Designing Scalable Cloud Applications
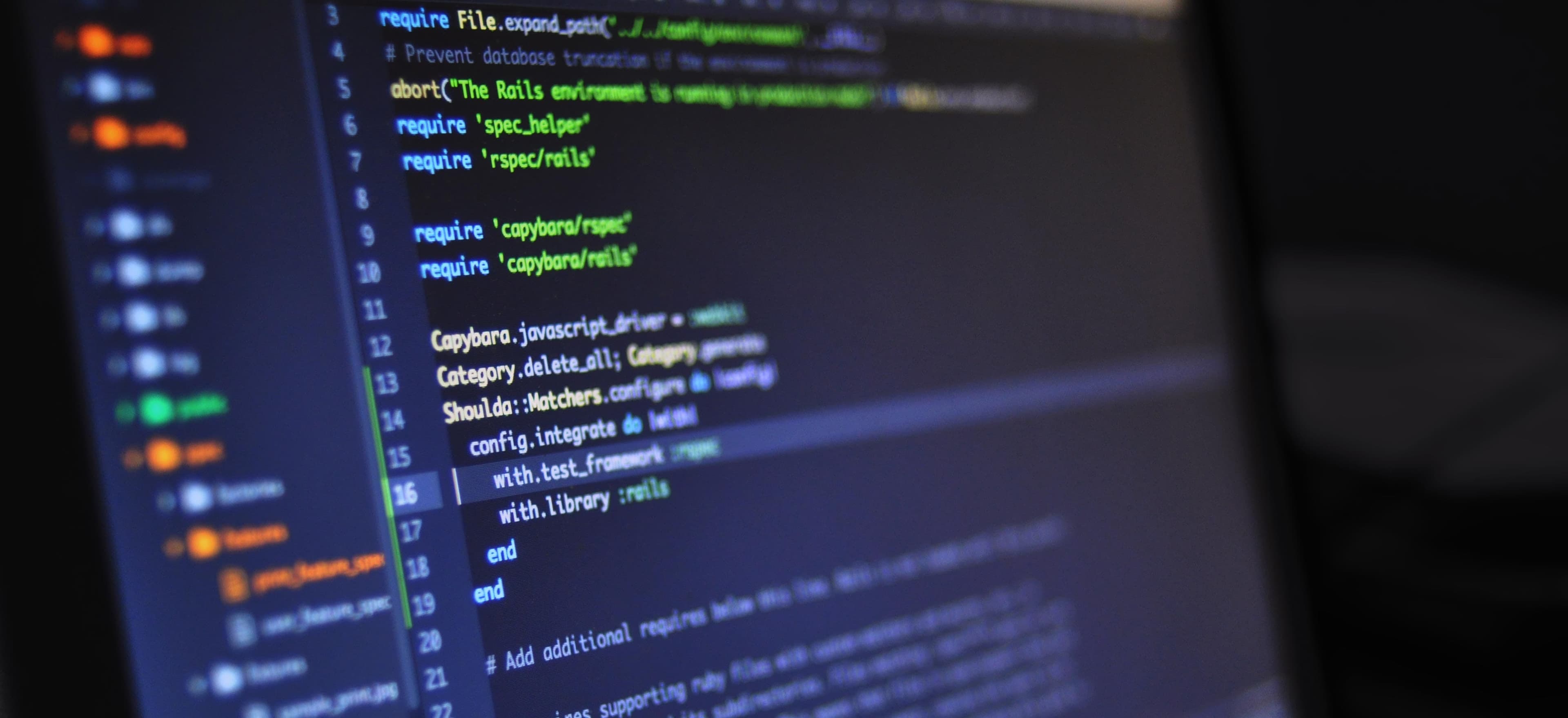
- Published on
Designing Scalable Cloud Applications with Java
In today's digital era, the demand for scalable cloud applications has skyrocketed. As a Java developer, it's crucial to understand how to design and implement cloud applications that can handle a growing number of users and data without compromising performance. In this article, we will explore the key principles and best practices for designing scalable cloud applications using Java.
Understanding Scalability
Scalability is the ability of a system to handle an increasing amount of work by adding resources to the system. This can be achieved by two main approaches: vertical scalability (adding more power to an existing machine) and horizontal scalability (adding more machines to distribute the load).
In cloud computing, horizontal scalability is more commonly used as it allows applications to scale out by adding more instances to the cloud infrastructure. This approach aligns with the fundamental principles of cloud computing, including elasticity and on-demand resource allocation.
Leveraging Java for Scalable Cloud Applications
Java, with its strong ecosystem and rich set of libraries, is well-suited for developing scalable cloud applications. It provides a robust foundation for building distributed and concurrent systems, essential for handling large-scale workloads in the cloud.
Asynchronous Programming
Java offers excellent support for asynchronous programming through its CompletableFuture
and ExecutorService
APIs. Leveraging asynchronous programming allows applications to perform non-blocking I/O operations and handle a large number of concurrent requests more efficiently.
Let's take a look at an example of using CompletableFuture
to perform asynchronous tasks:
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Perform asynchronous task here
});
By using asynchronous programming, applications can make better use of available resources and improve overall throughput, which is crucial for scalability in the cloud.
Choosing the Right Frameworks
When designing scalable cloud applications in Java, choosing the right frameworks is essential. Frameworks such as Spring Boot, Micronaut, and Quarkus provide extensive support for building cloud-native applications with features like auto-scaling, service discovery, and fault tolerance.
For instance, Spring Boot's auto-configuration and support for embedded containers make it a popular choice for developing microservices that can easily scale in a cloud environment.
@SpringBootApplication
public class ProductServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
}
By leveraging these frameworks, developers can expedite the development of scalable cloud applications while benefiting from built-in features designed for cloud environments.
Implementing Caching
Effective caching mechanisms play a crucial role in improving the scalability of cloud applications. Java developers can utilize frameworks like Ehcache or Caffeine to implement in-memory caching, reducing the load on backend services and enhancing overall application performance.
Cache<String, Product> productCache = Caffeine.newBuilder()
.maximumSize(1000)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
Caching frequently accessed data can significantly reduce response times and alleviate the pressure on databases, leading to better scalability and resource utilization.
Ensuring Data Consistency and Persistence
When designing scalable cloud applications, ensuring data consistency and persistence is paramount. Distributed systems and microservices architecture bring forth challenges in maintaining data integrity across the application.
Event Sourcing and CQRS
Event sourcing and CQRS (Command Query Responsibility Segregation) are patterns that can be employed to achieve scalable and consistent data management in cloud applications. Implementing these patterns often involves the use of frameworks like Axon Framework or Apache Kafka, which provide the necessary infrastructure for event-driven architectures.
By embracing event sourcing and CQRS, developers can design highly scalable systems that effectively manage data consistency and enable seamless horizontal scalability.
Distributed Data Stores
Utilizing distributed data stores, such as Apache Cassandra or MongoDB, is essential for maintaining data persistence in scalable cloud applications. These NoSQL databases offer horizontal scalability and fault tolerance, making them well-suited for cloud environments.
Cluster cluster = Cluster.builder().addContactPoint("localhost").build();
Session session = cluster.connect("mykeyspace");
By leveraging distributed data stores, applications can ensure that data storage can seamlessly scale out as the application's workload grows.
Monitoring and Auto-Scaling
Monitoring the performance of a cloud application is crucial to determine when and how to scale the application. Java developers can incorporate monitoring solutions like Prometheus or New Relic to collect and analyze key performance metrics. Additionally, cloud providers like AWS and Azure offer auto-scaling capabilities that can dynamically adjust the application's capacity based on demand.
By implementing monitoring and auto-scaling mechanisms, applications can effectively adapt to changing workloads and ensure optimal performance and resource utilization.
Bringing It All Together
Designing scalable cloud applications with Java requires a deep understanding of distributed systems, asynchronous programming, data management, and infrastructure orchestration. By leveraging Java's powerful features and embracing cloud-native best practices, developers can build high-performance, scalable applications capable of meeting the demands of modern cloud environments.
Incorporating best practices such as asynchronous programming, choosing the right frameworks, implementing caching, ensuring data consistency, and monitoring for auto-scaling are crucial steps towards building scalable cloud applications with Java. As technology continues to evolve, embracing these principles will be imperative for staying ahead in the rapidly advancing cloud landscape.
To delve deeper into scalable cloud architecture and Java programming, you can explore in detail the AWS Cloud Architecture Best Practices and the Java Concurrency in Practice book by Brian Goetz.