Speed Up Your Code Production with These Diagnosis Tips
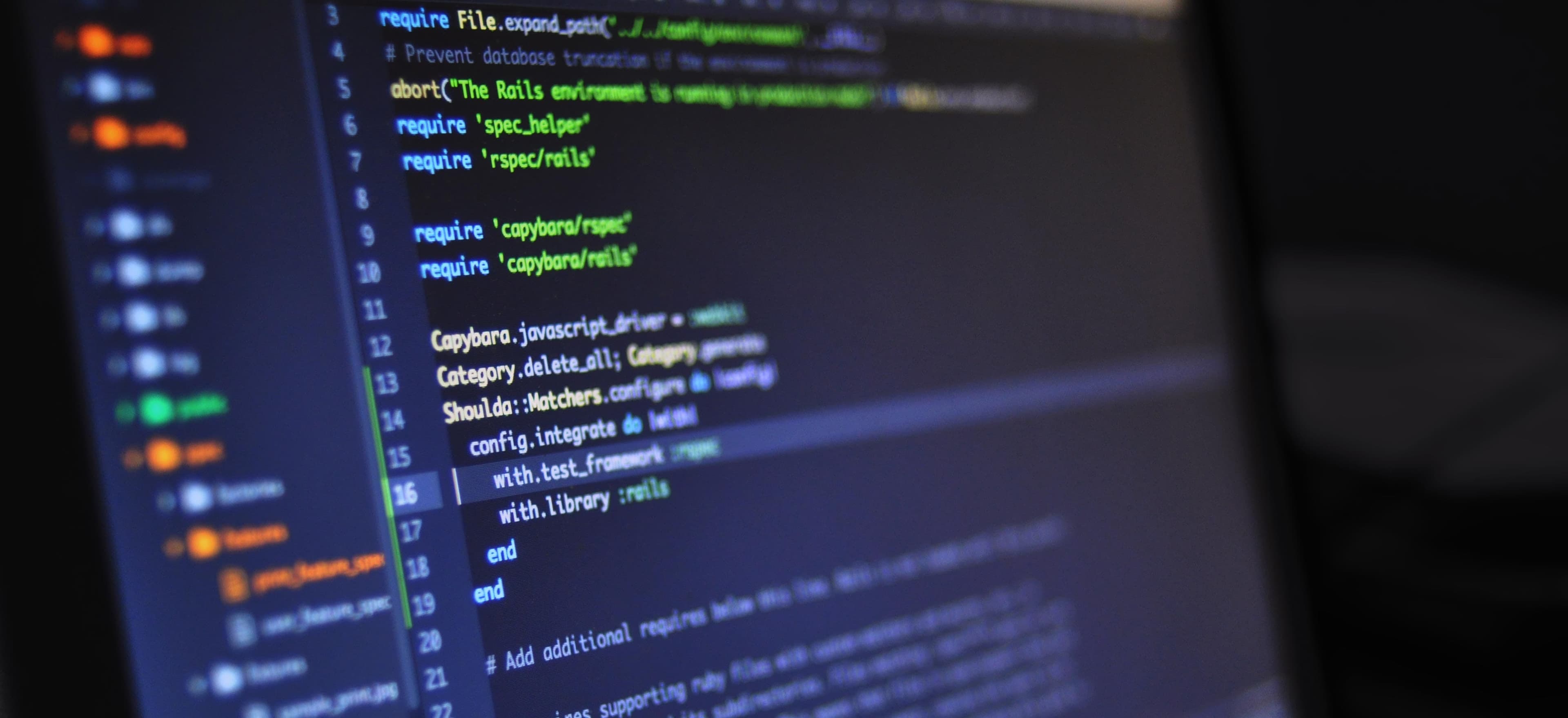
- Published on
Speed Up Your Code Production with These Diagnosis Tips
Developing in Java can be a fulfilling experience, but it can also be frustrating when your code isn't running as efficiently as you'd like. Before you start optimizing, it's crucial to diagnose where your code may be slowing down. In this post, we'll explore some valuable tips for diagnosing performance issues in your Java code.
Utilize Profiling Tools
Profiling tools are essential for pinpointing performance bottlene. They provide insightful data on memory usage, CPU consumption, and method execution times. Tools like VisualVM and YourKit offer intuitive interfaces and advanced profiling capabilities.
Example: Code profiling with VisualVM
public class ProfilingExample {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
String result = "";
for (int j = 0; j < 1000; j++) {
result += j; // This is a potential performance bottleneck
}
System.out.println(result);
}
}
}
By running the example code through a profiler like VisualVM, you can identify the performance bottleneck in the concatenation operation result += j
. This insight will guide you in optimizing your code effectively.
Leverage Java Flight Recorder
Java Flight Recorder (JFR) is a profiling and diagnostic tool integrated into the Java Virtual Machine (JVM). It provides detailed recordings of your application's performance, including method profiling, thread analysis, and garbage collection statistics.
Example: Using Java Flight Recorder
java -XX:+UnlockCommercialFeatures -XX:+FlightRecorder ...
After enabling JFR, you can analyze the generated recordings to identify areas for performance improvement.
Monitor Garbage Collection (GC) Activity
Inefficient memory management can significantly impact performance. Monitoring GC activity is crucial for identifying memory leaks and excessive object creation. Tools such as GCMV and GCViewer visualize GC logs, making it easier to spot inefficiencies.
Example: Monitoring GC with GCMV
java -Xlog:gc*:file=gc.log ...
Analyzing the GC log file with GCMV will provide insights into GC efficiency, allowing you to optimize memory usage in your Java application.
Use JMH for Micro-benchmarking
Java Microbenchmarking Harness (JMH) is a powerful tool for measuring the performance of small code snippets. It eliminates common benchmarking pitfalls and provides reliable performance comparisons.
Example: Micro-benchmarking with JMH
@State(Scope.Thread)
public class MyBenchmark {
@Benchmark
public void testMethod() {
// Code snippet to be benchmarked
}
}
By benchmarking critical code sections with JMH, you can identify areas for improvement and prevent premature optimizations.
Analyze CPU Utilization
Understanding how your code utilizes CPU resources is vital for optimizing performance. Tools like Java VisualVM and JMC offer CPU profiling capabilities to identify hot methods and CPU-consuming tasks.
Example: CPU profiling with Java VisualVM
java -server -XX:+UnlockCommercialFeatures -XX:+FlightRecorder -XX:StartFlightRecording=duration=60s,filename=myrecording.jfr ...
Analyzing the recording with Java VisualVM will highlight CPU-intensive sections, guiding your optimization efforts.
Closing the Chapter
Diagnosing performance issues in your Java code is an essential step towards achieving optimal performance. By utilizing profiling tools, monitoring GC activity, leveraging JMH for micro-benchmarking, and analyzing CPU utilization, you can identify performance bottlenecks and make informed optimization decisions.
Remember, it's crucial to diagnose before you optimize. Understanding where your code can be improved will result in targeted and effective performance enhancements. So, leverage these tips, diagnose efficiently, and propel your Java code to peak performance.