Efficient Cross-Browser Testing with LambdaTest
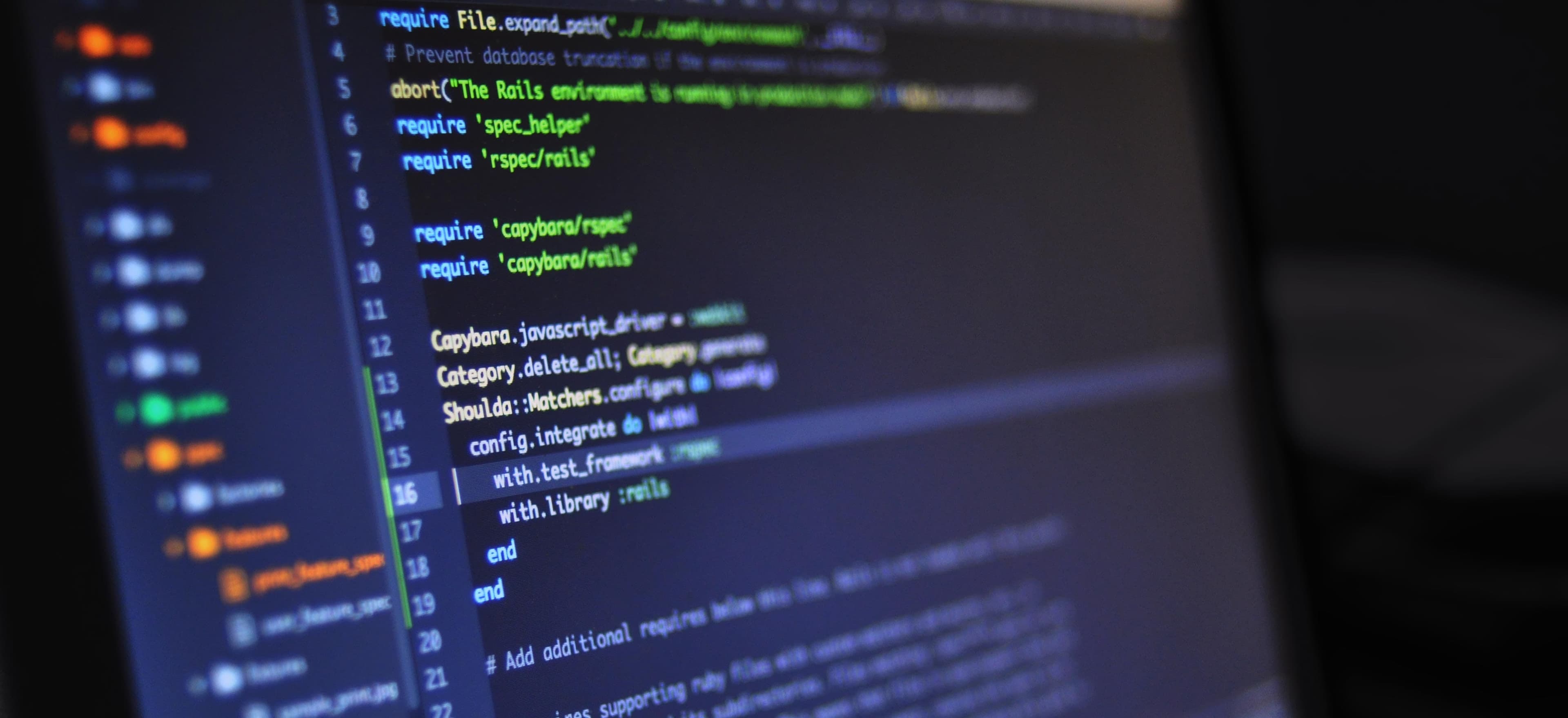
- Published on
The Importance of Cross-Browser Testing
As a Java developer, ensuring the compatibility of your web application across different browsers is critical. Users access your application through various browsers, each with its own rendering engine and unique quirks. Failing to test your app across multiple browsers can lead to a poor user experience, loss of potential customers, and a tarnished reputation.
Enter Cross-Browser Testing Tools
One way to ensure your web application looks and works as intended across different browsers is through cross-browser testing. This involves running your application in various browsers to identify and fix any discrepancies in how the application is rendered or behaves. However, manually testing your application across different browsers is time-consuming and error-prone.
This is where cross-browser testing tools like LambdaTest come into play.
Introducing LambdaTest
LambdaTest is a cloud-based cross-browser testing platform that allows you to test your website or web application on a combination of browsers and operating systems. With LambdaTest, you can perform live interactive testing or automate your tests using their Selenium Grid.
Advantages of Using LambdaTest
-
Wide Range of Browsers and Devices: LambdaTest provides access to a wide range of browsers, including older versions, and mobile devices, allowing you to simulate real user scenarios.
-
Scalability: You can run tests in parallel across multiple browsers, which can significantly reduce testing time.
-
Integration: LambdaTest integrates seamlessly with popular CI/CD tools and bug tracking systems, such as Jenkins, Jira, and Slack.
-
Bug Logging: You can log bugs directly from the LambdaTest platform, streamlining the debugging process.
Now let's dive into using LambdaTest with Java for efficient cross-browser testing.
Using LambdaTest with Java
Getting Started
The first step is to create a LambdaTest account and retrieve your LambdaTest Username and Access Key.
Next, you'll need to set up your Java project to use Selenium WebDriver, which provides a programming interface for interacting with web browsers. You can either add the Selenium dependency directly to your project or use a build tool like Maven or Gradle.
Using Selenium WebDriver with LambdaTest
With Selenium WebDriver, you can leverage LambdaTest's Selenium Grid to execute tests across different browsers. Here's a simple example of how you can use Selenium WebDriver with LambdaTest.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class LambdaTestExample {
public static void main(String[] args) {
// Set your LambdaTest username and access key
String username = "YOUR_USERNAME";
String accessKey = "YOUR_ACCESS_KEY";
// Set the desired capabilities
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("browserName", "Chrome");
capabilities.setCapability("version", "latest");
capabilities.setCapability("platform", "Windows 10");
capabilities.setCapability("build", "LambdaTestSampleApp");
capabilities.setCapability("name", "SampleTest");
// Initialize the WebDriver
try {
WebDriver driver = new RemoteWebDriver(new URL("https://" + username + ":" + accessKey +
"@hub.lambdatest.com/wd/hub"), capabilities);
// Navigate to the website to be tested
driver.get("https://www.example.com");
// Perform testing actions
// ...
// Quit the WebDriver session
driver.quit();
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
In this example, we use the RemoteWebDriver
provided by Selenium to initialize a connection to LambdaTest's Selenium Grid. We set the desired capabilities to specify the browser, version, platform, and other test details. This allows the test to run on a specific browser and platform configuration provided by LambdaTest.
Running Tests in Parallel
One of the key advantages of using LambdaTest is the ability to run tests in parallel across multiple browsers. This can significantly reduce the overall test execution time, enabling you to get quick feedback on the compatibility of your web application across various browsers.
You can achieve parallel testing by incorporating testing frameworks like TestNG or JUnit and leveraging LambdaTest's capabilities to execute tests across different browser configurations simultaneously.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class ParallelTestingExample {
public static void main(String[] args) {
// Set your LambdaTest username and access key
String username = "YOUR_USERNAME";
String accessKey = "YOUR_ACCESS_KEY";
// Define an array of DesiredCapabilities for the browsers to be tested
DesiredCapabilities[] capabilitiesArray = new DesiredCapabilities[]{
new DesiredCapabilities(), // Capability for Browser 1
new DesiredCapabilities() // Capability for Browser 2
// Add more browsers as needed
};
try {
for (DesiredCapabilities capabilities : capabilitiesArray) {
// Set the desired capabilities for each browser
capabilities.setCapability("browserName", "Chrome"); // Example browser
capabilities.setCapability("version", "latest");
capabilities.setCapability("platform", "Windows 10");
// Set other capabilities
// Initialize and run the WebDriver session for each browser
WebDriver driver = new RemoteWebDriver(new URL("https://" + username + ":" + accessKey +
"@hub.lambdatest.com/wd/hub"), capabilities);
// Perform testing actions
// ...
// Quit the WebDriver session
driver.quit();
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
In this example, we create an array of DesiredCapabilities
representing the browsers on which we want to run tests. We then iterate through the array, initializing a new RemoteWebDriver session for each browser using the corresponding desired capabilities. This allows us to execute tests in parallel across multiple browsers.
Integrating with CI/CD Pipelines
To further streamline your testing process, you can integrate LambdaTest with your CI/CD pipelines. This ensures that each code change triggers a full suite of cross-browser tests, providing quick feedback on any compatibility issues introduced during development.
Popular CI/CD tools such as Jenkins, TeamCity, and Travis CI can be configured to trigger LambdaTest tests as part of the build and deployment process. This integration automates the cross-browser testing workflow, making it an integral part of your development lifecycle.
A Final Look
Ensuring the compatibility of your web application across different browsers is crucial for providing a seamless user experience. LambdaTest, with its wide range of browsers, scalability, and seamless integration, provides an efficient platform for performing cross-browser testing.
By leveraging LambdaTest with Java and Selenium WebDriver, you can streamline your cross-browser testing efforts, run tests in parallel, and integrate them into your CI/CD pipelines, ultimately delivering a robust and consistent user experience across various browsers.
Start incorporating LambdaTest into your Java web development workflow and elevate the quality of your web applications with comprehensive cross-browser testing.