Improving Angular Performance with TrackBy Functions
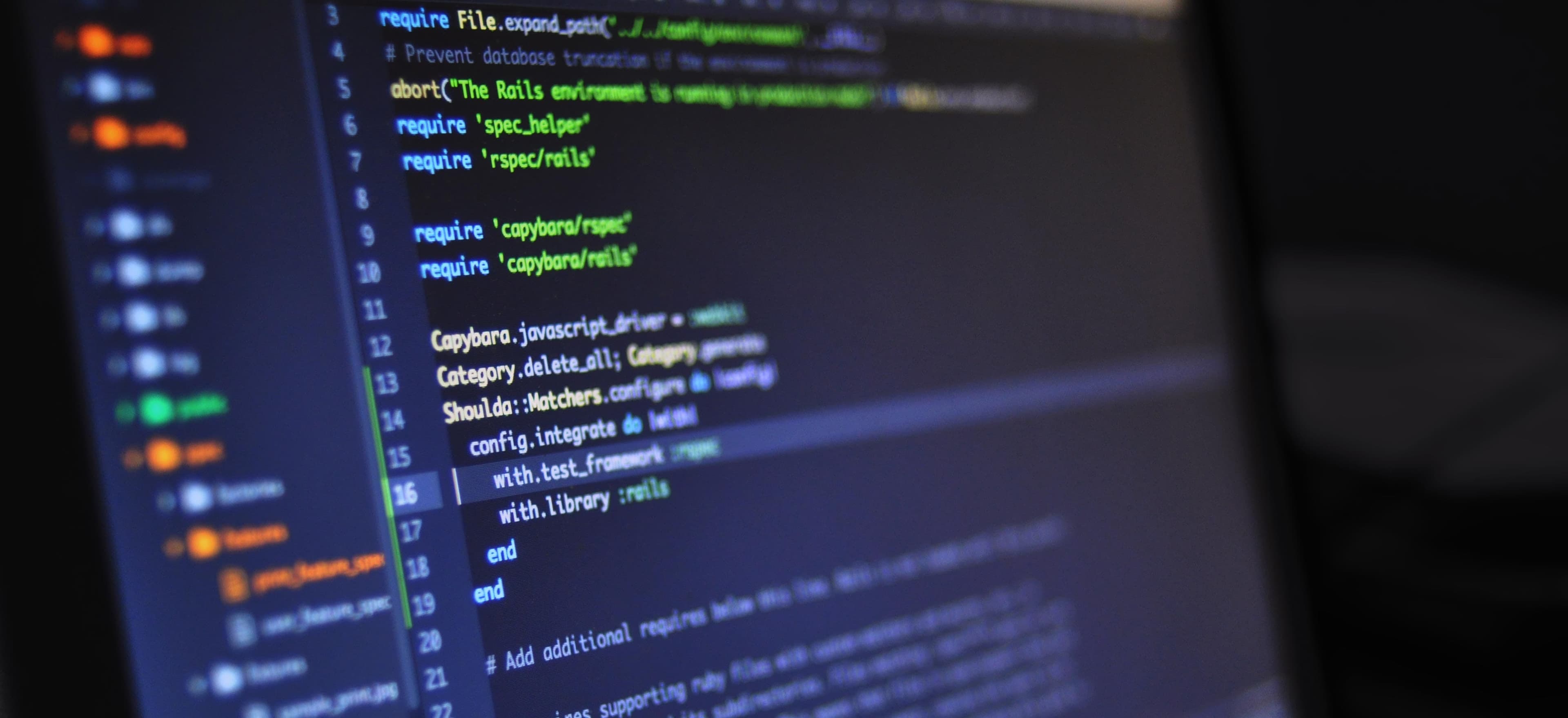
- Published on
Improving Angular Performance with TrackBy Functions
When working with Angular, performance optimization is always a crucial aspect to consider. As your application grows, so does the need for efficient data handling and rendering. One common area where performance can be optimized is in the rendering of lists using *ngFor
directive. In this article, we will explore how to enhance the performance of Angular applications by utilizing the trackBy
function in conjunction with *ngFor
to minimize unnecessary DOM manipulations.
Understanding the Issue
When rendering lists in Angular using *ngFor
, the framework needs a way to uniquely identify each item in the list. By default, Angular uses the object identity of the item to track changes, which can result in unnecessary re-rendering of the entire list when the identity of the items changes, even if the actual content remains the same.
Consider the following code snippet:
<ul>
<li *ngFor="let item of items">
{{ item.name }}
</li>
</ul>
In this scenario, if the items array is re-assigned, even if the content of the items remains the same, Angular re-renders the entire list. This can lead to performance overhead, especially when dealing with large lists or when the list is frequently updated.
Introducing the trackBy Function
To address this issue, Angular provides a way to optimize list rendering by using the trackBy
function with the *ngFor
directive. The trackBy
function allows Angular to identify the unique key of each item in the list, ensuring that only the items that have changed will be re-rendered.
Here's how the trackBy
function can be used:
<ul>
<li *ngFor="let item of items; trackBy: trackByFn">
{{ item.name }}
</li>
</ul>
In the component class, define the trackByFn
function:
trackByFn(index, item) {
return item.id; // or unique identifier of the item
}
By providing trackByFn
to the *ngFor
directive, Angular will use the return value of the function as the unique identifier for each item. This allows Angular to efficiently determine which items have changed and only re-render those specific items, leading to a significant performance improvement, especially for large lists.
Why Use the trackBy Function?
The primary benefit of using the trackBy
function is the reduction of unnecessary DOM manipulations. By enabling Angular to track the identity of each item using a specific key, we can prevent the re-rendering of items that have not changed, resulting in smoother and more efficient list rendering.
Without the trackBy
function, Angular relies solely on object identity to track changes, leading to performance bottlenecks, particularly in scenarios where the list is frequently updated or when dealing with complex data structures.
In addition to performance gains, utilizing the trackBy
function also provides a more maintainable codebase, as it ensures that the rendering logic remains optimized even as the application scales.
Practical Example
Let's illustrate the impact of the trackBy
function with a practical example. Consider a scenario where we have a list of user objects with a 'status' property that is frequently updated, triggering changes in the list. Without using the trackBy
function, every update to the list results in unnecessary re-rendering of all the items, even if the user's details remain the same.
By implementing the trackBy
function to utilize a unique identifier such as the user's ID, Angular can efficiently identify the changed items and update only those specific elements in the list, leading to a noticeable improvement in performance, especially when dealing with a large number of users.
Wrapping Up
In conclusion, the trackBy
function in Angular provides a powerful mechanism to enhance the performance of list rendering by enabling the framework to precisely identify the changed items and minimize unnecessary DOM manipulations. By leveraging the trackBy
function with the *ngFor
directive, developers can optimize the rendering of lists, leading to improved performance and a more responsive user interface.
When working with large datasets or frequently updated lists, incorporating the trackBy
function is essential for maintaining a performant Angular application. By reducing the overhead of unnecessary re-rendering, the trackBy
function contributes to a smoother user experience and a more efficient use of resources.
In your next Angular project, remember to consider the usage of the trackBy
function when dealing with list rendering, and experience the tangible performance benefits it brings to your application.
By implementing the trackBy
function effectively, you can optimize your Angular application and ensure that your users experience a seamless and responsive interface, even when working with dynamic and frequently updated data.
To further deepen your understanding of Angular performance optimization, consider exploring advanced techniques such as lazy loading and tree shaking, which can significantly impact the overall performance of your application.
Implementing performance optimizations not only improves the user experience but also reflects the commitment to delivering efficient and scalable applications in the dynamic world of web development.
Additional Resource: Angular Optimizations Additional Resource: Angular Performance - TrackBy Function