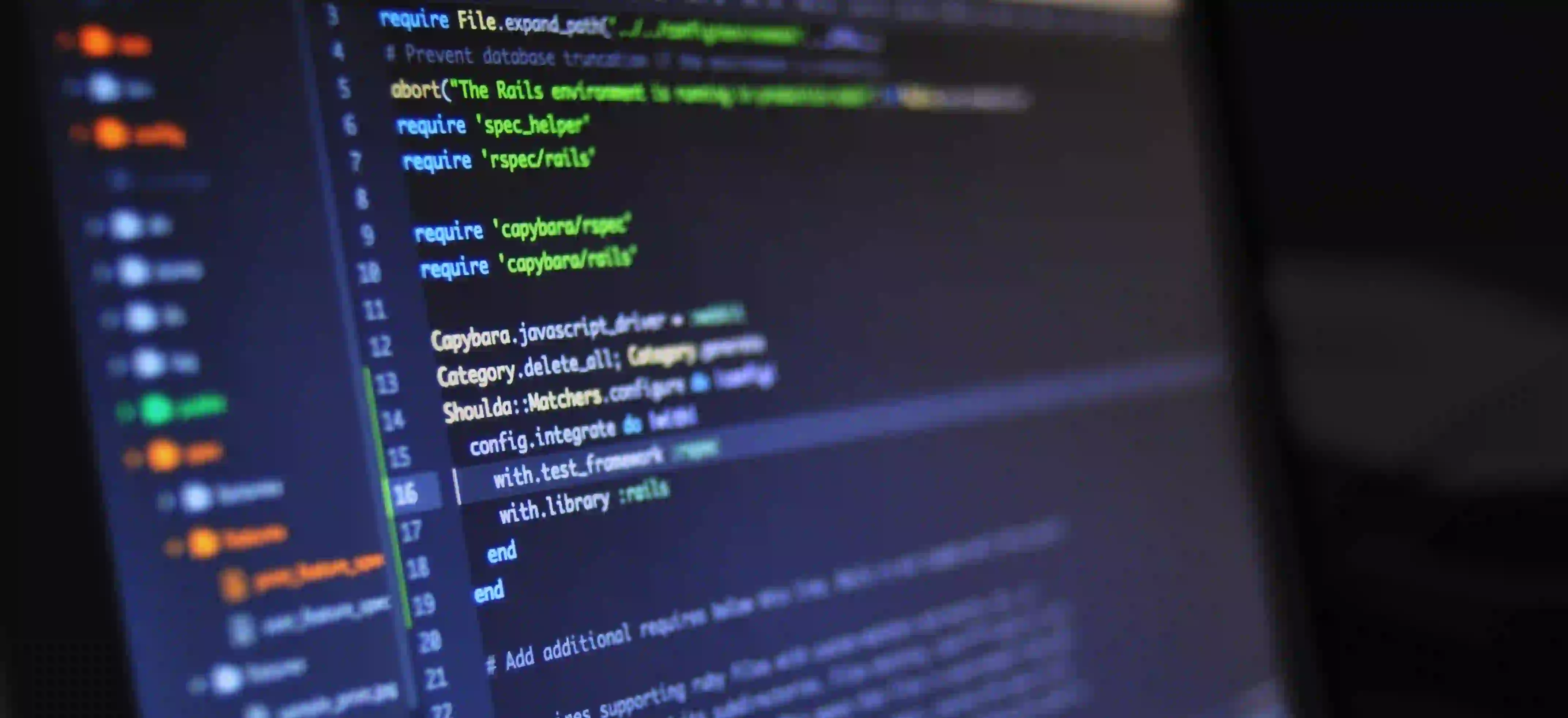
Managing Fragment Transactions and Backstack in Android
When it comes to building robust and flexible Android applications, proper management of fragment transactions and the backstack is crucial. Fragments play an essential role in creating dynamic and modular user interfaces, and understanding how to effectively manage their transactions and backstack can greatly improve the user experience. In this article, we'll delve into the best practices for managing fragment transactions and the backstack in Android applications using Java.
Understanding Fragment Transactions
In Android, a fragment represents a behavior or a portion of a user interface. Fragment transactions are the fundamental operations for adding, removing, replacing, or modifying fragments within an activity.
Let's take a look at a simple example of adding a fragment to an activity using a transaction:
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
MyFragment fragment = new MyFragment();
fragmentTransaction.add(R.id.fragment_container, fragment, "MY_FRAGMENT");
fragmentTransaction.commit();
In this code snippet, we obtain an instance of the FragmentManager
, begin a new transaction, create an instance of our fragment, add it to the activity layout, and commit the transaction.
It's essential to understand that fragment transactions are not immediately executed. Instead, they are scheduled to run on the next iteration of the activity's event loop. This means that multiple operations can be batched together and executed at once, which is more efficient.
Managing Fragment Backstack
The fragment backstack is a powerful feature in Android that allows users to navigate through previously visited fragments by pressing the back button. Each fragment transaction can be added to the backstack, enabling users to efficiently navigate through the app’s history.
To add the transaction to the backstack, you simply call addToBackStack()
before committing the transaction:
fragmentTransaction.addToBackStack(null);
By adding the transaction to the backstack, when the user presses the back button, the most recent fragment transaction is reversed, and the user is taken to the previous state of the app.
Best Practices for Managing Fragment Transactions and Backstack
1. Use Named Transactions
When adding transactions to the backstack, it's beneficial to provide a name for the transaction:
fragmentTransaction.addToBackStack("MY_TRANSACTION");
A named transaction allows you to pop the backstack to a specific transaction state, facilitating more control over the fragment navigation flow.
2. Handle Back Button Press
Proper handling of the back button press is essential when managing the backstack. Override the onBackPressed
method in your activity to define custom behavior when the back button is pressed. This allows you to intercept the back button press and customize the navigation flow as per your application's requirements.
3. Ensure Consistent UX
Ensure that the fragment transactions and backstack management provide a consistent and intuitive user experience. Unexpected behavior, such as erratic fragment transitions or improper backstack management, can lead to a confusing user experience.
4. Test Backstack Navigation
Thoroughly test the backstack navigation to ensure that the app behaves as expected when the user navigates through the fragments using the back button.
The Bottom Line
Effective management of fragment transactions and the backstack is crucial for building seamless user experiences in Android applications. By following best practices, such as utilizing named transactions, handling back button presses, ensuring consistency in the user experience, and thorough testing, developers can create robust and user-friendly apps.
Properly managing fragment transactions and the backstack not only enhances the user experience but also contributes to the overall stability and maintainability of the Android application.
In conclusion, mastering the art of fragment transaction and backstack management is an essential skill for any Android developer aiming to build high-quality, responsive applications.
For further reading, refer to the Android Developer Guide for a comprehensive understanding of fragment transactions and backstack management in Android.
Remember, a well-structured backstack and seamless fragment transitions can make the difference between a good app and a great app. Happy coding!
Implementing proper fragment transactions and backstack handling can greatly improve the usability of an Android application. By understanding the nuances of these operations, developers can create seamless user experiences and maintain clean code structures. Do you have any tips or experiences to share regarding fragment transactions and backstack management? Let's discuss in the comments below!