Mastering Mocks and Stubs in Testing
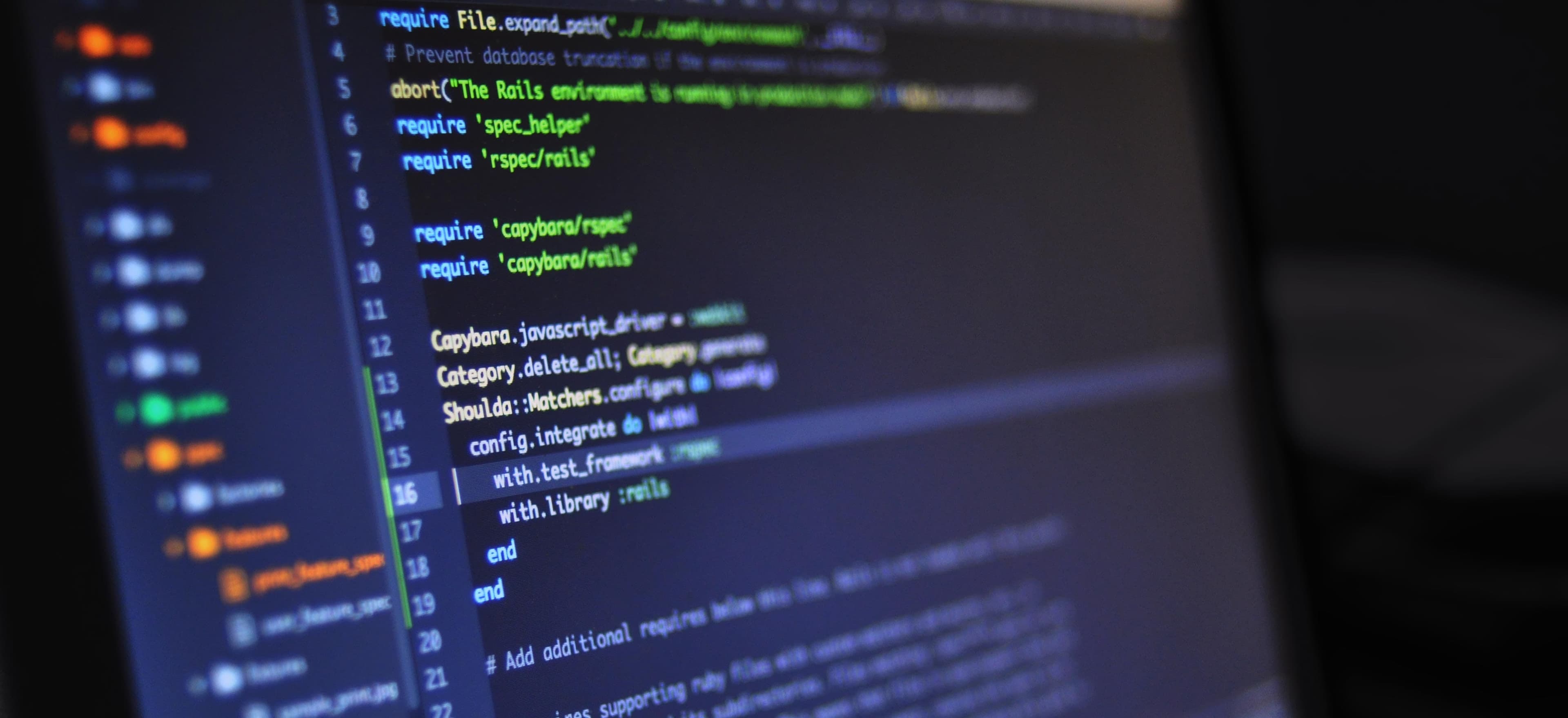
- Published on
Mastering Mocks and Stubs in Testing
When it comes to Java development, unit testing plays a crucial role in ensuring the quality and reliability of the code. As part of the unit testing process, developers often come across the concepts of mocks and stubs. In this article, we will delve into the intricacies of mocks and stubs, understand their significance in testing, and learn how to effectively use them in Java applications.
Understanding Mocks and Stubs
Mocks
Mocks, in the context of testing, are simulated objects that mimic the behavior of real objects. They are primarily used to isolate the code under test and verify interactions with dependencies. Mock objects are programmed to return specific outputs and capture specific inputs, allowing developers to verify that the expected interactions with dependencies are taking place.
Stubs
Stubs, on the other hand, are minimal implementations of interfaces or classes, providing canned responses to method calls. They are used to simulate the behavior of components that the code under test depends on. By using stubs, developers can control the indirect inputs of the code under test and focus solely on testing its functionality.
Why Use Mocks and Stubs?
Isolation and Independence
Mocks and stubs facilitate the isolation of the code under test by decoupling it from its dependencies. This isolation ensures that the behavior being tested is solely due to the code itself and not influenced by the external components.
Faster and More Reliable Testing
By using mocks and stubs, developers can create controlled environments for testing, leading to faster and more reliable tests. With dependencies replaced by mocks or stubs, tests become less reliant on the intricacies of external systems, resulting in more predictable and consistent outcomes.
Simulating Unreachable Scenarios
Mocks and stubs enable developers to simulate scenarios that would otherwise be hard to reproduce, such as network errors, database failures, or other exceptional conditions. This allows for comprehensive testing of error-handling and edge cases.
Implementing Mocks and Stubs in Java
Now that we understand the significance of mocks and stubs, let’s explore how to implement them in Java using popular testing frameworks such as JUnit and Mockito.
Using Mockito for Mocking
Mockito is a widely used mocking framework for Java. Let’s consider a simple example where we want to test a PaymentService
class that depends on a PaymentGateway
interface. We can use Mockito to create a mock instance of the PaymentGateway
interface and define its behavior within our test.
import static org.mockito.Mockito.*;
public class PaymentServiceTest {
@Test
public void testPaymentProcess() {
PaymentGateway mockedGateway = mock(PaymentGateway.class);
when(mockedGateway.processPayment(anyDouble())).thenReturn(true);
PaymentService paymentService = new PaymentService(mockedGateway);
boolean result = paymentService.processPayment(100.00);
assertTrue(result);
verify(mockedGateway, times(1)).processPayment(100.00);
}
}
In this example, we create a mock instance of the PaymentGateway
interface using mock()
. We then define the behavior of the processPayment()
method using when().thenReturn()
to return true
for any input. We proceed to test the PaymentService
class by passing the mock instance of PaymentGateway
and verifying the expected interactions using verify()
.
Using Stubs with JUnit
When it comes to using stubs in Java, JUnit provides a simple and effective way to create stub objects. Let’s consider a scenario where we want to test a Calculator
class that utilizes a MathService
interface for performing mathematical operations. We can create a stub implementation of the MathService
interface using a simple inline class definition within our test.
public class CalculatorTest {
@Test
public void testAddition() {
MathService mathServiceStub = new MathService() {
@Override
public int add(int a, int b) {
return a + b;
}
};
Calculator calculator = new Calculator(mathServiceStub);
int result = calculator.add(3, 5);
assertEquals(8, result);
}
}
In this example, we create a stub implementation of the MathService
interface inline, providing a simple addition operation. We then test the Calculator
class by passing this stub implementation and verifying the addition functionality.
Best Practices for Using Mocks and Stubs
Clear and Focused Tests
When using mocks and stubs, it’s important to keep the tests clear and focused on the behavior of the code under test. Avoid overly complex setups or assertions in the tests, aiming for simplicity and readability.
Balance Realism and Simplicity
While using mocks and stubs, strike a balance between creating realistic simulations of dependencies and keeping the tests simple. Overcomplicating the mocks or stubs can lead to brittle tests, while oversimplifying may result in inaccurate representations of dependencies.
Regular Maintenance and Review
As the codebase evolves, it’s crucial to regularly review and update the mocks and stubs used in tests. Changes in the dependencies or their behavior should be reflected in the corresponding mocks and stubs to ensure the tests remain relevant and reliable.
My Closing Thoughts on the Matter
Mastering mocks and stubs in testing is a valuable skill for Java developers. By effectively using mocks and stubs, developers can create thorough, reliable, and maintainable tests for their code. Understanding the significance of isolation, speed, and simulation, along with best practices, empowers developers to harness the full potential of mocks and stubs in their testing endeavors.
In conclusion, incorporating mocks and stubs into unit testing not only enhances the overall quality of the codebase but also instills confidence in the robustness of the application.
To broaden your understanding of testing in Java, consider exploring additional topics such as integration testing and test-driven development. Happy testing!