Practical Tips for Applying SOLID Principles
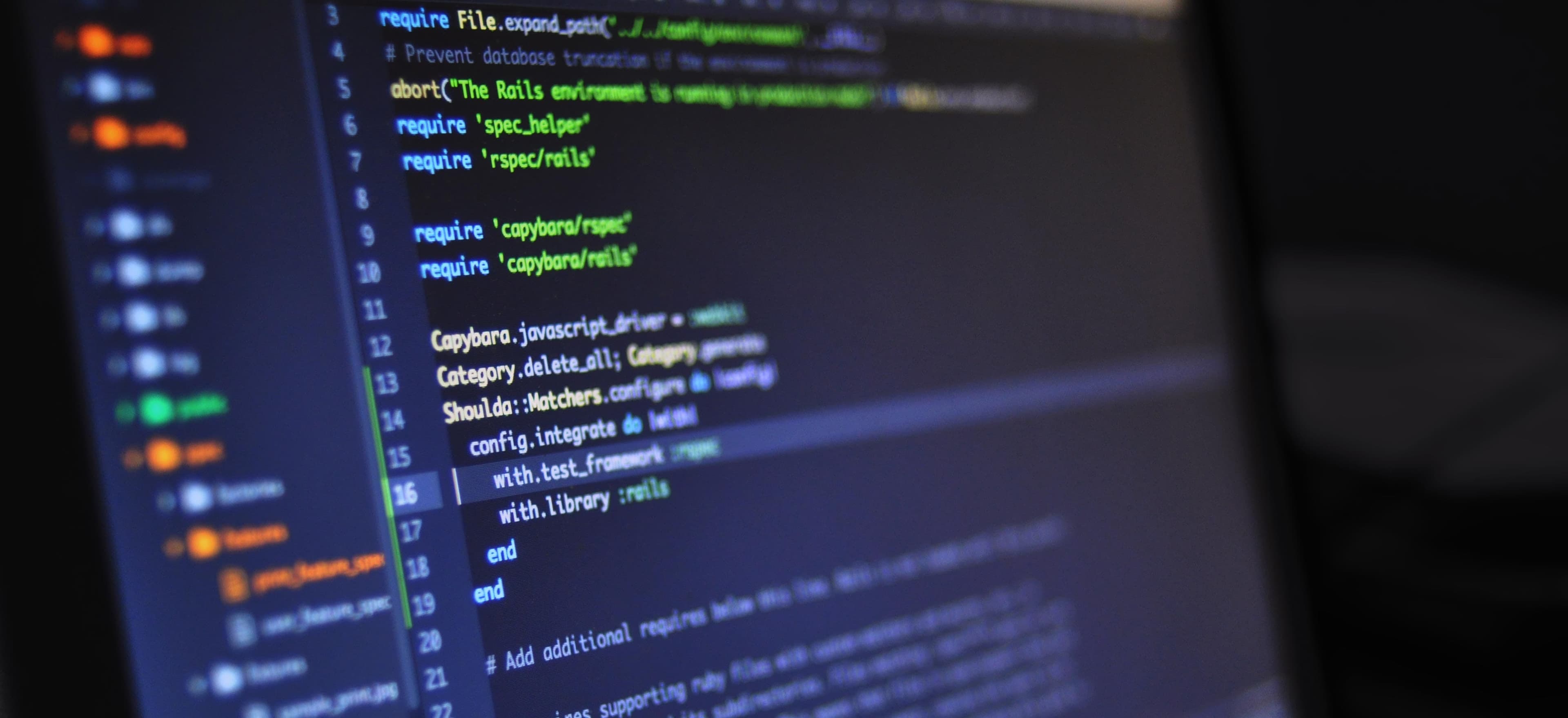
- Published on
Practical Tips for Applying SOLID Principles in Java Development
In the world of software development, SOLID principles are a crucial set of guidelines for writing maintainable, scalable, and flexible code. These principles, introduced by Robert C. Martin, aim to improve the design, readability, and maintainability of object-oriented code.
What are the SOLID Principles?
SOLID is an acronym that stands for five principles namely:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
In this blog, we will discuss practical tips and techniques for applying these principles in Java development.
Single Responsibility Principle (SRP)
The SRP states that a class should have only one reason to change. In other words, a class should have only one job. To adhere to SRP:
- Identify the responsibilities of your class and ensure that each class focuses on fulfilling a single responsibility.
- If a class grows to handle multiple responsibilities, consider refactoring it into smaller, more focused classes.
- Apply the "High Cohesion" principle where the methods and fields within a class are related and contribute to a single, well-defined purpose.
// Example of Violation of SRP
public class Employee {
public void calculatePay() {
// calculate pay logic
}
public void saveEmployee() {
// save employee logic
}
}
In the above code, the Employee
class is responsible for both calculating pay and saving employee details. This violates the SRP, and it would be better split into PayCalculator
and EmployeeRepository
classes.
Open/Closed Principle (OCP)
The OCP states that a class should be open for extension but closed for modification. To follow OCP:
- Use abstraction and inheritance to allow adding new functionality to a class without altering its source code.
- Embrace design patterns like Strategy and Template Method to facilitate extension without modification.
- Seal off parts of the code that should not be extended or modified unnecessarily.
// Example of OCP
public interface Shape {
double area();
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
public class Square implements Shape {
private double side;
public Square(double side) {
this.side = side;
}
@Override
public double area() {
return side * side;
}
}
In this example, the Shape
interface is open for extension, and the Circle
and Square
classes are closed for modification, allowing for the addition of new shapes without altering existing code.
Liskov Substitution Principle (LSP)
The LSP states that objects of a superclass should be replaceable with objects of its subclass without affecting the correctness of the program. To ensure LSP compliance:
- Subclass implementations should maintain the contracts of their base classes and not change the expected behavior.
- Overriding methods should enhance functionality without altering the behavior of the superclass.
- Be wary of violating the LSP when using conditional statements to check the type of an object before performing operations.
// Example of LSP Violation
public class Rectangle {
protected int width;
protected int height;
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
public int area() {
return width * height;
}
}
public class Square extends Rectangle {
@Override
public void setWidth(int width) {
this.width = width;
this.height = width;
}
@Override
public void setHeight(int height) {
this.width = height;
this.height = height;
}
}
The Square
class violates the LSP by changing the behavior of the setWidth
and setHeight
methods to maintain the square's invariant. This violates the expected behavior of the base class, Rectangle
.
Interface Segregation Principle (ISP)
The ISP states that a client should not be forced to implement interfaces they don't use. To adhere to ISP:
- Divide large interfaces into smaller, more specific interfaces based on client requirements.
- Use composition or delegation to achieve the segregation of interfaces rather than forcing clients to implement unnecessary methods.
// Example of ISP Violation
public interface Worker {
void work();
void eat();
void takeBreak();
}
public class Robot implements Worker {
@Override
public void work() {
// work logic
}
@Override
public void eat() {
// eat logic
}
@Override
public void takeBreak() {
// take break logic
}
}
In this example, the Robot
class is forced to implement the eat
and takeBreak
methods, which are unnecessary for a robot. This violates the ISP, and the Worker
interface should be segregated into more specific interfaces such as Workable
and Eatable
.
Dependency Inversion Principle (DIP)
The DIP states that high-level modules should not depend on low-level modules. Both should depend on abstractions. To apply DIP:
- Use interfaces or abstractions to decouple high-level and low-level modules, allowing for flexibility and easy substitution of implementations.
- Practice dependency injection to invert the dependency relationship and promote loosely coupled code.
- Leverage inversion of control containers like Spring to manage dependencies and promote decoupling.
// Example of DIP Violation
public class FrontendDeveloper {
private BackendDeveloper backendDeveloper = new BackendDeveloper();
public void implementFeature() {
backendDeveloper.writeBackendLogic();
// frontend logic
}
}
In the above code, the FrontendDeveloper
class directly depends on the BackendDeveloper
class, violating the DIP. Instead, it should depend on an abstraction (interface) and utilize dependency injection to decouple from the concrete implementation.
The Last Word
In conclusion, applying SOLID principles in Java development is essential for writing maintainable, scalable, and flexible code. By adhering to the SRP, OCP, LSP, ISP, and DIP, developers can produce robust and easily maintainable software systems.
Remember, while these principles may seem abstract at first, following them diligently will lead to cleaner, more maintainable, and extensible code in the long run.
For more practical tips on writing clean and efficient Java code, check out Clean Code Java. Additionally, if you want to delve deeper into design patterns and principles, Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides is an excellent resource.
Happy coding!