Efficient Prime Factorization for Integer Numbers
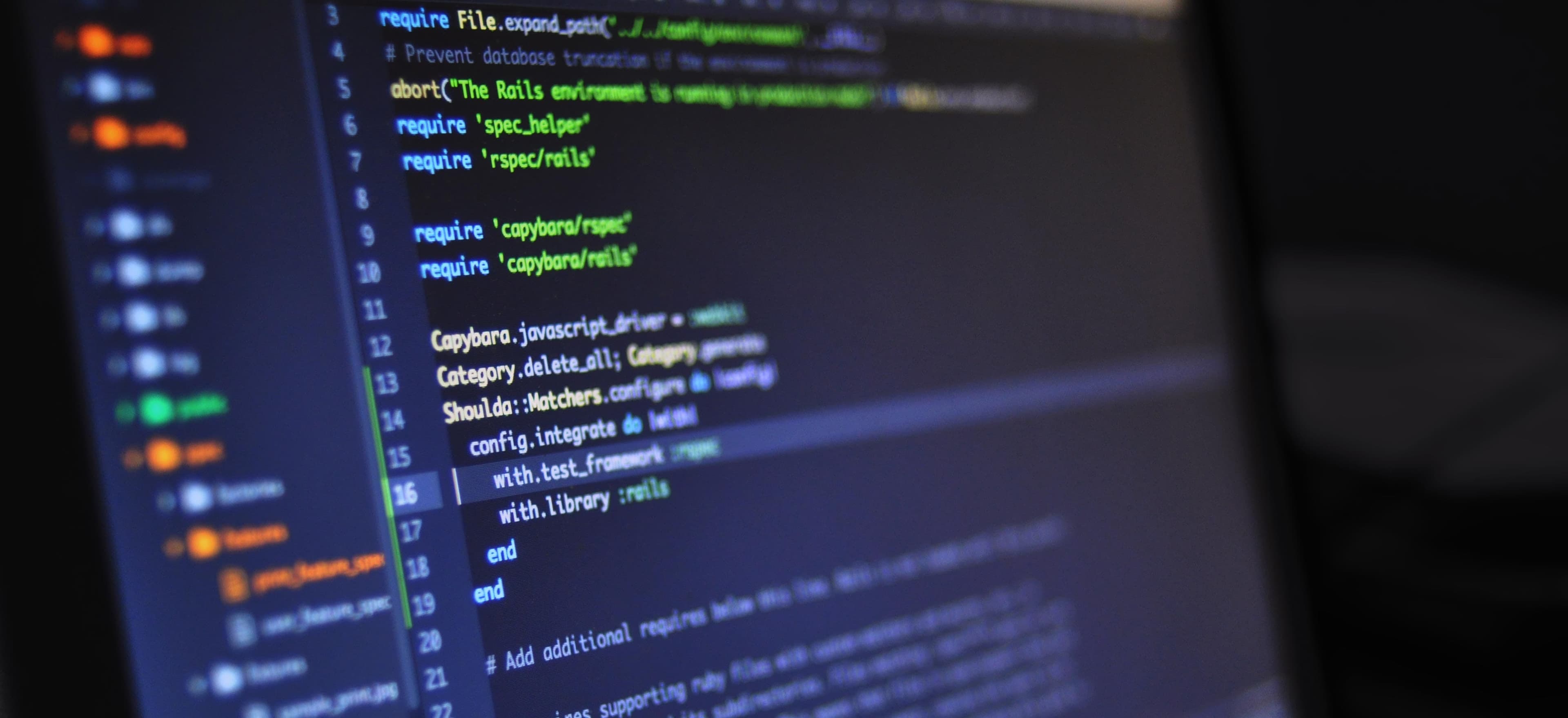
- Published on
Efficient Prime Factorization for Integer Numbers
Prime factorization is the process of finding the prime numbers that multiply together to give the original integer. In this blog post, we will explore an efficient way to perform prime factorization for integer numbers using Java.
The Approach to Prime Factorization
Prime factorization is a fundamental concept in number theory and has various applications in cryptography, computer science, and mathematics. The prime factorization of a number is a unique representation of that number as a product of prime numbers.
For example, the prime factorization of the number 12 is 2 * 2 * 3, where 2 and 3 are prime numbers. Prime factorization is essential for simplifying fractions, finding the greatest common divisor (GCD) of two numbers, and solving certain types of equations.
Naive Approach to Prime Factorization
The simplest approach to prime factorization is to use trial division, where we divide the number by increasingly larger prime numbers until the quotient becomes 1. While this approach is straightforward, it can be inefficient for large numbers, especially when the prime factors are large.
public static void primeFactorization(int n) {
for (int i = 2; i <= n; i++) {
while (n % i == 0) {
System.out.print(i + " ");
n = n / i;
}
}
}
In the above code snippet, we iterate through all numbers from 2 to n
and divide n
by each number to check for divisibility. If the current number is a factor, we print it and update the value of n
by dividing it by the factor.
While this approach works, its time complexity is O(N), making it inefficient for large numbers with multiple prime factors.
Efficient Approach using Sieve of Eratosthenes
To efficiently factorize large numbers, we can use the Sieve of Eratosthenes to generate a list of prime numbers up to a certain limit. We can then use these prime numbers to factorize the given number.
import java.util.*;
public class PrimeFactorization {
public static void primeFactorization(int n) {
List<Integer> primeNumbers = generatePrimes((int) Math.sqrt(n) + 1);
for (int prime : primeNumbers) {
while (n % prime == 0) {
System.out.print(prime + " ");
n = n / prime;
}
}
if (n > 1) {
System.out.print(n);
}
}
private static List<Integer> generatePrimes(int limit) {
boolean[] isPrime = new boolean[limit + 1];
Arrays.fill(isPrime, true);
for (int i = 2; i * i <= limit; i++) {
if (isPrime[i]) {
for (int j = i * i; j <= limit; j += i) {
isPrime[j] = false;
}
}
}
List<Integer> primes = new ArrayList<>();
for (int i = 2; i <= limit; i++) {
if (isPrime[i]) {
primes.add(i);
}
}
return primes;
}
}
In the above code, we first generate a list of prime numbers up to the square root of the given number using the generatePrimes
method. We then iterate through the list of primes and divide the number n
by each prime while it is divisible. Finally, if the remaining n
is greater than 1, it is also a prime factor and should be printed.
By using the Sieve of Eratosthenes to generate prime numbers, we reduce the time complexity of the prime factorization algorithm to O(sqrt(N)), making it much more efficient than the naive approach for large numbers.
To Wrap Things Up
Efficient prime factorization is crucial in various mathematical and computational scenarios. By leveraging the Sieve of Eratosthenes to generate prime numbers, we can significantly improve the performance of prime factorization algorithms for large numbers.
In this blog post, we discussed the concept of prime factorization, explored a naive approach using trial division, and demonstrated an efficient approach using the Sieve of Eratosthenes. By understanding and implementing these algorithms, we can effectively factorize integer numbers in Java and handle a wide range of mathematical computations.
For further exploration of prime factorization and its applications, you can refer to the Wikipedia page on prime factorization.
Prime factorization is a fascinating topic with diverse applications, and mastering efficient algorithms for prime factorization can be immensely rewarding for Java developers.