Optimizing Pipeline Efficiency in Jenkinsfile
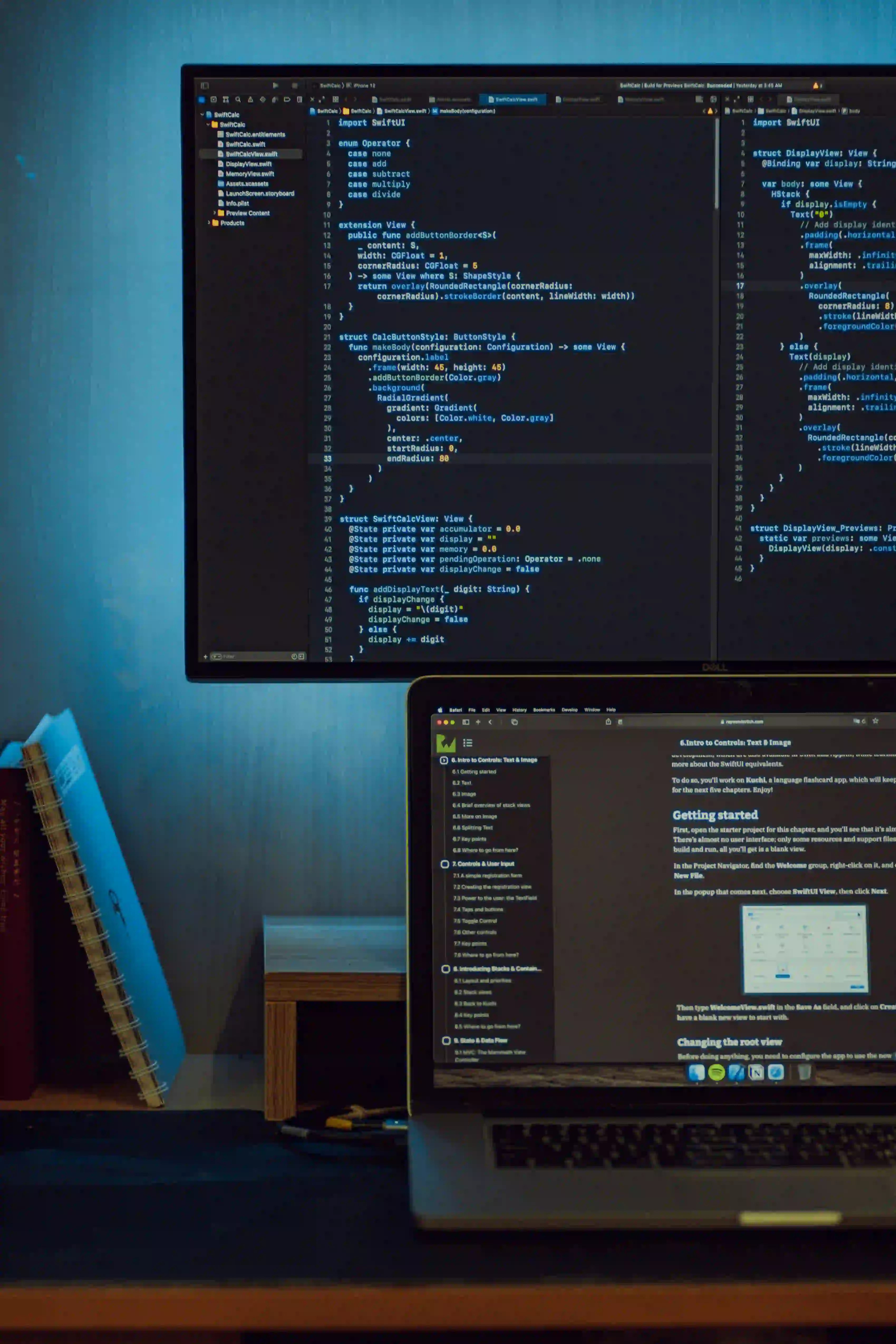
Optimizing Pipeline Efficiency in Jenkinsfile
In the realm of DevOps, Jenkins plays a pivotal role in automating the software delivery process. Its declarative pipeline feature, powered by Jenkinsfile, allows developers to define efficient and structured pipelines as code. However, as pipelines grow in complexity and scale, optimization becomes crucial to ensure swift and efficient processes.
In this post, we will explore various techniques to optimize the efficiency of Jenkins pipelines defined in Jenkinsfile, from reducing build times to minimizing resource utilization.
1. Parallel Execution
When dealing with tasks that are independent of each other, parallel execution can significantly reduce build times. Jenkins provides a straightforward way to achieve this within a Jenkinsfile using the parallel
step.
Consider this example where two separate tasks, build
and unitTest
, can be executed concurrently:
pipeline {
agent any
stages {
stage('Parallel Execution') {
steps {
script {
parallel(
build: {
// Execute build task
},
unitTest: {
// Execute unit tests
}
)
}
}
}
}
}
In this scenario, both build
and unitTest
stages will run simultaneously, effectively reducing the overall pipeline execution time.
2. Workspace Cleanup
Over time, workspaces tend to accumulate unnecessary files, consuming disk space and impacting build performance. To address this, it's vital to incorporate workspace cleanup steps within the pipeline.
Below, a simple example demonstrates how to clean up the workspace before the build starts:
pipeline {
agent any
stages {
stage('Workspace Cleanup') {
steps {
deleteDir() // Clean up workspace
}
}
stage('Build') {
steps {
// Build steps
}
}
}
}
By including deleteDir()
at the beginning of the pipeline, redundant files and directories are removed, resulting in a leaner workspace and optimized build performance.
3. Build Cache Utilization
Jenkins offers the capability to cache dependencies and intermediate build outputs, further enhancing build performance. The stash
and unstash
steps can be leveraged to persist and retrieve files respectively, thereby reducing redundant computations.
The following snippet demonstrates how to utilize build caching in Jenkinsfile:
pipeline {
agent any
stages {
stage('Cache Dependencies') {
steps {
script {
// Cache dependencies
stash(name: 'deps', includes: 'path/to/dependencies')
}
}
}
stage('Build') {
steps {
script {
// Retrieve dependencies from cache
unstash 'deps'
// Build steps
}
}
}
}
}
By caching dependencies in the deps
stash, subsequent builds can utilize this cached data, thereby accelerating the build process.
4. Declarative Agent Selection
Efficient agent utilization is crucial for optimal resource allocation. In Jenkinsfile, the agent
directive allows specifying where the pipeline runs. Leveraging this effectively can minimize resource contention and enhance pipeline efficiency.
Consider the following example, where pipeline stages are executed on different agents based on their resource requirements:
pipeline {
agent none
stages {
stage('Build on Linux') {
agent {
label 'linux'
}
steps {
// Build steps for Linux
}
}
stage('Test on Windows') {
agent {
label 'windows'
}
steps {
// Test steps for Windows
}
}
}
}
Here, the agent
directive is tailored to the specific requirements of each stage, ensuring optimal utilization of resources and expediting the pipeline execution.
5. Reusable Function Libraries
As pipelines grow in complexity, leveraging reusable functions and libraries can streamline pipeline development and maintenance. Jenkins Shared Libraries enable the creation of custom pipeline steps, which can be reused across multiple pipeline definitions.
Let's consider a scenario where a custom function for sending notifications is defined in a shared library:
// Shared Library: vars/sendNotification.groovy
def call(String message) {
// Send notification logic
}
This function can then be utilized in Jenkinsfile as follows:
@Library('shared-library') _
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build steps
}
}
stage('Notify') {
steps {
sendNotification('Build completed')
}
}
}
}
By centralizing common pipeline functionality in reusable libraries, Jenkins pipelines maintain conciseness and promote maintainability.
In conclusion, optimizing the efficiency of Jenkins pipelines defined in Jenkinsfile is essential for streamlining the software delivery process. By implementing parallel execution, workspace cleanup, build caching, efficient agent selection, and reusable function libraries, developers can significantly enhance the speed and effectiveness of their pipelines.
By incorporating these optimization techniques into Jenkinsfile, development teams can ensure smooth and efficient pipeline executions, leading to accelerated software delivery and enhanced DevOps workflows.
Implementing these techniques in your Jenkinsfile will translate to more streamlined and productive development workflows.
Do you have any experience with optimizing Jenkins pipelines? Let's discuss in the comments below!