Understanding Hibernate NonStrictReadWrite CacheConcurrencyStrategy
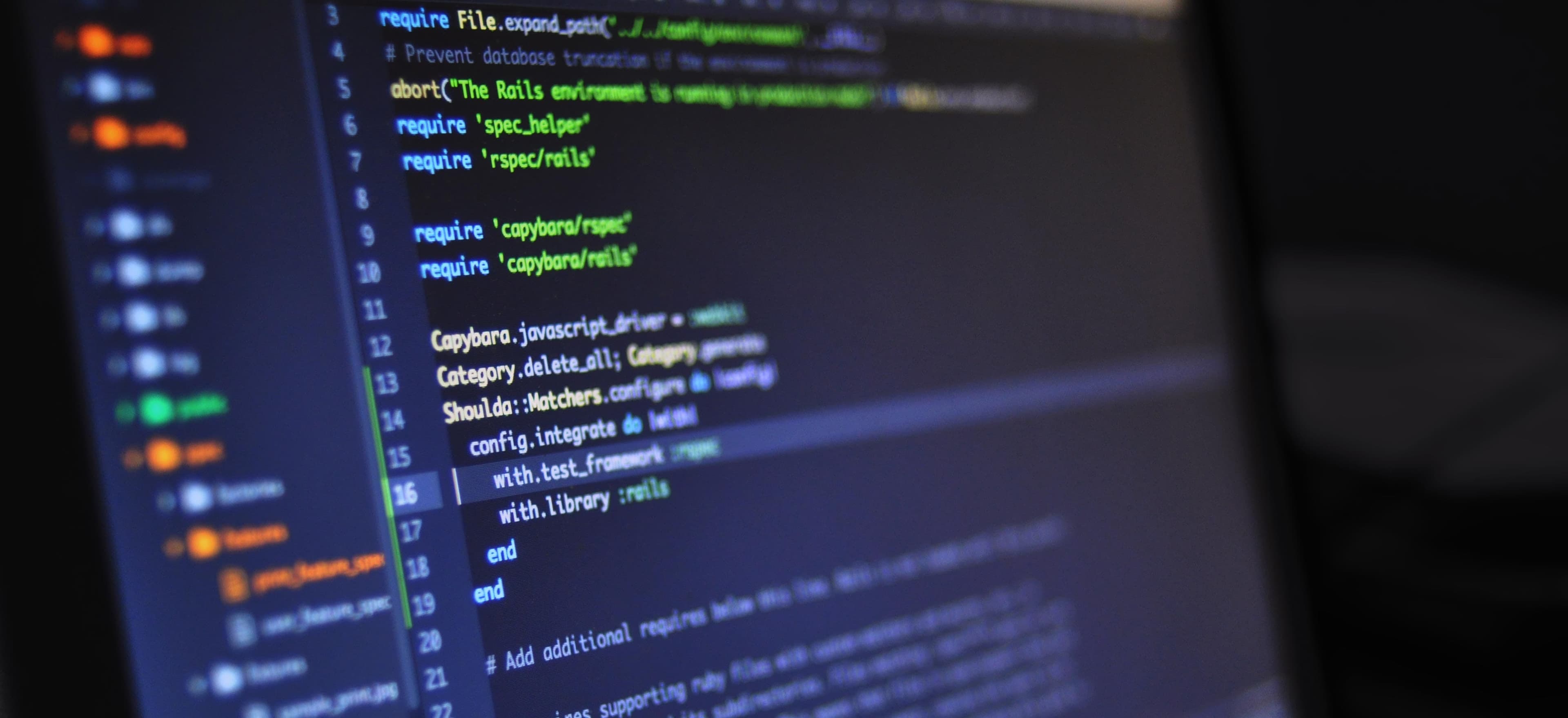
- Published on
Understanding Hibernate NonStrictReadWrite CacheConcurrencyStrategy
When it comes to optimizing the performance of a Java application, caching plays a critical role. Hibernate, the widely-used object-relational mapping (ORM) framework for Java, provides various caching strategies to improve application performance. One such strategy is the NonStrictReadWrite
CacheConcurrencyStrategy
, which we'll explore in this blog post.
What is CacheConcurrencyStrategy?
In Hibernate, the CacheConcurrencyStrategy
defines how cache concurrency is managed for entities and collections. It determines how the caching system interacts with the underlying database to ensure data consistency and performance.
NonStrictReadWrite
The NonStrictReadWrite
strategy is one of the cache concurrency strategies provided by Hibernate. It is aimed at improving read performance while sacrificing strict consistency. When using this strategy, the cache is updated asynchronously, allowing for better read performance at the expense of potential stale data.
Code Example:
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
@Entity
public class Product {
// Entity mapping
}
In the code snippet above, we annotate the Product
entity with the NONSTRICT_READ_WRITE
cache concurrency strategy. This tells Hibernate to use the NonStrictReadWrite
strategy for caching instances of the Product
entity.
Why Use NonStrictReadWrite?
- Improved Read Performance: By allowing stale data in the cache, the
NonStrictReadWrite
strategy can significantly improve read performance, especially in read-heavy applications. - Reduced Database Load: With the
NonStrictReadWrite
strategy, frequently accessed data can be served from the cache, reducing the load on the database server. - Asynchronous Updates: As updates to the cache are performed asynchronously, write operations on the database can proceed without waiting for the cache to be updated, enhancing overall system responsiveness.
When to Use NonStrictReadWrite?
While the NonStrictReadWrite
strategy offers improved read performance, it may not be suitable for all use cases. Consider using this strategy in the following scenarios:
- Read-Heavy Workloads: Applications that prioritize read operations over strict data consistency can benefit from the
NonStrictReadWrite
strategy. - Data with Low Update Frequency: Entities or collections that are updated infrequently are good candidates for this caching strategy.
Lessons Learned
In summary, the NonStrictReadWrite
CacheConcurrencyStrategy
provided by Hibernate offers improved read performance and reduced database load at the expense of strict data consistency. By understanding its benefits and trade-offs, developers can make informed decisions when optimizing the caching strategy for their Hibernate-powered applications.
To delve deeper into Hibernate caching strategies, you can explore the official Hibernate documentation on Caching.
Stay tuned for more insightful posts on Java, Hibernate, and software performance optimization!