Solving Real-Time Data Push in PWAs
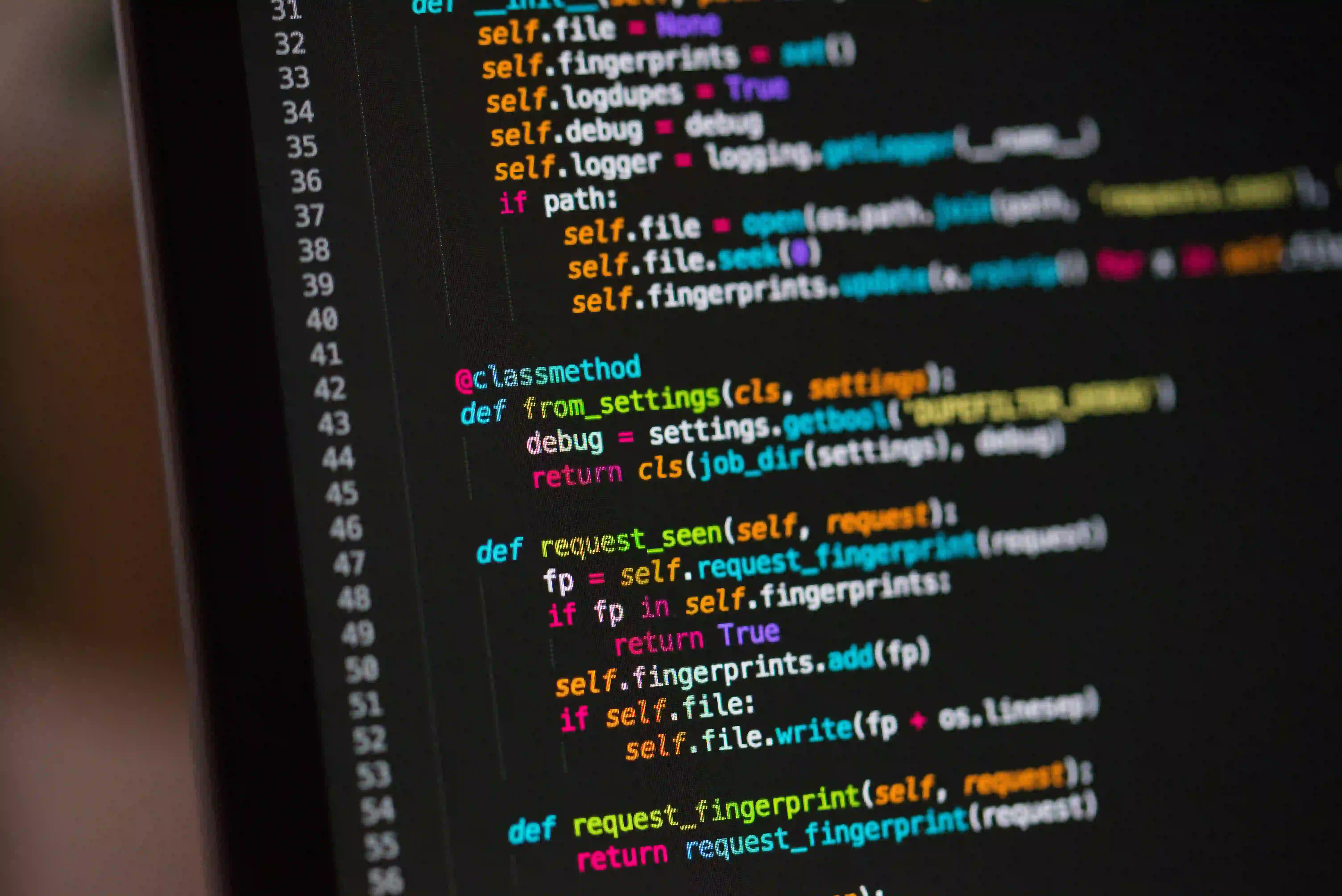
Solving Real-Time Data Push in PWAs
Progressive Web Apps (PWAs) have gained significant popularity due to their ability to deliver a native app-like experience on the web. However, one common challenge faced by developers when building PWAs is enabling real-time data push to keep the user interface up-to-date without manual refreshing. In this article, we'll explore how to solve the real-time data push challenge in PWAs using Java.
Leveraging WebSockets for Real-Time Communication
WebSockets provide a two-way, full-duplex communication channel over a single, long-lived connection between a client and a server. This makes them an ideal choice for enabling real-time data push in PWAs.
In Java, you can leverage the javax.websocket
package to implement WebSocket-based communication. This package provides the necessary APIs for creating WebSocket endpoints and handling WebSocket messages.
Let's take a look at a simple Java WebSocket endpoint that broadcasts real-time updates to connected clients:
import javax.websocket.*;
import javax.websocket.server.ServerEndpoint;
import java.io.IOException;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
@ServerEndpoint(value = "/realtimeupdates")
public class RealTimeUpdatesEndpoint {
private static Set<Session> sessions = Collections.synchronizedSet(new HashSet<>());
@OnOpen
public void onOpen(Session session) {
sessions.add(session);
}
@OnClose
public void onClose(Session session) {
sessions.remove(session);
}
@OnError
public void onError(Throwable error) {
// Error handling logic
}
@OnMessage
public void onMessage(String message, Session session) {
// Handle incoming messages
}
public static void broadcast(String message) {
for (Session session : sessions) {
if (session.isOpen()) {
try {
session.getBasicRemote().sendText(message);
} catch (IOException e) {
// Error handling logic
}
}
}
}
}
In this example, we create a WebSocket endpoint /realtimeupdates
that maintains a set of active sessions. When a client connects (@OnOpen
), its session is added to the set. On disconnection (@OnClose
), the session is removed. The broadcast
method sends a message to all connected clients.
Integrating WebSocket Communication with PWA
Now that we have a WebSocket server implementation in Java, let's integrate it with a PWA to enable real-time data push.
Assuming you have an existing PWA built with HTML, CSS, and JavaScript, you can use the JavaScript WebSocket
API to connect to the Java WebSocket server and receive real-time updates.
const socket = new WebSocket('ws://example.com/realtimeupdates');
socket.onopen = () => {
console.log('WebSocket connection established');
};
socket.onmessage = (event) => {
const data = JSON.parse(event.data);
// Handle the incoming real-time data update
};
socket.onclose = () => {
console.log('WebSocket connection closed');
};
socket.onerror = (error) => {
console.error('WebSocket error: ' + error);
};
In this JavaScript snippet, we create a WebSocket connection to the /realtimeupdates
endpoint on the server. When the connection is established, we log a message. We handle incoming messages in the onmessage
event handler. Additionally, we handle connection close and error events.
Handling Authentication and Security
When dealing with real-time data push in PWAs, it's crucial to ensure the security and authentication of WebSocket connections. You can integrate authentication mechanisms such as JSON Web Tokens (JWT) or OAuth with WebSocket connections to verify the identity of clients connecting to the server.
Here's an example of how you can implement JWT-based authentication for WebSocket connections in Java:
@ServerEndpoint(value = "/realtimeupdates")
public class RealTimeUpdatesEndpoint {
// ...
private static boolean authenticate(String token) {
// Validate the JWT token and return true if valid, false otherwise
// Example: Use a library like jjwt for JWT validation
}
@OnOpen
public void onOpen(Session session, @PathParam("token") String token) {
if (authenticate(token)) {
sessions.add(session);
} else {
// Handle authentication failure
try {
session.close(new CloseReason(CloseReason.CloseCodes.VIOLATED_POLICY, "Authentication failed"));
} catch (IOException e) {
// Error handling logic
}
}
}
// ...
}
In this example, we extract the JWT token from the WebSocket connection URL using @PathParam
and validate it in the onOpen
method. If the authentication fails, we close the session with an appropriate close reason.
The Last Word
By leveraging WebSockets for real-time communication and integrating WebSocket communication with PWAs, you can effectively solve the real-time data push challenge in PWAs. Additionally, ensuring the security of WebSocket connections is essential for protecting the integrity of real-time data exchange.
In conclusion, Java provides robust capabilities for implementing real-time data push in PWAs, allowing developers to create engaging and responsive user experiences.
Remember, leveraging real-time data push in PWAs not only enhances user experience but also opens up possibilities for a wide range of applications, from real-time collaboration tools to live dashboards and notifications.
So, embrace the power of real-time data push in PWAs with Java and elevate your web applications to a whole new level of interactivity and responsiveness.