Repetitive String Operations in Java
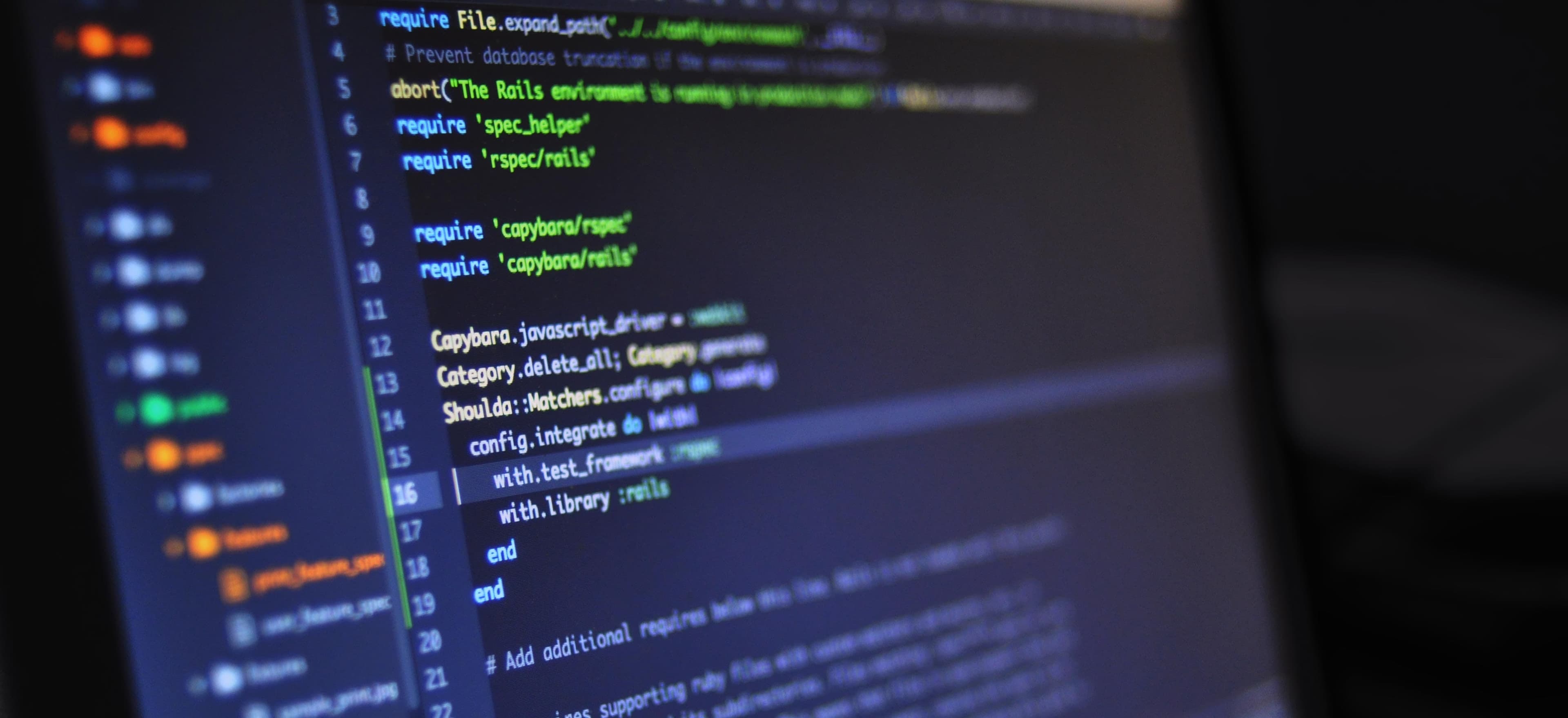
- Published on
Are you tired of writing the same repetitive string operations in your Java code? Fear not! In this post, we'll explore efficient ways to handle common string manipulations using Java and discover how to streamline your code for better readability and performance.
The Struggle with Repetitive String Operations
As Java developers, we often find ourselves performing repetitive string operations such as concatenation, substring extraction, and case conversions. While these operations are fundamental, writing them out over and over again can lead to code duplication, reduced maintainability, and potential performance bottlenecks.
Let's dive into how we can address these issues by leveraging Java's built-in features and libraries to simplify and optimize our string operations.
String Concatenation using StringBuilder
String concatenation using the +
operator can be convenient, but it can lead to inefficient code, especially when dealing with a large number of concatenations. Each +
operation creates a new string object, which can result in unnecessary memory allocation and performance overhead.
Instead, we can use StringBuilder
to efficiently construct strings without the overhead of creating multiple intermediate string objects. Let's consider an example where we want to concatenate a large number of strings:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("hello");
}
String result = sb.toString();
In this example, we use a StringBuilder
to efficiently append the string "hello" 1000 times. By using StringBuilder
, we avoid creating unnecessary intermediate string objects, resulting in better performance and memory usage.
Efficient Substring Extraction with substring()
When working with substrings, it's important to be mindful of memory usage and performance. The substring()
method in Java allows us to extract substrings from a given string, but it's crucial to understand how it works under the hood.
Consider the following code snippet:
String original = "hello world";
String sub = original.substring(6);
In this example, we extract a substring starting from index 6. It's important to note that substring()
does not create a new string but rather shares the original string's underlying character array. This means that holding onto a long-lived substring can prevent the garbage collector from reclaiming the memory associated with the original string, leading to potential memory leaks.
It's crucial to use substring()
judiciously and consider whether the extracted substrings need to be long-lived. If not, consider creating new string instances using the String
constructor to release the reference to the original string's character array.
Case Conversions using toLowerCase() and toUpperCase()
Changing the case of strings is a common task, and Java provides toLowerCase()
and toUpperCase()
methods for this purpose. However, it's essential to be mindful of the locale when performing case conversions.
Consider the following snippet:
String original = "Hello, World";
String lowercase = original.toLowerCase();
String uppercase = original.toUpperCase();
In this example, we convert the original string to lowercase and uppercase. However, it's important to remember that case conversions can behave differently based on the locale. When dealing with locale-sensitive case conversions, it's recommended to use the overloaded versions of toLowerCase()
and toUpperCase()
that accept a Locale
parameter to ensure consistent behavior across different locales.
String lowercase = original.toLowerCase(Locale.ENGLISH);
String uppercase = original.toUpperCase(Locale.ENGLISH);
By explicitly specifying the locale, we can avoid unexpected behavior in case conversions and ensure our code is more robust and predictable.
Leveraging Regular Expressions for Advanced String Manipulation
In addition to the built-in string methods, Java provides robust support for regular expressions through the java.util.regex
package. Regular expressions are powerful tools for advanced string manipulation, allowing us to perform complex search and replace operations, pattern matching, and more.
Let's consider an example where we use a regular expression to extract all the email addresses from a given input string:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
String input = "Contact us at support@example.com or sales@example.com";
Pattern pattern = Pattern.compile("\\b[\\w.-]+@[\\w.-]+\\.\\w+\\b");
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
System.out.println("Found email: " + matcher.group());
}
In this example, we define a regular expression pattern to match email addresses and use a Matcher
to find all matches in the input string. Regular expressions provide a concise and powerful way to handle complex string manipulations, making them a valuable tool in a Java developer's arsenal.
A Final Look
In this post, we've explored various strategies for handling repetitive string operations in Java. By leveraging features such as StringBuilder
for efficient concatenation, carefully managing substring extraction, considering locale in case conversions, and harnessing the power of regular expressions, we can write cleaner, more efficient code.
By optimizing our string operations, we can improve the performance and readability of our code, leading to a more maintainable and robust codebase. So, the next time you find yourself facing repetitive string manipulations, remember to apply these techniques to streamline your Java code.
Happy coding!