Optimizing Elasticsearch Indexing Performance
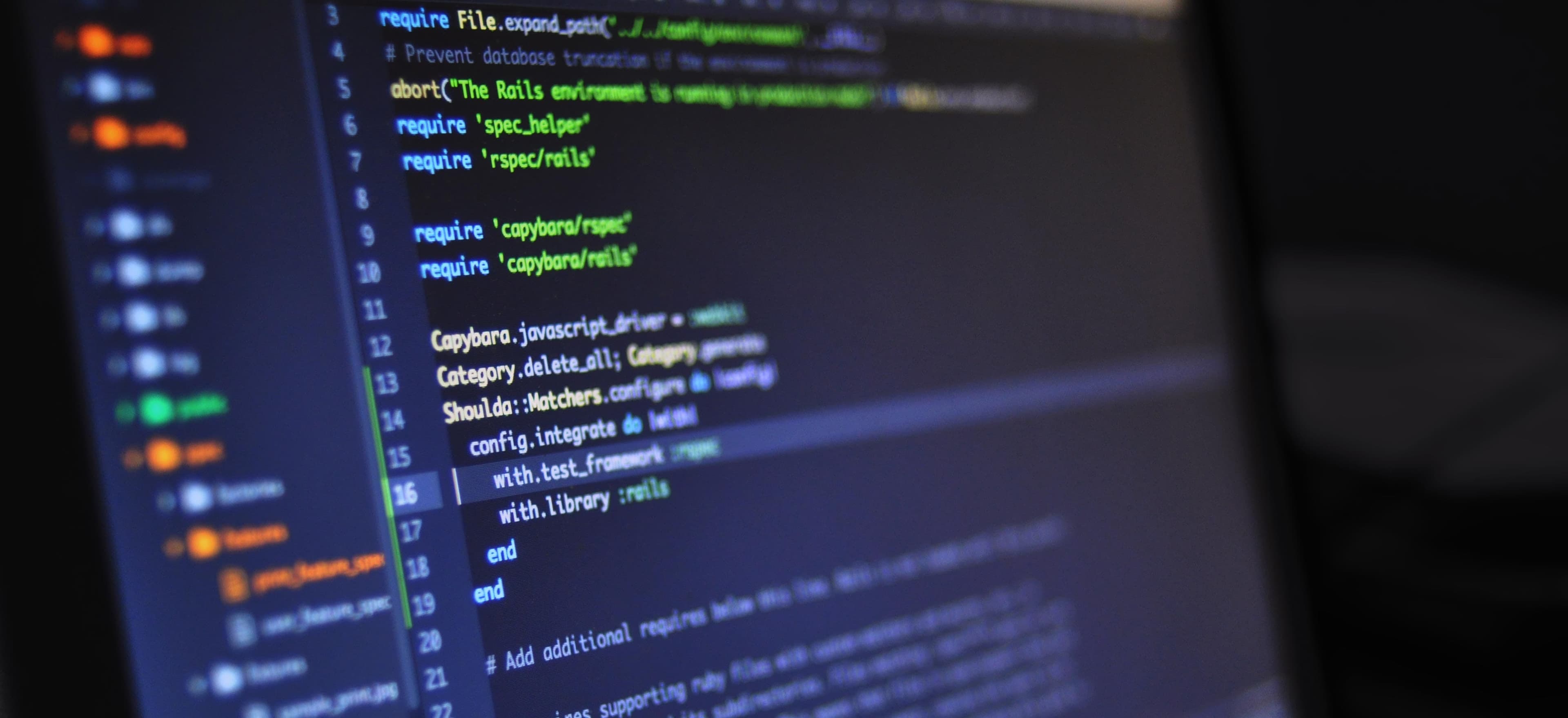
- Published on
Optimizing Elasticsearch Indexing Performance
When it comes to dealing with large volumes of data in Elasticsearch, optimizing indexing performance is crucial. Indexing refers to the process of adding new documents to an index or updating existing documents. In this article, we'll explore various strategies and best practices for optimizing indexing performance in Elasticsearch, leveraging the power of Java.
Understanding Indexing Performance
Before we dive into optimization techniques, it's important to have a clear understanding of what affects indexing performance in Elasticsearch:
- Document Size: The size of the documents being indexed can have a significant impact on performance.
- Bulk Requests: Sending data to Elasticsearch in bulk helps reduce overhead and improves performance.
- Index Settings: Configuring index settings, such as the number of shards and replicas, can affect indexing performance.
- Hardware and Network: The underlying hardware and network infrastructure play a crucial role in determining indexing performance.
Leveraging the Elasticsearch Java API
Elasticsearch provides a robust Java API that allows developers to interact with Elasticsearch programmatically. Leveraging the Java API enables fine-grained control over the indexing process and paves the way for performance optimizations.
Batch Processing with Bulk API
One of the most effective ways to optimize indexing performance is to use the Bulk API for batch processing. The Bulk API allows multiple index, delete, or update operations to be performed in a single request, reducing the overhead of individual requests.
BulkRequest bulkRequest = new BulkRequest();
bulkRequest.add(new IndexRequest("indexName").id("1").so...());
bulkRequest.add(new IndexRequest("indexName").id("2").so...());
bulkRequest.add(new DeleteRequest("indexName").id("3"));
BulkResponse bulkResponse = client.bulk(bulkRequest, RequestOptions.DEFAULT);
In the above code snippet, we create a BulkRequest
and add multiple index and delete requests to it. This approach minimizes network overhead and can significantly improve indexing throughput.
Asynchronous Indexing with Java CompletableFuture
To further boost indexing performance, Java's CompletableFuture
can be utilized for asynchronous indexing operations. Asynchronous indexing allows the application to continue processing other tasks while the indexing operation is in progress, leading to better overall throughput.
CompletableFuture<Void> indexRequest1 = CompletableFuture.runAsync(() -> {
// Index document 1
IndexResponse response = client.index(indexRequest, RequestOptions.DEFAULT);
});
CompletableFuture<Void> indexRequest2 = CompletableFuture.runAsync(() -> {
// Index document 2
IndexResponse response = client.index(indexRequest, RequestOptions.DEFAULT);
});
CompletableFuture<Void> deleteRequest = CompletableFuture.runAsync(() -> {
// Delete document
DeleteResponse response = client.delete(deleteRequest, RequestOptions.DEFAULT);
});
CompletableFuture.allOf(indexRequest1, indexRequest2, deleteRequest).join();
In this example, we use CompletableFuture
to perform indexing and deletion operations asynchronously, allowing for concurrent processing of multiple indexing tasks, thereby improving overall performance.
Tuning Elasticsearch Index Settings
Configuring index settings can have a significant impact on indexing performance. Let's explore some important settings and how they can be optimized.
Sharding and Replication
The number of shards and replicas for an index impacts indexing performance and data availability. While creating an index, it's crucial to determine the appropriate number of primary shards based on the data volume and hardware capabilities.
CreateIndexRequest request = new CreateIndexRequest("indexName");
request.settings(Settings.builder()
.put("index.number_of_shards", 5)
.put("index.number_of_replicas", 1)
);
CreateIndexResponse createIndexResponse = client.indices().create(request, RequestOptions.DEFAULT);
In the above code snippet, we use the CreateIndexRequest
to specify the number of shards and replicas for the index. Properly configuring these settings can significantly impact indexing performance and search speed.
Refresh Interval and Bulk Size
Another crucial aspect of tuning index settings is adjusting the refresh interval and bulk size. The refresh interval determines how often newly indexed documents are made available for search, while the bulk size specifies the number of documents processed in each bulk request.
UpdateSettingsRequest request = new UpdateSettingsRequest("indexName");
request.settings(Settings.builder()
.put("index.refresh_interval", "30s")
.put("index.translog.flush_threshold_size", "1gb")
);
client.indices().putSettings(request, RequestOptions.DEFAULT);
In this example, we use the UpdateSettingsRequest
to modify the refresh interval and translog flush threshold size, thereby fine-tuning the indexing behavior for improved performance and resource utilization.
Monitoring and Profiling
Constant monitoring and profiling of the indexing process are essential for identifying bottlenecks and optimizing performance. Elasticsearch provides various monitoring and profiling tools that can be leveraged to gain insights into the indexing process.
Monitoring with Elasticsearch REST Client
Using the Elasticsearch REST client, we can retrieve valuable metrics related to indexing performance, such as indexing throughput, latency, and error rates.
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http")));
GetRequest getRequest = new GetRequest(".monitoring-es-7-2022.03.01-000001");
getRequest.fetchSourceContext(new FetchSourceContext(false));
getRequest.storedFields("_none_");
GetResponse getResponse = client.get(getRequest, RequestOptions.DEFAULT);
By querying the appropriate monitoring indices and analyzing the obtained metrics, we can identify performance bottleneks and inefficiencies in the indexing process.
My Closing Thoughts on the Matter
In conclusion, optimizing indexing performance in Elasticsearch is crucial for efficiently handling large volumes of data. By leveraging the Elasticsearch Java API, tuning index settings, and employing effective monitoring techniques, developers can significantly improve indexing throughput and overall system performance. Implementing batch processing, asynchronous operations, and fine-tuning index settings are key strategies for achieving optimal indexing performance. Constant monitoring and profiling play a vital role in identifying bottlenecks and ensuring the continued optimization of the indexing process.
In the world of Elasticsearch and Java, indexing performance optimization is a critical aspect of building efficient and scalable applications, and mastering these techniques is essential for developers and organizations aiming to make the most of their Elasticsearch deployments.
By incorporating these best practices and harnessing the power of Java, developers can unlock the full potential of Elasticsearch to manage and query large datasets with exceptional performance.
Remember, indexing performance tuning is an ongoing process that requires continuous monitoring, optimization, and adaptation to the evolving needs of the application and underlying data infrastructure.
Start optimizing your Elasticsearch indexing performance today and unleash the full power of your data!
Checkout our other articles