Common Pitfalls When Building Your First Jakarta EE 8 Service
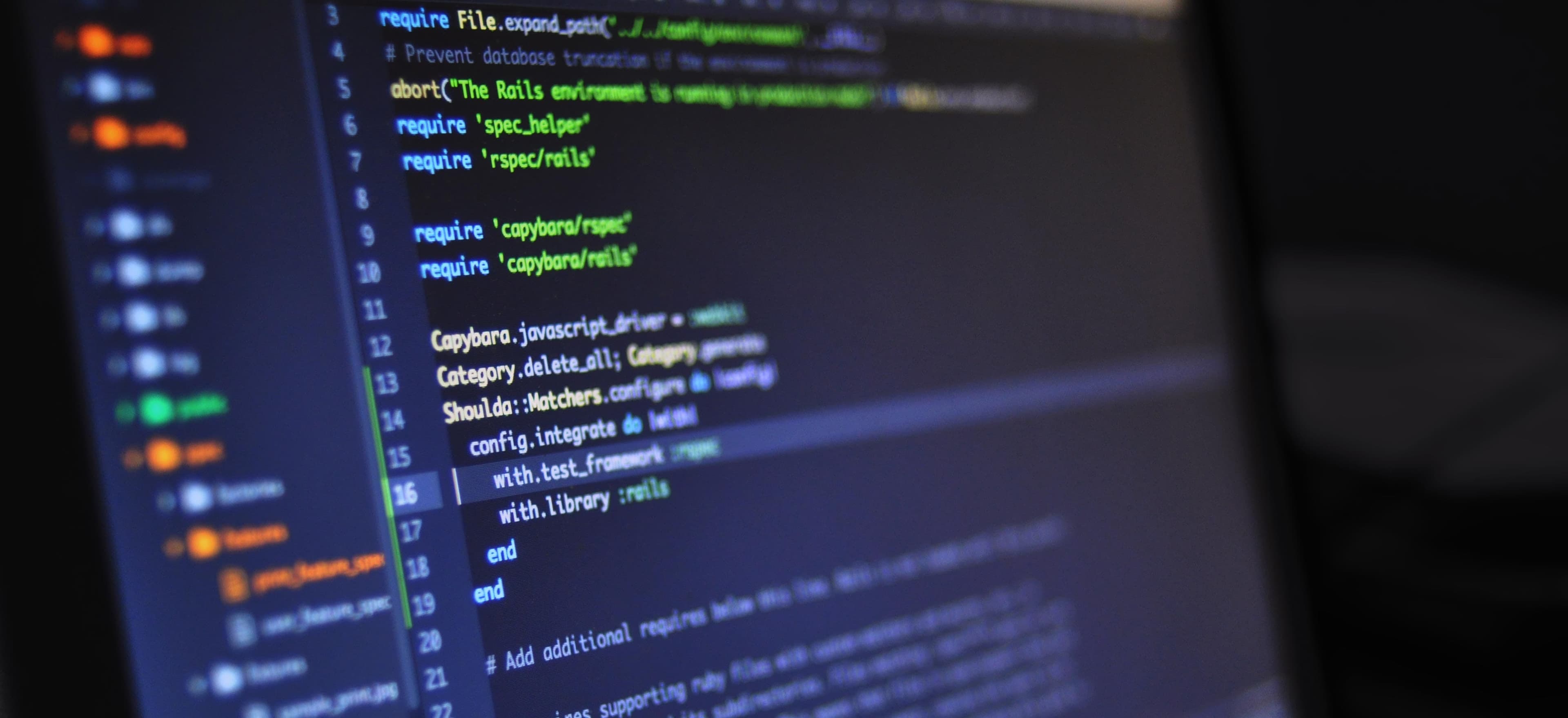
- Published on
Common Pitfalls When Building Your First Jakarta EE 8 Service
Jakarta EE (formerly Java EE) 8 is a powerful framework that provides developers with a robust model for building enterprise-level applications. However, diving into this expansive environment can be daunting for beginners. In this post, we will explore common pitfalls when building your first Jakarta EE 8 service and how to avoid them. Whether you are new to the world of enterprise Java or looking to improve your expertise, this guide will help you navigate the complexities of Jakarta EE 8.
Understanding Jakarta EE 8
Before we delve into the pitfalls, let's briefly understand what Jakarta EE 8 entails:
Jakarta EE 8 is a set of specifications that extend the Java SE (Standard Edition) API with specifications for enterprise features such as dependency injection, web services, and transactions. Notably, it provides APIs like JAX-RS for building RESTful web services and CDI for context and dependency injection.
Now, let's explore the pitfalls.
1. Ignoring Configuration Management
Why This Matters
In Jakarta EE, configuration settings can significantly affect application behavior. Ignoring or mishandling configuration files can lead to hard-to-diagnose issues.
Avoiding This Pitfall
Make sure to leverage configuration management effectively. Utilize the microprofile-config
specification where appropriate. Create a dedicated config.properties
file that can define key-value pairs central to your service.
Here’s an example of how to load a configuration value using MicroProfile Config:
import javax.inject.Inject;
import org.eclipse.microprofile.config.inject.ConfigProperty;
public class MyService {
@Inject
@ConfigProperty(name = "my.service.url", defaultValue = "http://localhost")
private String serviceUrl;
public void execute() {
System.out.println("Service URL: " + serviceUrl);
}
}
The value of my.service.url
can be modified without changing the source code. This promotes flexibility and better maintenance.
2. Misunderstanding Dependency Injection
Why This Matters
Jakarta EE heavily relies on dependency injection (DI). Misusing or misunderstanding DI can lead to tightly coupled code, which will be challenging to maintain or test.
Avoiding This Pitfall
Familiarize yourself with CDI concepts. Utilize annotations like @Inject
to manage dependencies.
For instance, consider the following code snippet:
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Inject;
@ApplicationScoped
public class UserService {
@Inject
private UserRepository userRepository;
public User findUser(Long id) {
return userRepository.findById(id);
}
}
With DI, UserRepository
can be easily replaced with a mock or a different implementation when unit testing, which enhances testability.
3. Neglecting the CDI Lifecycle
Why This Matters
Jakarta EE applications maintain a complex lifecycle. Misunderstanding the lifecycle of CDI beans can lead to memory leaks or unexpected behavior.
Avoiding This Pitfall
Understand the different scopes available in CDI, such as @RequestScoped
, @SessionScoped
, and @ApplicationScoped
.
For example, the following code defines a @RequestScoped
bean:
import javax.enterprise.context.RequestScoped;
@RequestScoped
public class RequestService {
public void performAction() {
// This method will only exist for the duration of a single request
}
}
Using the correct scope for your services will ensure that resources are managed correctly, and instances are created and destroyed as needed.
4. Hardcoding Configuration Values
Why This Matters
Hardcoding configuration values in your code can make it difficult to manage different environments (e.g., development, staging, production).
Avoiding This Pitfall
Always externalize your configuration settings using environment variables, configuration files, or JNDI resources.
Consider how the previous example can be adjusted to pull configurations from environment variables:
@ConfigProperty(name = "DATABASE_URL")
private String dbUrl;
This approach lets your application adapt easily across various deployment environments.
5. Not Utilizing JAX-RS Properly
Why This Matters
JAX-RS is crucial for developing REST APIs in Jakarta EE. Misunderstanding JAX-RS can lead to poorly structured APIs that do not adhere to the principles of REST.
Avoiding This Pitfall
Follow REST principles closely. Use proper HTTP methods (GET, POST, PUT, DELETE) and status codes. Define resource endpoints clearly.
Here's an example illustrating how to create a resource with JAX-RS:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/users")
public class UserResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<User> getAllUsers() {
return UserService.getAllUsers();
}
}
This endpoint adheres to RESTful principles, allowing clients to retrieve user data effectively.
6. Neglecting Error Handling
Why This Matters
Failure to implement robust error handling can result in server crashes or unhelpful error messages for users.
Avoiding This Pitfall
Leverage exception mappers provided by JAX-RS to handle errors globally. Here's an example:
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class GenericExceptionMapper implements ExceptionMapper<WebApplicationException> {
@Override
public Response toResponse(WebApplicationException exception) {
return Response.status(exception.getResponse().getStatus())
.entity("Custom error message: " + exception.getMessage())
.build();
}
}
This approach enhances user experience by giving meaningful feedback about the error.
7. Overcomplicating Project Structure
Why This Matters
Having an overly complicated project structure can create confusion and hinder development.
Avoiding This Pitfall
Stick to a clean, modular architecture. For Jakarta EE projects, it's sensible to follow a standard directory structure. A simple layout might look like this:
src/
├── main/
│ ├── java/
│ │ └── com/
│ │ └── yourapp/
│ ├── resources/
│ └── webapp/
│ └── WEB-INF/
└── test/
Keeping your project organized will streamline collaboration and make future maintenances easier.
8. Failing to Implement Logging
Why This Matters
Logging is essential for tracking application behavior and diagnosing issues. Neglecting to implement proper logging can lead to challenges in maintenance.
Avoiding This Pitfall
Use Jakarta EE's integrated logging capabilities. For instance, Java Util Logging can be adopted like this:
import java.util.logging.Logger;
public class MyService {
private static final Logger logger = Logger.getLogger(MyService.class.getName());
public void performAction() {
logger.info("Action performed.");
}
}
Effective logging will not only help in debugging but also assist in monitoring the application's performance.
Key Takeaways
Building your first Jakarta EE 8 service can be challenging, but by recognizing these common pitfalls, you can enhance the quality and maintainability of your application.
Remember that practice makes perfect. Leverage resources and documentation along the way:
By understanding the framework's capabilities and limitations, you can create robust and efficient enterprise applications that thrive in a competitive landscape. Happy coding!