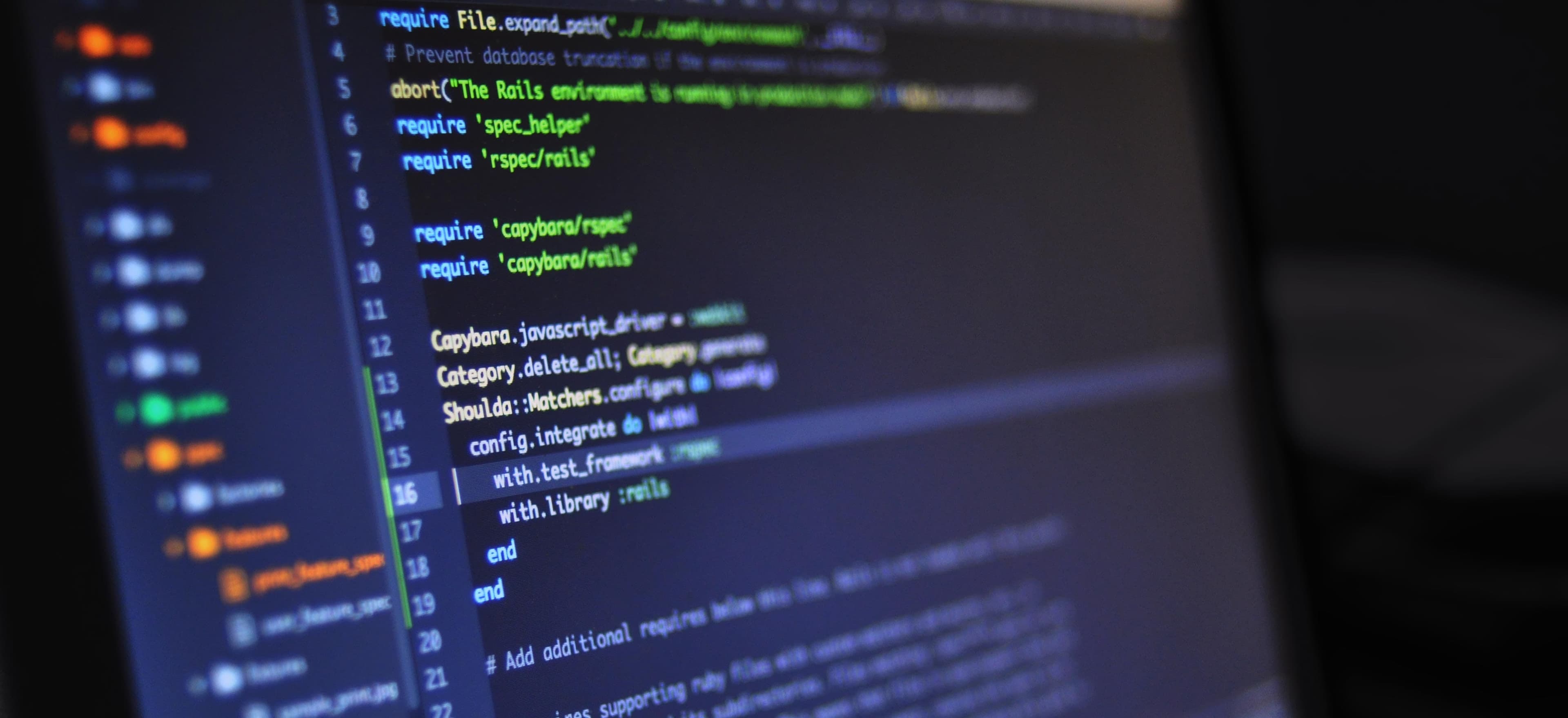
- Published on
When it comes to creating stunning GUIs in Java, one feature that can greatly enhance the visual appeal is a transparent JFrame. A transparent JFrame allows you to see through the window, adding a touch of modernity and elegance to your application. In this tutorial, we will explore how to create a transparent JFrame in Java, discussing both the technical implementation and the design considerations.
Why Use a Transparent JFrame?
Before we dive into the technical details, let's briefly discuss the reasons why you might want to use a transparent JFrame in your Java application.
-
Visual Appeal: A transparent JFrame can give your application a sleek and modern look, especially when used in conjunction with other design elements such as animations or background effects.
-
Enhanced User Experience: By incorporating transparency, you can create layered interfaces that provide users with additional context and visual cues, enhancing the overall user experience.
Now that we understand the benefits, let's move on to the implementation.
Setting up the Project
To begin, make sure you have the latest version of Java installed on your system. We will be using Java Swing for our GUI components. If you haven't already, install an integrated development environment (IDE) such as IntelliJ IDEA or Eclipse.
Once your development environment is set up, create a new Java project and add a new JFrame to the project. For this example, we will name the JFrame class TransparentFrame
.
Creating a Transparent JFrame
In order to make our JFrame transparent, we need to make use of the setOpacity
method introduced in Java 1.7. This method allows us to set the opacity of the JFrame, making it semi-transparent. Let's look at the code snippet below to see how this is achieved:
import javax.swing.*;
public class TransparentFrame extends JFrame {
public TransparentFrame() {
setBounds(100, 100, 450, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setOpacity(0.8f); // Set the opacity to 80%
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
TransparentFrame frame = new TransparentFrame();
frame.setVisible(true);
});
}
}
In this example, we create a new class TransparentFrame
which extends JFrame
. In the constructor, we use the setBounds
method to set the initial position and size of the frame. We then set the default close operation and, most importantly, use the setOpacity
method to make the frame partially transparent. The value passed to setOpacity
is a float ranging from 0.0f (completely transparent) to 1.0f (completely opaque).
Design Considerations
While using transparency can greatly enhance your GUI, it's important to consider the usability and readability of your application. Here are some design considerations to keep in mind:
-
Contrast: Ensure that any text or important elements remain easily readable against the background. You may need to adjust the transparency level to achieve sufficient contrast.
-
Consistency: Maintain a consistent design language throughout your application. If certain components are transparent, ensure that the transparency is used purposefully and consistently.
-
Performance: Be mindful of the performance implications of using transparency, especially if your application contains complex graphics or runs on less powerful hardware.
Key Takeaways
In this tutorial, we explored the process of creating a transparent JFrame in Java. We discussed the benefits of using transparency in GUI design, provided a code example, and highlighted important design considerations. By incorporating a transparent JFrame into your Java application, you can elevate the visual appeal and user experience of your software.
So go ahead, experiment with transparency in your Java GUIs, and watch your applications take on a whole new level of sophistication!