Reliability Issues with Guaranteed Messaging in JMS
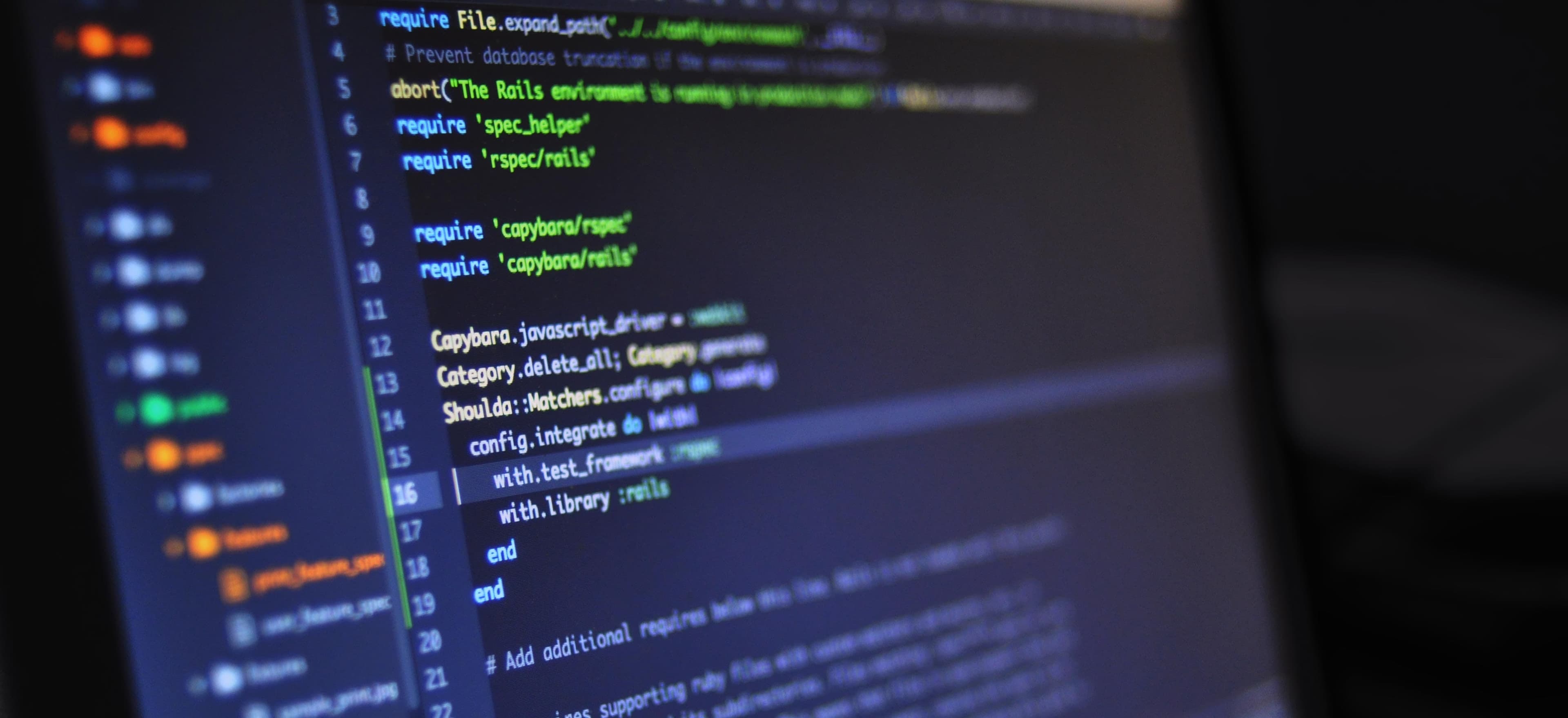
- Published on
Reliability Issues with Guaranteed Messaging in JMS
Java Message Service (JMS) is a widely used messaging standard that allows Java applications to create, send, receive, and read messages. JMS provides two messaging models: Point-to-Point (P2P) and Publish/Subscribe. While JMS offers a powerful and versatile messaging solution, there can be some reliability issues with guaranteed messaging. In this blog post, we will delve into the challenges and potential solutions associated with guaranteed messaging in JMS.
Understanding Guaranteed Messaging
Guaranteed messaging ensures that a message sent by a producer will be reliably delivered to the intended consumer, even in the presence of system failures. This is achieved through mechanisms such as persistent messaging, acknowledgments, and redelivery.
In JMS, the concept of guaranteed messaging is primarily implemented through the use of queues. When a message is sent to a queue, it remains in the queue until it is consumed by a client. This ensures that the message will not be lost, even if the consumer is not immediately available.
Reliability Challenges
Despite the inherent guarantees provided by JMS, there are several challenges that can affect the reliability of guaranteed messaging.
1. Message Persistence
One of the key challenges is ensuring message persistence. While JMS allows messages to be marked as persistent, there is no guarantee that a message will be safely stored in the event of a system failure. This can lead to message loss if the broker crashes before messages are persistently stored.
2. Message Acknowledgment
In JMS, message acknowledgment is a critical aspect of guaranteed messaging. However, there are scenarios where a consumer may fail to acknowledge the message due to various reasons such as network issues, application errors, or crashes. This can result in message redelivery, leading to potential duplicates and out-of-order message processing.
3. Handling Redelivery
Handling message redelivery is another challenge in JMS. If a message is not acknowledged within a specified time frame, it may be redelivered to the consumer. While redelivery is essential for ensuring message reliability, it can introduce complexities in message processing logic and potentially impact system performance.
Strategies for Improving Reliability
To address the reliability issues associated with guaranteed messaging in JMS, consider the following strategies:
1. Message Persistence Configuration
When sending messages to a queue, ensure that the message persistence configuration is appropriately set. This includes configuring the JMS broker to persist messages to durable storage and setting appropriate replication and backup mechanisms to prevent message loss in the event of a system failure.
Example of configuring message persistence in JMS:
// Set delivery mode to PERSISTENT
MessageProducer producer = session.createProducer(queue);
producer.setDeliveryMode(DeliveryMode.PERSISTENT);
In the above code snippet, setting the delivery mode to PERSISTENT
ensures that messages are persistently stored by the JMS broker.
2. Handling Message Acknowledgment
Implement robust message acknowledgment logic to handle scenarios where consumers fail to acknowledge messages. Consider using JMS transactions to ensure that message acknowledgment and processing occur atomically, preventing message loss and duplicate processing.
Example of handling message acknowledgment with JMS transactions:
// Start a JMS transaction
session = connection.createSession(true, Session.SESSION_TRANSACTED);
Message message = consumer.receive();
// Process the message
// Commit the JMS transaction
session.commit();
By using JMS transactions, message acknowledgment and processing can be coordinated within a transaction boundary, ensuring message reliability.
3. Redelivery Policies
Define and implement redelivery policies to govern the behavior of message redelivery in JMS. Configure redelivery limits, delay intervals, and backoff strategies to control the redelivery behavior and prevent excessive redelivery attempts that could affect system performance.
Example of configuring redelivery policies in JMS:
// Set the maximum redelivery count
RedeliveryPolicy redeliveryPolicy = new RedeliveryPolicy();
redeliveryPolicy.setMaximumRedeliveries(5);
In the above code snippet, a redelivery policy is defined with a maximum redelivery count of 5, ensuring that messages are not indefinitely redelivered in case of consumer failures.
Lessons Learned
Guaranteed messaging in JMS provides a robust mechanism for ensuring message reliability, but it comes with its own set of challenges. By understanding the reliability issues and implementing appropriate strategies, such as configuring message persistence, handling message acknowledgment, and defining redelivery policies, it is possible to improve the reliability of guaranteed messaging in JMS.
As with any messaging system, it is essential to carefully design and test the reliability mechanisms to ensure consistent and predictable behavior in production environments. By addressing these reliability issues, JMS can continue to serve as a dependable and resilient messaging solution for Java applications.
In conclusion, while guaranteed messaging in JMS may present challenges, with careful configuration and thoughtful implementation, these challenges can be addressed to ensure reliable message delivery in Java applications.