Unavoidable Consequences of Ignoring Technical Debt
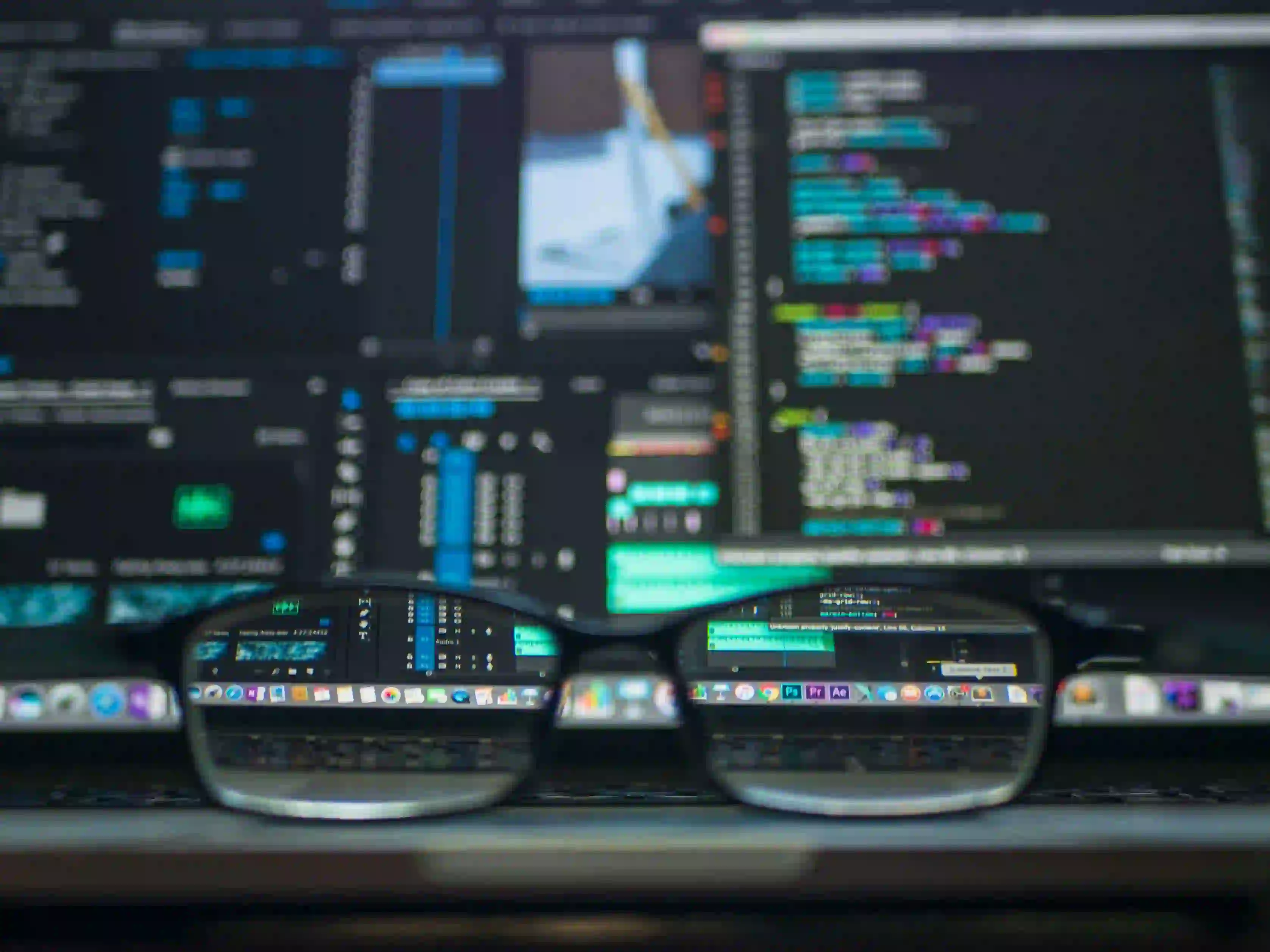
The Unavoidable Consequences of Ignoring Technical Debt
In the fast-paced world of software development, it's easy to succumb to the pressure of delivering new features quickly, often at the expense of code quality. However, taking shortcuts and accumulating technical debt can have severe consequences that can't be ignored.
What is Technical Debt?
Technical debt refers to the accumulated cost of additional rework created by choosing an easy, expedient solution now instead of using a better approach that would take longer. It's akin to financial debt – you can incur it to achieve certain goals more quickly, but you will have to repay it eventually, often with interest.
The Unavoidable Consequences
1. Reduced Productivity
Ignoring technical debt increases the complexity of the codebase, making it harder and more time-consuming to implement new features. Developers get bogged down by having to work around existing issues or refactor poorly written code, leading to decreased productivity.
2. Bug Proliferation
Technical debt tends to manifest itself in the form of bugs. As workarounds and quick fixes pile up, the code becomes more fragile and error-prone. This can result in an endless cycle of identifying and fixing new bugs without addressing the underlying issues.
3. Diminished Code Quality
Accrued technical debt often leads to diminished code quality. As teams hurry to meet deadlines and deliver new features, the focus shifts from writing clean, maintainable code to just getting the job done. This can create a snowball effect, as poor code begets more poor code.
4. Increased Maintenance Costs
Just like financial debt, technical debt accrues interest. The longer code remains in a suboptimal state, the more effort it takes to maintain and enhance it. This eventually results in higher maintenance costs, consuming resources that could have been invested in driving the product forward.
5. Impeded Innovation
In a codebase riddled with technical debt, it becomes increasingly difficult to innovate. The majority of development efforts are spent on managing the existing mess rather than exploring new ideas and implementing cutting-edge features.
6. Eroded Customer Satisfaction
Ultimately, the consequences of technical debt culminate in a diminished user experience. The system becomes less stable, suffers from performance issues, and offers a generally underwhelming experience to the end-user, leading to reduced customer satisfaction and potentially lost business.
Mitigating Technical Debt
While it may seem daunting, there are strategies to mitigate and manage technical debt effectively.
1. Regular Refactoring
Schedule time for regular refactoring sessions to address technical debt. This involves restructuring existing code without changing its external behavior, enhancing its maintainability, and reducing complexity. By doing so incrementally, the daunting task becomes more manageable.
Code Example:
// Example of refactoring to extract a method
// Before refactoring
void complexMethod() {
// ... complex logic
// ... more complex logic
}
// After refactoring
void complexMethod() {
extractMethodForComplexLogicPart1();
extractMethodForComplexLogicPart2();
}
void extractMethodForComplexLogicPart1() {
// ... complex logic
}
void extractMethodForComplexLogicPart2() {
// ... more complex logic
}
In this example, the initial complex method is refactored into smaller, more manageable methods, making the code easier to read and maintain.
2. Automated Testing
Invest in comprehensive automated testing to catch regressions and bugs early. This not only ensures the stability of the codebase but also provides a safety net for making changes or refactoring without introducing new issues.
Code Example:
// Example of a unit test using JUnit
@Test
void testAddition() {
Calculator calculator = new Calculator();
assertEquals(4, calculator.add(2, 2));
}
In this example, a unit test for the add
method of a Calculator
class ensures that the method behaves as expected.
3. Continuous Integration and Delivery (CI/CD)
Implementing CI/CD pipelines enforces frequent integration of code changes and automated deployment. This reduces the risk of integration problems and ensures that the codebase is always in a deployable state, making it easier to address technical debt incrementally.
Code Example:
# Example of a CI/CD pipeline using Jenkins declarative syntax
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build the project
}
}
stage('Test') {
steps {
// Run automated tests
}
}
stage('Deploy') {
steps {
// Deploy the project
}
}
}
}
This example depicts a basic CI/CD pipeline that builds, tests, and deploys the project automatically upon code changes.
4. Educating and Sharing Knowledge
Foster a culture of code reviews, knowledge sharing, and mentoring among developers. This not only helps in identifying and addressing technical debt early but also spreads awareness about best practices, leading to better code quality overall.
Code Example:
// Example of code review feedback
// Reviewer: Consider using a more descriptive variable name here for clarity.
// Original: int x;
// Suggested: int numberOfUsers;
In this example, the code reviewer suggests using a more descriptive variable name for improved clarity, contributing to better code quality.
5. Prioritizing Technical Debt
Make addressing technical debt a priority in the development process. This involves weighing the trade-offs of addressing technical debt versus implementing new features and striking a balance to ensure the long-term sustainability of the codebase.
Code Example:
// Example of prioritizing technical debt
// TODO: Refactor the legacy authentication module to use modern cryptographic algorithms
// Implementing this task ensures enhanced security and mitigates technical debt associated with outdated encryption methods.
In this example, a code comment clearly outlines the technical debt to be addressed and its impact on security, emphasizing its importance.
Bringing It All Together
Ignoring technical debt can lead to a myriad of unavoidable consequences that impede productivity, diminish code quality, increase maintenance costs, hinder innovation, and erode customer satisfaction. However, by implementing strategies such as regular refactoring, automated testing, CI/CD, knowledge sharing, and prioritization, it's possible to mitigate and manage technical debt effectively, ensuring the long-term health and sustainability of the codebase.
Addressing technical debt is not just a technical decision; it's a strategic investment in the future of the product and the satisfaction of its users.
By acknowledging the inevitable consequences of technical debt and actively working to mitigate it, software development teams can pave the way for a more robust, resilient, and innovative future.
Incorporating these strategies into the development process fosters a culture of continuous improvement and long-term success. It's essential to act today to avoid the unavoidable consequences of tomorrow.