Handling File I/O Efficiently in Java 7
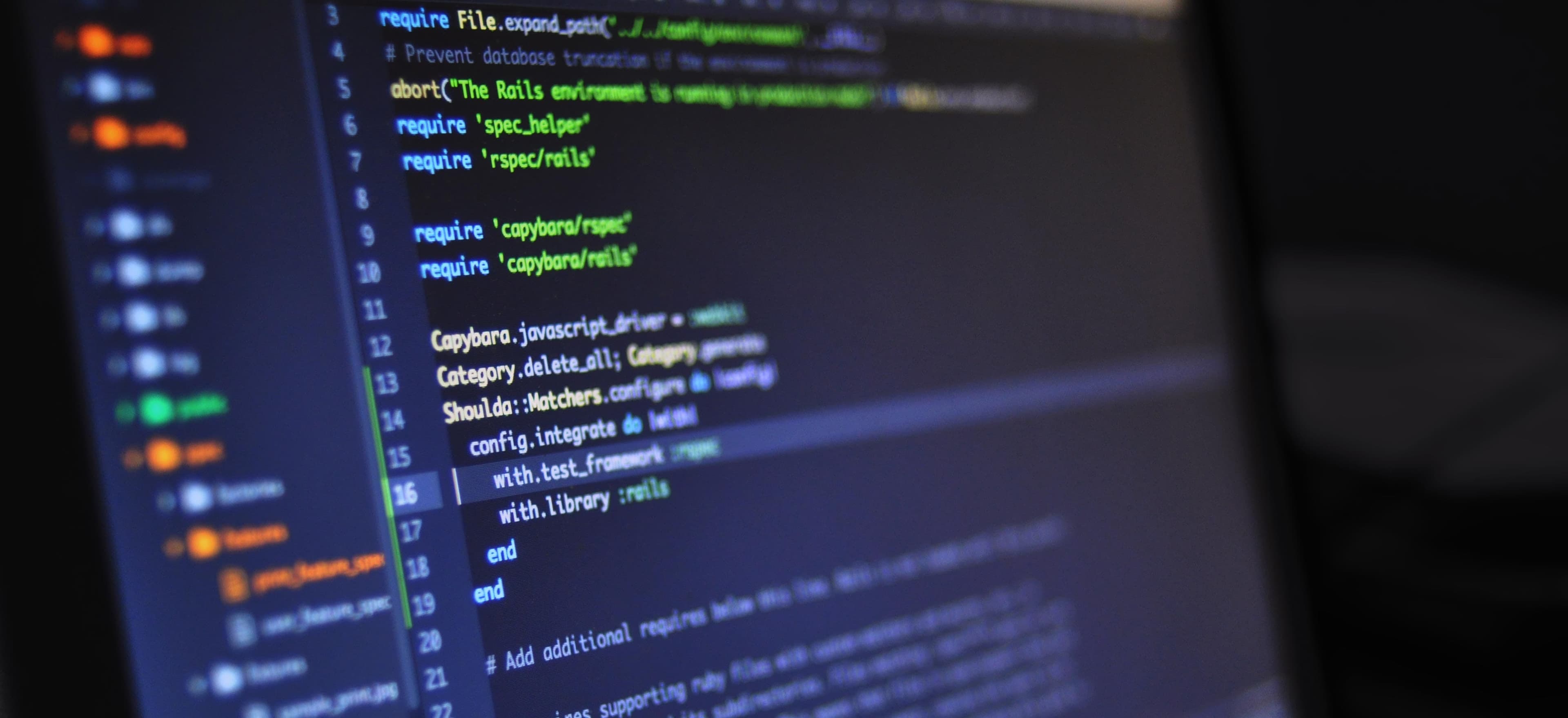
- Published on
Efficient File I/O in Java 7
When developing applications in Java, efficient file input/output (I/O) operations are crucial for enhancing performance and minimizing resource usage. In Java 7, several features and techniques can be leveraged to achieve efficient file I/O. This article will cover some best practices and approaches to handle file I/O efficiently in Java 7.
1. Using try-with-resources Statement
One of the significant enhancements in Java 7 is the introduction of the try-with-resources statement, which simplifies resource management by automatically closing resources such as streams once they are no longer needed. When working with file I/O, it is essential to properly manage file streams to ensure that system resources are released promptly.
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
// Process the file contents
}
} catch (IOException e) {
// Handle exception
}
The try-with-resources statement eliminates the need for explicit resource cleanup code and ensures that file streams are closed gracefully, leading to more efficient file I/O operations.
2. Buffered I/O
Buffered I/O can significantly improve file I/O performance by reducing the number of physical I/O operations. In Java, buffering can be achieved using classes such as BufferedReader
and BufferedWriter
to minimize the overhead associated with frequent disk access.
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
// Read file using buffered I/O
} catch (IOException e) {
// Handle exception
}
try (BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) {
// Write to file using buffered I/O
} catch (IOException e) {
// Handle exception
}
With buffered I/O, data is read or written in larger chunks, reducing the overall I/O overhead and improving the efficiency of file operations.
3. Path and File API
Java 7 introduced the Path and File API, which provides a more modern and flexible way to work with files and directories. The Path class offers a rich set of methods for manipulating file paths, resolving relative paths, and performing various file operations. By using the Path and Files classes, developers can perform file I/O operations in a more efficient and platform-independent manner.
Path sourcePath = Paths.get("source.txt");
Path targetPath = Paths.get("target.txt");
try {
Files.copy(sourcePath, targetPath, StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
// Handle exception
}
The Path and File API in Java 7 simplifies file I/O tasks and provides better support for working with files and directories.
4. NIO.2 File Channel
Java 7's NIO.2 (New I/O) introduced the FileChannel class, which offers a more efficient and flexible way to perform low-level I/O operations on files. FileChannel supports operations such as memory-mapped I/O, direct buffer I/O, and transfer operations, making it suitable for scenarios that require high-performance file I/O.
try (FileChannel sourceChannel = new FileInputStream("source.txt").getChannel();
FileChannel targetChannel = new FileOutputStream("target.txt").getChannel()) {
sourceChannel.transferTo(0, sourceChannel.size(), targetChannel);
} catch (IOException e) {
// Handle exception
}
The NIO.2 File Channel provides a powerful mechanism for efficient file I/O and is particularly beneficial for handling large files and high-throughput applications.
Lessons Learned
Efficient file I/O is vital for optimizing the performance and scalability of Java applications. By leveraging features such as try-with-resources, buffered I/O, Path and File API, and NIO.2 File Channel, developers can enhance the efficiency of file operations while ensuring proper resource management and platform compatibility.
Java 7's advancements in file I/O handling have paved the way for more streamlined and high-performing applications. By adopting best practices and utilizing the available APIs effectively, developers can harness the full potential of efficient file I/O in Java 7.
In conclusion, Java 7 provides a range of features and APIs to handle file I/O efficiently, from try-with-resources for automatic resource management and buffered I/O to the modern Path and File API and the high-performance NIO.2 File Channel. By incorporating these techniques into your Java applications, you can maximize performance and resource utilization while ensuring smooth and efficient file I/O operations.
Would you like to learn more about Java 7 file I/O or how to optimize file handling in later versions of Java? Check out Java I/O Tutorial and Oracle's Java Tutorials.
Checkout our other articles