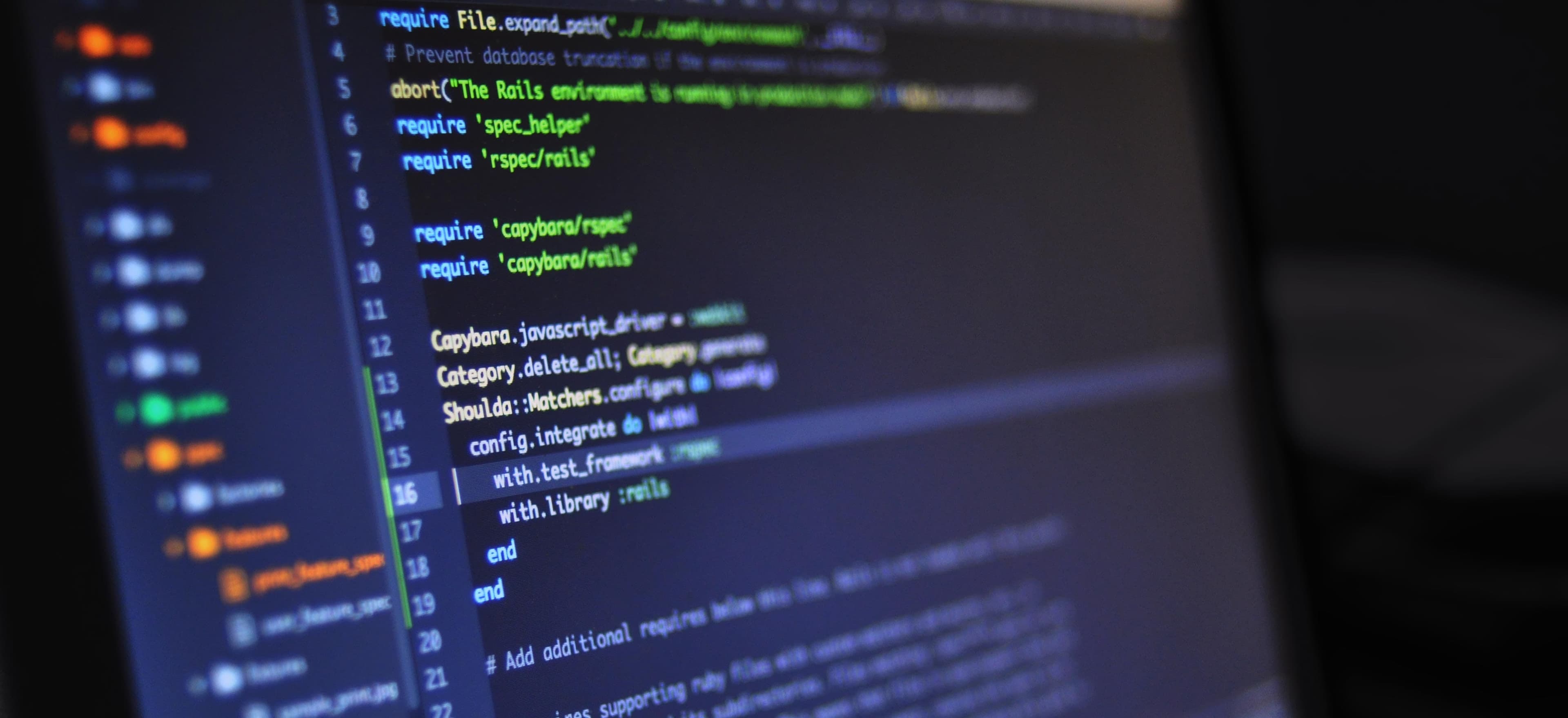
- Published on
Optimizing Database Performance with Hibernate Sorting
In this blog post, we will delve into the world of Hibernate sorting and how it can be utilized to optimize database performance. Efficient sorting is crucial in ensuring that database operations are executed in a manner that minimizes overhead and maximizes speed. We will explore the significance of sorting in the context of Hibernate, and how we can employ best practices to enhance the performance of our applications.
Understanding the Importance of Sorting
Sorting plays a pivotal role in database operations, as it allows us to arrange the data in a specific order based on one or more criteria. This is particularly significant when dealing with large datasets, as unsorted data can lead to sluggish performance and inefficient queries. By organizing the data, we can streamline our retrieval and manipulation processes, ultimately leading to a more responsive and efficient application.
Sorting with Hibernate
Hibernate, as an Object-Relational Mapping (ORM) framework, provides an array of features for sorting data fetched from the database. When working with Hibernate, we have the flexibility to perform sorting operations at the database level, thereby optimizing the retrieval process and minimizing the amount of data transferred to the application.
Sorting in HQL (Hibernate Query Language)
Hibernate Query Language (HQL) allows us to construct database-independent queries for sorting data. Let's take a look at an example of sorting entities using HQL:
String hql = "FROM User ORDER BY registrationDate DESC";
Query query = session.createQuery(hql);
List<User> users = query.list();
In this example, we are sorting the User
entities based on their registrationDate
in descending order. By specifying the sorting criteria within the HQL query, we can offload the sorting operation to the database, optimizing the performance of our application.
Criteria API for Sorting
Hibernate also offers the Criteria API, which provides a programmatic approach for building queries, including sorting operations. Let's explore how we can perform sorting using the Criteria API:
CriteriaBuilder criteriaBuilder = session.getCriteriaBuilder();
CriteriaQuery<User> criteriaQuery = criteriaBuilder.createQuery(User.class);
Root<User> root = criteriaQuery.from(User.class);
criteriaQuery.orderBy(criteriaBuilder.desc(root.get("registrationDate")));
List<User> users = session.createQuery(criteriaQuery).getResultList();
In this snippet, we are utilizing the Criteria API to create a query that sorts the User
entities based on their registrationDate
in descending order. By leveraging the Criteria API, we can construct type-safe queries and incorporate sorting seamlessly into our data retrieval process.
Optimizing Sorting in Hibernate
While Hibernate equips us with powerful tools for sorting data, it is essential to optimize our sorting operations to enhance database performance. Here are some best practices to consider:
Indexing
Indexing plays a crucial role in optimizing sorting operations. By creating indexes on the columns frequently used for sorting, we can significantly reduce the time taken to retrieve and arrange the data. It is imperative to identify the appropriate columns for indexing based on the application's querying patterns.
Pagination
When dealing with large datasets, implementing pagination alongside sorting can drastically improve performance. By fetching and displaying data in smaller, manageable chunks, we can alleviate the burdens on the database and enhance the responsiveness of our application.
Efficient Data Modeling
Careful consideration of data modeling can greatly impact sorting performance. By designing the database schema to align with the application's querying and sorting requirements, we can minimize the need for complex sorting operations and streamline data retrieval.
Query Performance Tuning
Regular performance evaluation and query optimization are essential for maintaining efficient sorting operations. Analyzing the query execution plans, identifying bottlenecks, and fine-tuning the queries can lead to substantial performance enhancements.
Final Considerations
Sorting is an integral aspect of database operations, and its efficient implementation is paramount for optimizing application performance. In the realm of Hibernate, leveraging sorting capabilities wisely can result in substantial performance gains. By combining effective indexing, pagination, data modeling, and query optimization, we can harness the full potential of Hibernate sorting to create responsive and high-performing applications.
In conclusion, Hibernate provides a robust set of tools for sorting data efficiently, and by adhering to best practices, we can elevate the performance of our applications and deliver optimal user experiences.
Implementing sorting in Hibernate isn't just about arranging data - it's about fine-tuning the performance of our applications for seamless and efficient database operations. With the right approach and best practices, Hibernate sorting becomes a powerful ally in our quest for top-notch application performance.