Implementing Strategy Pattern in Chess Game
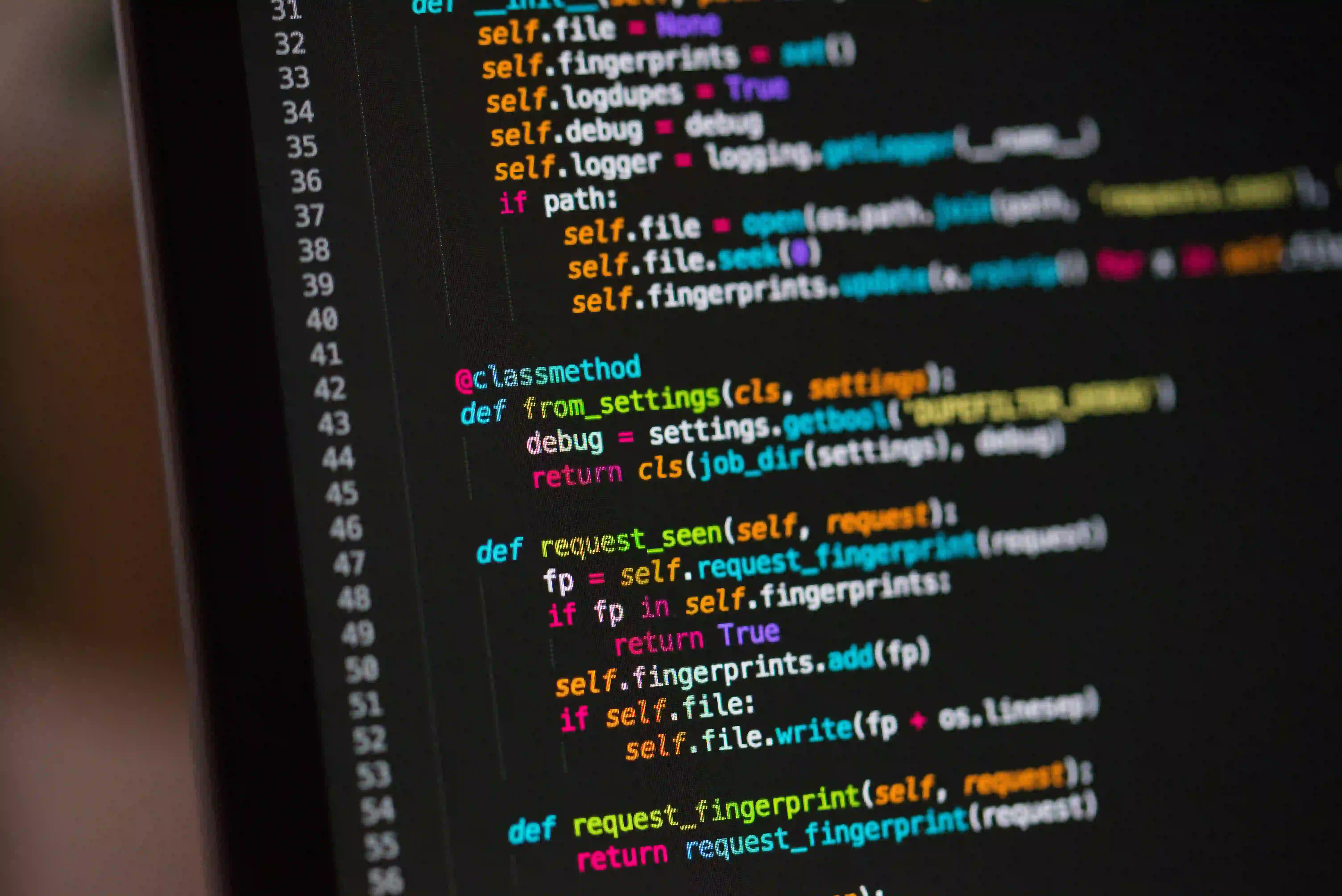
Implementing Strategy Pattern in Chess Game
In this post, we will explore the implementation of the Strategy Pattern in a Java chess game. The Strategy Pattern is a behavioral design pattern that enables defining a family of algorithms, encapsulating each one, and making them interchangeable. This pattern allows the algorithm to vary independently from clients that use it.
Understanding the Strategy Pattern
The Strategy Pattern involves defining a family of algorithms, encapsulating each one, and making them interchangeable. It allows the algorithm to vary independently from clients that use it.
In the context of a chess game, the application of the Strategy Pattern can be seen in the various movements that different pieces can make. Each piece (e.g., pawn, knight, bishop) can have its own unique movement strategy, which can be defined in separate classes implementing a common interface.
Applying the Strategy Pattern in Chess
Let's consider the implementation of the Strategy Pattern for the movements of chess pieces.
Defining the Strategy Interface
We begin by defining a common interface, PieceMovementStrategy
, that declares a method for moving the chess piece.
public interface PieceMovementStrategy {
void move();
}
Implementing Concrete Strategies
Next, we create concrete strategy classes for each type of chess piece. For instance, let's consider the movement strategy for a pawn:
public class PawnMovementStrategy implements PieceMovementStrategy {
@Override
public void move() {
// Implement pawn movement logic
}
}
Similarly, we can create strategy classes for other chess pieces such as knight, rook, bishop, queen, and king.
Context - Chess Piece
We then define the context, which in this case is the chess piece. This context class should have a reference to the strategy interface and a method to execute the movement strategy.
public class ChessPiece {
private PieceMovementStrategy movementStrategy;
public void setMovementStrategy(PieceMovementStrategy movementStrategy) {
this.movementStrategy = movementStrategy;
}
public void movePiece() {
movementStrategy.move();
}
}
Utilizing the Strategy Pattern
With the setup in place, we can utilize the Strategy Pattern in our chess game. When a player selects a piece to move, we can set the appropriate movement strategy for that piece and execute its movement using the movePiece
method.
ChessPiece pawn = new ChessPiece();
pawn.setMovementStrategy(new PawnMovementStrategy());
pawn.movePiece();
In this way, the Strategy Pattern allows the movement behavior of chess pieces to vary independently, and new movement strategies can be added without altering the existing code.
Advantages of Using the Strategy Pattern in Chess Game
Encapsulating Behavior
The Strategy Pattern encapsulates the behavior of each chess piece into its own strategy class, promoting a clean and organized code structure.
Flexibility and Extensibility
The Strategy Pattern allows for the easy addition of new movement strategies for chess pieces without affecting the existing code, making the system more flexible and extensible.
Improved Testability
By isolating the movement strategies into separate classes, unit testing and maintenance become more straightforward, leading to improved code quality.
My Closing Thoughts on the Matter
In conclusion, the Strategy Pattern provides an elegant solution for implementing different movement strategies in a Java chess game. By decoupling the movement behavior from the chess pieces, it enables better maintainability, flexibility, and extensibility of the codebase. Embracing design patterns like the Strategy Pattern can greatly enhance the quality and scalability of software systems.
Implementing the Strategy Pattern in a chess game not only demonstrates the practical application of this design pattern but also showcases its effectiveness in managing complex and varying behaviors within a system.
To delve deeper into design patterns, and to explore their applications in various software development scenarios, check out Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, commonly referred to as the "Gang of Four."
Incorporating the Strategy Pattern into your codebase can greatly enhance its maintainability, flexibility, and extensibility, making it a valuable addition to your design pattern arsenal.