Managing State in Functional-Style Java
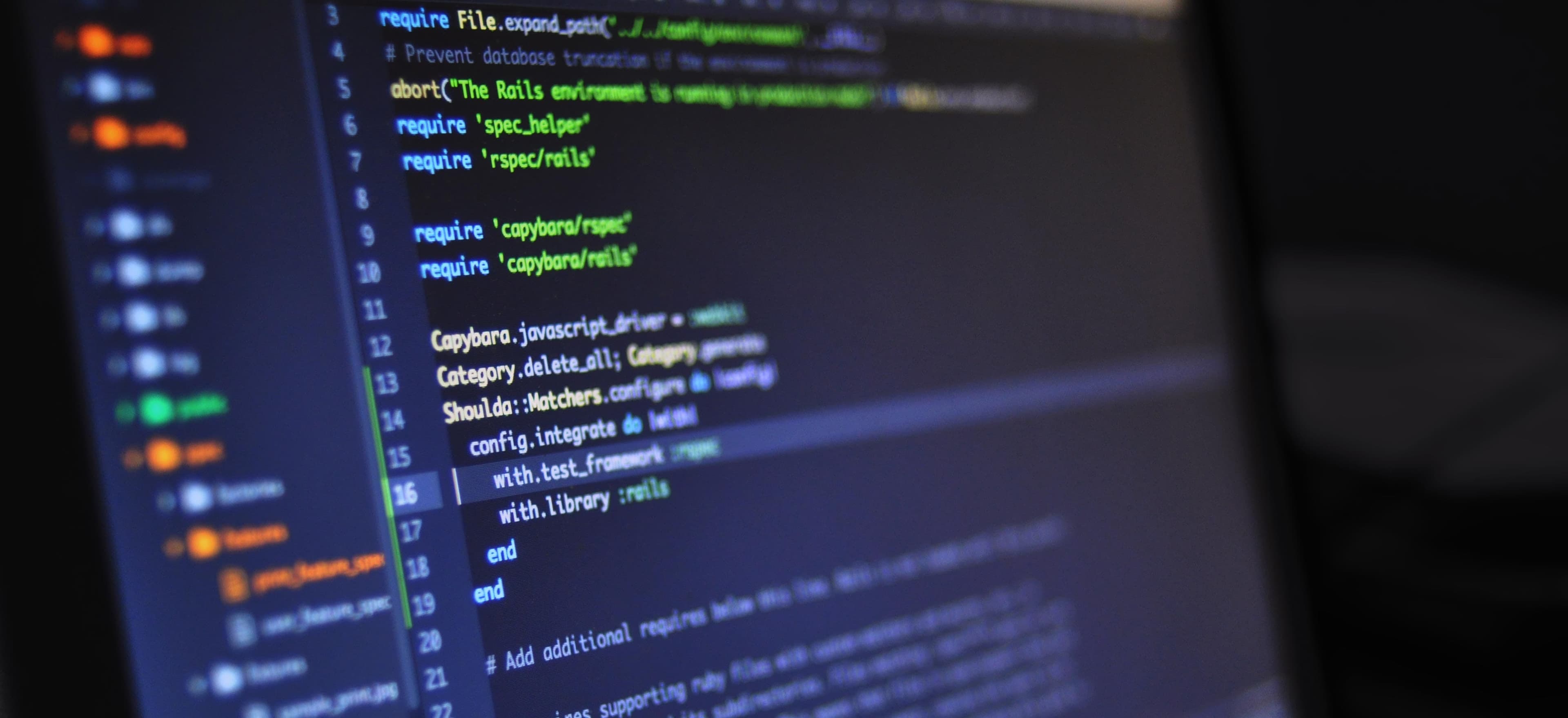
- Published on
Managing State in Functional-Style Java
When it comes to managing state in Java, the traditional approach has been to rely heavily on mutable objects and imperative programming paradigms. However, with the rise of functional programming, there has been a shift towards immutability and a more declarative style of programming.
In this article, we will explore how to manage state in a functional-style Java application, leveraging concepts such as immutability, pure functions, and the java.util.stream
package.
Immutability and State Management
In functional programming, immutability is a key concept. An immutable object is one whose state cannot be modified after it is created. This can lead to more predictable and easier-to-reason-about code, as it eliminates the risk of unexpected state changes.
In Java, we can create immutable classes by following a few simple guidelines:
- Make the class
final
to prevent it from being extended. - Make all fields
private
andfinal
to prevent them from being modified. - Do not provide any mutator methods, and instead initialize the fields through the constructor.
Let's take a look at an example of an immutable class:
public final class ImmutablePerson {
private final String name;
private final int age;
public ImmutablePerson(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In this example, the ImmutablePerson
class is immutable, as its state (i.e., name
and age
) cannot be modified after the object is constructed. This ensures that instances of ImmutablePerson
will always maintain their initial state, making it easier to reason about their behavior.
Pure Functions and Side Effects
Another important concept in functional programming is the idea of pure functions. A pure function is a function that, given the same input, will always produce the same output, and has no side effects. This means that it does not modify any state outside of its own scope.
In Java, we can strive to write pure functions by avoiding the use of mutable state and ensuring that our functions do not have any side effects. This can help make our code more predictable and easier to test and debug.
Let's consider an example of a pure function in Java:
public class PureFunctions {
public static int square(int x) {
return x * x;
}
}
The square
function is a pure function because it always produces the same output for a given input, and it does not modify any state outside of its scope.
Using Streams for Stateful Operations
In Java, the java.util.stream
package provides powerful abstractions for processing sequences of elements in a functional style. Streams allow us to perform stateful operations on collections of data using a declarative syntax, without explicitly mutating the original data.
For example, consider the following code snippet:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * 2)
.reduce(0, Integer::sum);
System.out.println("The sum is: " + sum);
In this example, we use a stream to perform stateful operations on a list of numbers. We filter out the odd numbers, map each even number to its double, and then calculate the sum of the resulting numbers. Throughout this process, the original numbers
list remains unchanged.
By leveraging streams and stateful operations, we can write code that is more concise, expressive, and easier to reason about, while still maintaining a functional style.
The Last Word
In conclusion, managing state in a functional-style Java application involves embracing immutability, writing pure functions, and leveraging stateful operations using streams. By adhering to these principles, we can create code that is more predictable, easier to reason about, and less prone to bugs.
Functional-style programming offers an elegant approach to managing state, and by applying these concepts, we can take full advantage of the features Java has to offer, while writing code that is both performant and maintainable.
For further reading on Java and functional programming, I highly recommend the book "Functional Programming in Java" by Pierre-Yves Saumont.
Thank you for reading! Happy coding!