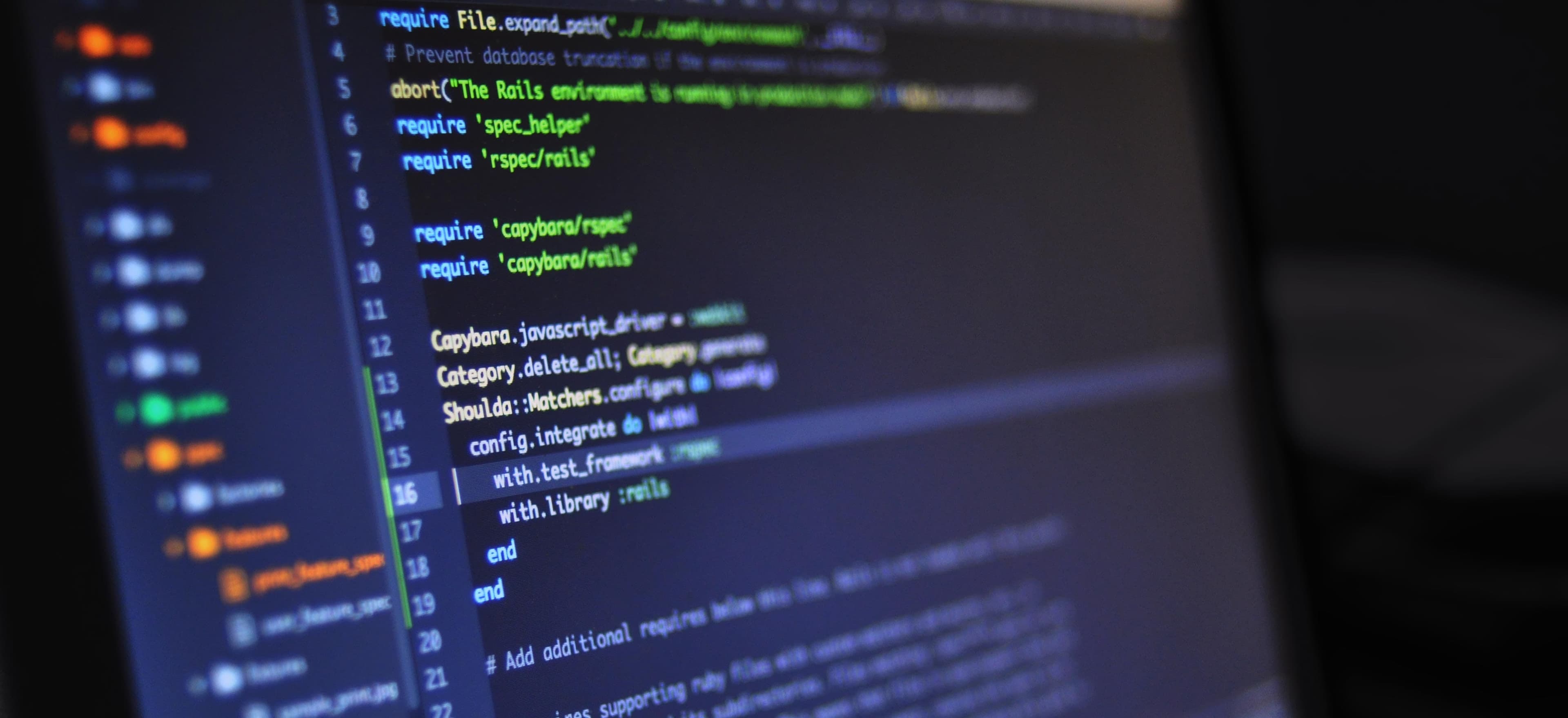
- Published on
Unit Testing Thymeleaf Views in Spring: Best Practices
When developing a web application using Spring, unit testing is a crucial aspect of ensuring the robustness and reliability of the application. While unit testing the backend code is a common practice, testing the presentation layer, specifically Thymeleaf views, is equally important. In this blog post, we will explore the best practices for unit testing Thymeleaf views in a Spring application, leveraging the power of Java and its testing frameworks.
Why Unit Test Thymeleaf Views?
Thymeleaf is a popular server-side Java template engine for web and standalone environments. It is extensively used in Spring-based applications for rendering dynamic HTML content. Unit testing views generated by Thymeleaf helps in verifying their correctness and the data displayed. This ensures that the application's UI functions as intended, and any potential regressions are caught early in the development cycle.
Setting Up the Project
Before delving into writing unit tests for Thymeleaf views, let's set up a basic Spring Boot project. You can use Spring Initializr to generate a new project with the necessary dependencies. In this example, we will include Web
, Thymeleaf
, and Mockito
dependencies.
Writing the Unit Test
Assuming a Spring MVC controller returns a view named index
with some model attributes, we can write a unit test to verify the behavior of this view and the data it renders. Below is a sample JUnit test that accomplishes this using Mockito for mocking dependencies and Spring's MockMvc
for testing the controller:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.model;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.view;
import static org.mockito.Mockito.when;
import static org.springframework.test.web.servlet.setup.MockMvcBuilders.standaloneSetup;
@RunWith(MockitoJUnitRunner.class)
public class ThymeleafViewUnitTest {
@InjectMocks
private YourController yourController;
@Mock
private YourService yourService;
@Test
public void testIndexView() throws Exception {
when(yourService.getData()).thenReturn("MockedData");
MockMvc mockMvc = standaloneSetup(yourController).build();
mockMvc.perform(get("/index"))
.andExpect(status().isOk())
.andExpect(view().name("index"))
.andExpect(model().attribute("data", "MockedData"));
}
}
In the above code snippet, we are mocking the service layer and setting its behavior to return a specific value when called. Then, we use MockMvc
to perform a GET request to the URL mapped by the controller method that returns the index
view. Finally, we assert that the view name is index
and that it has the expected model attribute data
with the value returned by the mocked service.
Assertions and Verifications
When unit testing Thymeleaf views, it's important to make assertions not only on the view name but also on the model attributes being passed to the view. This ensures that the data displayed in the view is correct. Additionally, you can verify the HTML elements and their content for more granular testing. Libraries like Jsoup can be useful for parsing and querying the HTML content in the view for these verifications.
Edge Cases and Error Handling
It's essential to test edge cases and error handling in Thymeleaf views as well. This includes scenarios where the model attributes are null or empty, and validating the behavior of the view in such cases. By covering these edge cases in our unit tests, we can guarantee the resilience of our views in handling unexpected data scenarios.
Integration with Selenium
While unit tests are great for testing the rendering of views and the data they display, they fall short in testing the actual behavior of the web elements and their interactions. For more comprehensive testing involving user interactions, integration with tools like Selenium for end-to-end testing can be beneficial. Selenium allows simulating user actions and validating the behavior of the web application from a user's perspective.
My Closing Thoughts on the Matter
Unit testing Thymeleaf views in a Spring application is an integral part of ensuring the correctness and reliability of the presentation layer. By leveraging Java testing frameworks like JUnit and Mockito, along with Spring's MockMvc, we can effectively verify the behavior of our views and the data they render. Additionally, integrating with tools like Jsoup for HTML parsing and Selenium for end-to-end testing can further enhance the testing coverage of our Thymeleaf views. As a best practice, it's crucial to write comprehensive unit tests for views, encompassing different scenarios and edge cases, to fortify the quality and resilience of the application's UI.
In conclusion, combining the power of Java testing frameworks, leveraging mocking libraries, and integrating with complementary tools provides a robust approach to unit testing Thymeleaf views in a Spring application, ultimately contributing to a solid and reliable application.