Optimizing Java Performance with Intrinsics
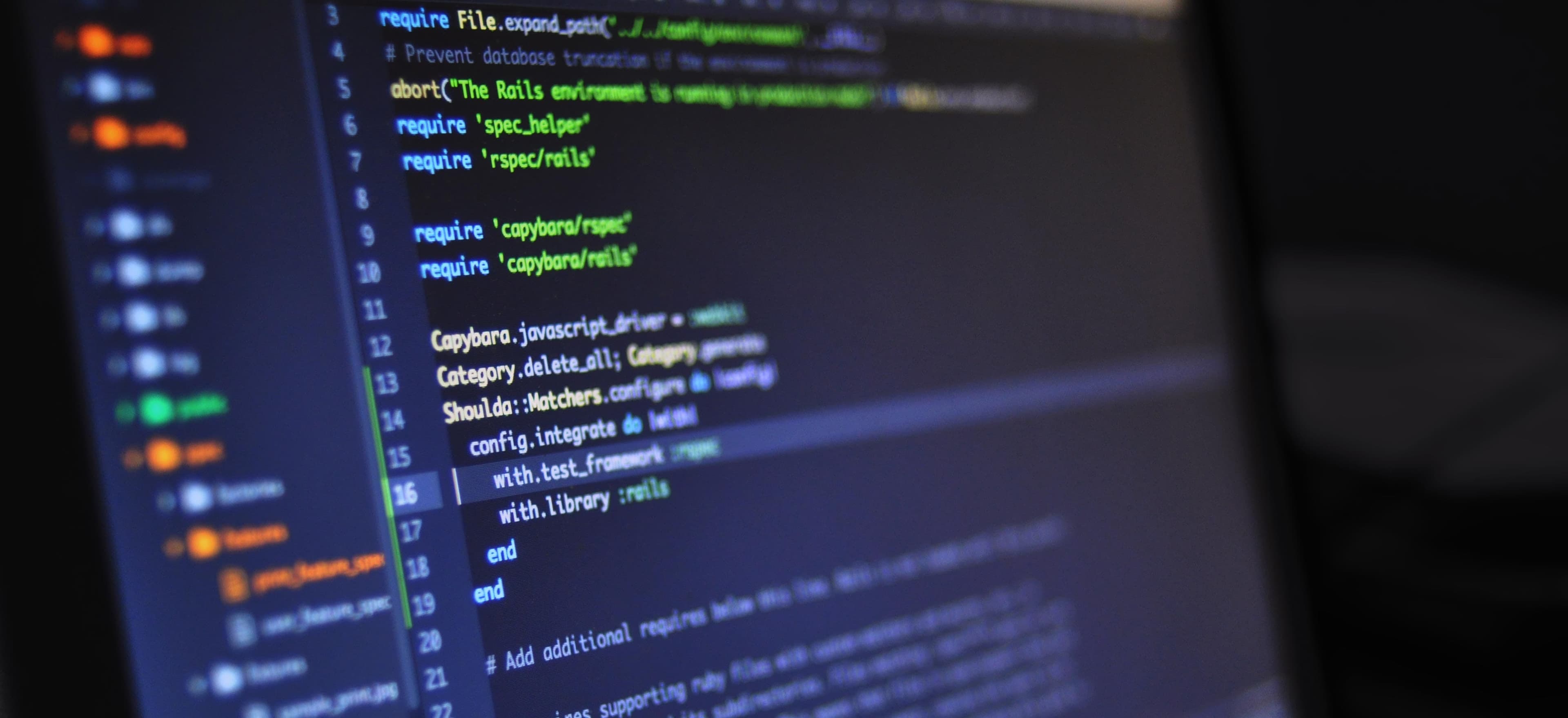
- Published on
Optimizing Java Performance with Intrinsics
Java, as a high-level language, comes with the promise of platform independence and ease of use. However, when it comes to performance-critical tasks, Java can sometimes lag behind languages like C or C++. This is where Java Intrinsics come into play. Intrinsics allow Java developers to write platform-specific optimized code for critical operations, providing a significant performance boost. In this article, we will explore the concept of Java Intrinsics, how they work, and how they can be leveraged to optimize performance in Java applications.
Understanding Java Intrinsics
Java Intrinsics allow the JVM to replace certain method calls with hand-written assembly code, thereby improving performance for critical operations. These operations are typically related to low-level tasks such as arithmetic, bit manipulation, and memory operations.
One of the most significant advantages of using Java Intrinsics is that it enables developers to write code that can be optimized for a specific hardware platform. This means that the performance enhancements offered by Intrinsics can be tailored to take advantage of the unique capabilities of the underlying hardware, such as SIMD (Single Instruction, Multiple Data) instructions on modern CPUs.
How Intrinsics Work
When a method marked with an intrinsic annotation is called, the JVM can replace the method call with a native function that directly interacts with the underlying hardware. This allows the JVM to bypass the overhead of interpreting and executing bytecode, resulting in improved performance for the specific operation.
Intrinsics are defined using the @HotSpotIntrinsicCandidate
annotation in the java.lang.reflect
package. This annotation marks a method as a potential candidate for intrinsic expansion by the HotSpot JIT compiler. It indicates to the JVM that the method is a good candidate for replacement with hand-written assembly code to improve performance.
Leveraging Intrinsics for Performance Optimization
To demonstrate the concept of using Java Intrinsics for performance optimization, let's consider a common scenario where performance is critical: mathematical operations on arrays. For this example, we will focus on optimizing the sum of elements in an array.
Traditional Approach
public class ArraySum {
public static int sum(int[] array) {
int sum = 0;
for (int value : array) {
sum += value;
}
return sum;
}
}
In the traditional approach, the sum of elements in the array is calculated using a simple loop. While this approach works fine for small arrays, it might not offer optimal performance for large arrays due to the overhead of array access and addition operations in Java bytecode.
Using Java Intrinsics
To optimize the array sum operation using Java Intrinsics, we can leverage the java.util.Arrays
class, which provides an intrinsic method for calculating the sum of elements in an array.
public class ArraySum {
public static int sum(int[] array) {
return java.util.Arrays.stream(array).sum();
}
}
In this optimized approach, the java.util.Arrays.stream(array).sum()
method call is a candidate for intrinsic expansion by the HotSpot JIT compiler. It can be replaced with a highly optimized native function that directly operates on the array elements, leading to improved performance, especially for large arrays.
When to Use Java Intrinsics
While Java Intrinsics offer significant performance benefits for critical operations, it is essential to use them judiciously. Here are some key considerations for deciding when to use Java Intrinsics:
-
Performance-critical Operations: Use Java Intrinsics for operations that are performance-critical and can benefit from low-level optimization. Examples include mathematical computations, bit manipulation, and memory-intensive tasks.
-
Platform-specific Optimization: Use Java Intrinsics when you need to take advantage of the unique capabilities of the underlying hardware, such as SIMD instructions on modern CPUs.
-
Compatibility: Be mindful of platform independence when using Intrinsics. If your application needs to run on multiple platforms, ensure that the intrinsic functions have fallback implementations for non-supported platforms.
-
Maintainability: Consider the trade-off between performance optimization and code maintainability. Intrinsics can introduce platform-specific code, which may require careful maintenance and testing.
Key Takeaways
In conclusion, Java Intrinsics offer a powerful mechanism for optimizing performance-critical operations in Java applications. By leveraging platform-specific optimizations and low-level hand-written assembly code, developers can achieve significant performance improvements for targeted operations. When used judiciously, Java Intrinsics can be a valuable tool for optimizing performance without sacrificing the platform independence and ease of use that Java is known for.
By understanding the concepts of Java Intrinsics, how they work, and when to use them, Java developers can unlock the full potential of the language when it comes to performance optimization.
For further reading on Java Intrinsics, you can refer to the official documentation on Java Platform, Standard Edition Intrinsics.
Remember, always profile your code before attempting optimization, as the benefits of using Intrinsics may vary depending on the specific use case and hardware environment. Happy optimizing!