Optimizing RESTful Architecture for Scalability
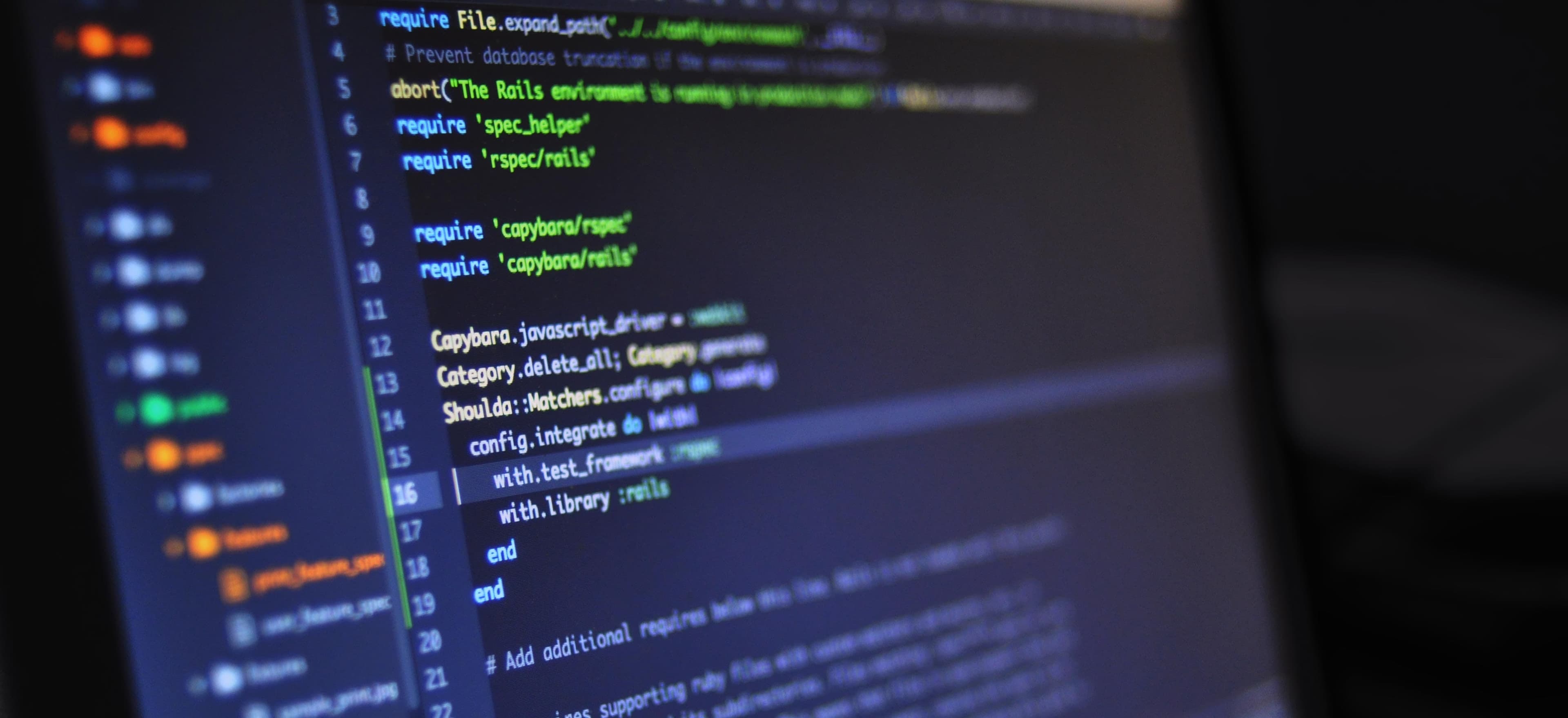
- Published on
Optimizing RESTful Architecture for Scalability
In today's digital era, with the exponential growth of users and data, building scalable and high-performing RESTful architecture is crucial for the success of any modern application. This blog post will delve into the strategies and best practices for optimizing RESTful architecture to ensure scalability.
Understanding RESTful Architecture
Representational State Transfer (REST) is an architectural style for designing networked applications. RESTful architecture relies on a stateless, client-server communication model, where each request from the client to the server must contain all the information necessary to understand and fulfill the request. This architectural style is built upon a set of constraints, including uniform interface, statelessness, cacheability, layered system, code on demand, and client-server architecture.
Importance of Scalability in RESTful Architecture
Scalability is the ability of a system to handle growing amounts of work or its potential to be enlarged to accommodate that growth. In the context of RESTful architecture, scalability is crucial for ensuring that as traffic and data volume increase, the system can handle the load without sacrificing performance, responsiveness, or reliability.
Optimizing RESTful Architecture for Scalability
1. Embracing Asynchronous Processing
Traditional (Synchronous) Processing:
public String getUserDetails(String userId) {
UserDetails userDetails = userRepository.getUserDetails(userId);
// Additional processing
return userDetails;
}
Asynchronous Processing with CompletableFuture (Java 8+):
public CompletableFuture<UserDetails> getUserDetails(String userId) {
return CompletableFuture.supplyAsync(() -> userRepository.getUserDetails(userId))
.thenApplyAsync(userDetails -> {
// Additional processing
return userDetails;
});
}
Why Asynchronous Processing?
Asynchronous processing allows the system to handle a larger number of concurrent requests without blocking threads. This is particularly essential in RESTful services where response times greatly impact scalability and user experience.
2. Caching to Reduce Server Load
Caching involves storing server responses in a cache and reusing those responses to satisfy future requests. By leveraging tools such as Redis or Memcached, RESTful services can cache frequently accessed data and reduce the load on the server.
Example of Response Caching using Spring Boot:
@Cacheable("userDetails")
public UserDetails getUserDetails(String userId) {
// Logic to fetch user details from the database
}
Why Caching?
Caching minimizes the need to repeatedly perform expensive operations, such as accessing a database or making external API calls, thus enhancing the overall performance and scalability of the system.
3. Horizontal Scaling with Microservices
Dividing a monolithic architecture into microservices allows for horizontal scaling, where each microservice can be independently scaled based on its specific resource demands.
Example of Microservice Communication using Spring Cloud Netflix and Eureka:
// Service Registration
@EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
// Microservice Consumer
@EnableEurekaClient
@SpringBootApplication
public class UserConsumerServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserConsumerServiceApplication.class, args);
}
}
// Microservice Provider
@EnableEurekaClient
@SpringBootApplication
public class UserDetailsProviderServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserDetailsProviderServiceApplication.class, args);
}
}
Why Horizontal Scaling with Microservices?
By breaking down the system into smaller, independently deployable microservices, it becomes feasible to scale specific services that are experiencing heavier loads, thus enhancing overall system scalability.
4. Leveraging Content Delivery Networks (CDNs)
Integrating a Content Delivery Network (CDN) can significantly enhance the scalability of a RESTful architecture by caching static content and distributing it closer to the end users, thereby reducing server load and minimizing latency.
Example of CDN Integration with Amazon CloudFront:
// Amazon S3 Bucket Configuration
// Content is stored in an S3 bucket and distributed through CloudFront
Why CDN Integration?
CDNs reduce the load on the origin server by caching and serving content from edge locations, leading to improved scalability and a better user experience, especially for geographically distributed user bases.
5. Load Balancing for Even Distribution
Load balancers distribute network or application traffic across multiple servers, ensuring no single server becomes overwhelmed with traffic while others remain underutilized.
Example of Load Balancing with Nginx:
upstream backend {
server backend1.example.com;
server backend2.example.com;
// Additional server configurations
}
server {
location / {
proxy_pass http://backend;
}
}
Why Load Balancing?
Load balancing optimizes resource utilization, maximizes throughput, minimizes response time, and avoids overloading any single resource, thereby promoting scalability and fault tolerance in the system.
Closing Remarks
In conclusion, optimizing RESTful architecture for scalability is essential for meeting the growing demands of digital applications. By embracing asynchronous processing, leveraging caching, adopting microservices, integrating CDNs, and implementing load balancing, developers can ensure that their RESTful architecture can handle increasing loads without sacrificing performance or reliability.
By following these best practices and strategies, developers can create highly scalable and responsive RESTful architectures that are well-equipped to meet the challenges of modern, data-intensive applications.
Optimizing RESTful architecture for scalability is an ongoing process, and staying updated with the latest tools, techniques, and best practices is crucial to staying ahead in the competitive landscape of modern software development.
For more in-depth discussions on RESTful architecture and scalability, check out the RESTful API Design: Best Practices and Scalability Strategies for Modern Applications articles.