Creating a Fat JAR for Your Java Application
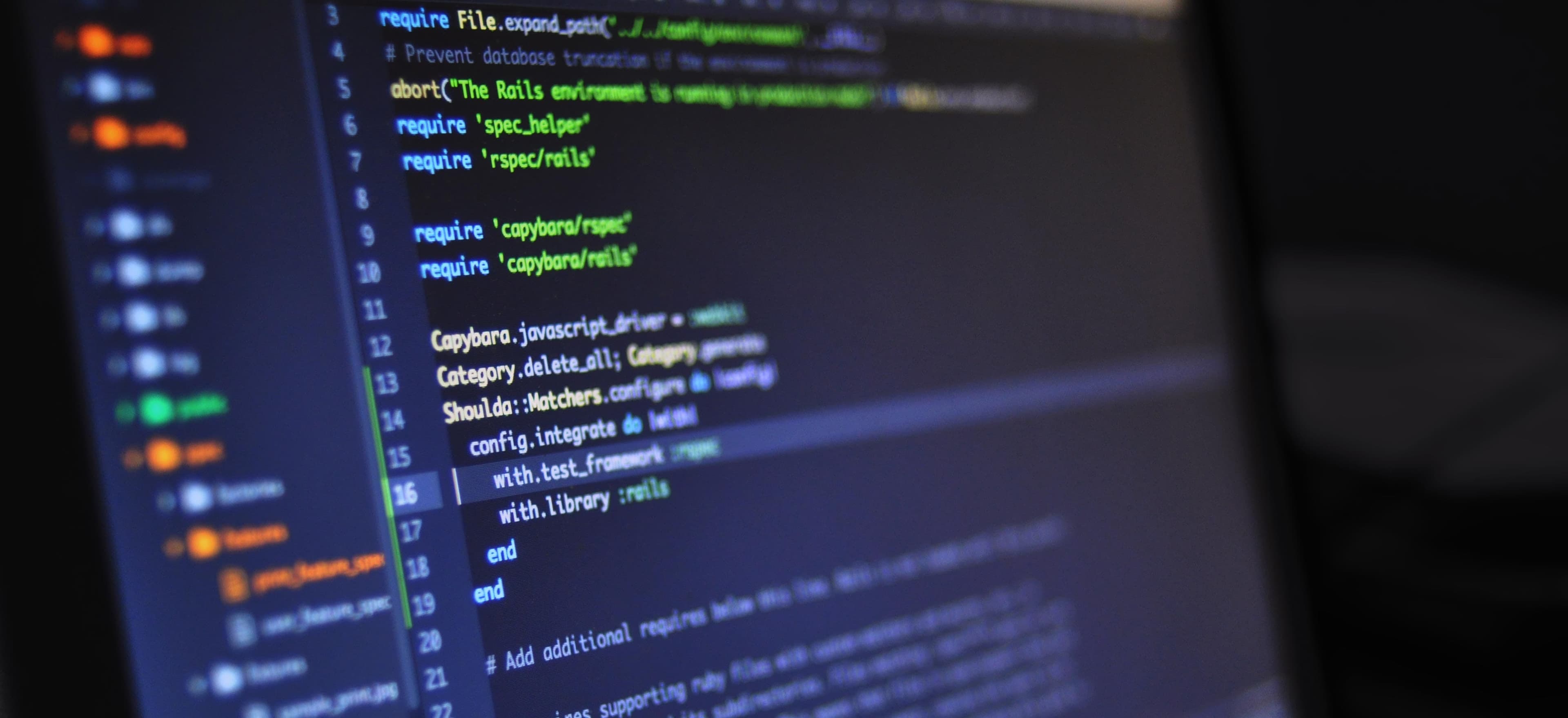
- Published on
In the world of Java development, creating a Fat JAR is a common practice to simplify the deployment of applications. A Fat JAR, also known as an uber-jar, is a self-contained executable JAR file that contains all the dependencies needed to run a Java application. This means that you can distribute your application as a single JAR file without worrying about the end user having to install and configure additional libraries or dependencies.
In this tutorial, we'll explore the process of creating a Fat JAR for a Java application. We'll discuss the benefits of using Fat JARs, the tools and techniques involved, and provide code examples to illustrate the process.
Why Use a Fat JAR?
Before diving into the technical details, let's take a moment to understand why Fat JARs are a popular choice for Java applications.
Simplified Dependency Management
One of the main advantages of using Fat JARs is that they simplify dependency management. Instead of having to manage a separate set of dependencies and ensure that they are installed and configured correctly on the target system, you can package all the dependencies into a single executable JAR file. This makes the deployment process much simpler and less error-prone.
Portability
Fat JARs are highly portable. Since they contain all the necessary dependencies, they can be easily transferred and executed on different systems without worrying about compatibility issues or missing libraries.
Self-Containment
By bundling all the dependencies into a single JAR file, you create a self-contained unit that is easier to manage, distribute, and execute. This reduces the likelihood of version conflicts and other runtime issues that can arise when dealing with external dependencies.
Tools for Creating Fat JARs
Now that we understand the benefits of using Fat JARs, let's explore the tools and techniques for creating them.
Maven Shade Plugin
One of the most popular tools for creating Fat JARs in the Java ecosystem is the Maven Shade Plugin. The Shade Plugin allows you to package your project and its dependencies into a single JAR file, resolving any conflicts that may arise from overlapping dependencies.
To use the Maven Shade Plugin, you need to include the following configuration in your project's pom.xml
file:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<createDependencyReducedPom>false</createDependencyReducedPom>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
In this configuration, the maven-shade-plugin
is bound to the package
phase, ensuring that the Fat JAR is created as part of the build process. The createDependencyReducedPom
element is set to false
to prevent the plugin from generating a reduced POM file.
Gradle Shadow Plugin
For those using Gradle as their build tool, the Shadow Plugin provides similar functionality to the Maven Shade Plugin. It allows you to create Fat JARs by merging project dependencies and classes into a single JAR file.
To use the Gradle Shadow Plugin, you need to include the following configuration in your project's build.gradle
file:
plugins {
id 'com.github.johnrengelman.shadow' version '7.0.0'
}
shadowJar {
mergeServiceFiles()
merge 'META-INF/*.sf'
}
In this configuration, we apply the com.github.johnrengelman.shadow
plugin, which enables the shadowJar
task. The mergeServiceFiles()
method and the merge 'META-INF/*.sf'
statement ensure that service files and signature files are properly merged when creating the Fat JAR.
Creating a Fat JAR
Now that we've covered the tools and techniques for creating Fat JARs, let's walk through the process of actually creating one for a sample Java application.
For this example, let's assume we have a simple Java application with the following directory structure:
./
├── src/
│ └── main/
│ └── java/
│ └── com/
│ └── example/
│ └── MyApp.java
└── pom.xml
Our goal is to create a Fat JAR that includes the application classes along with their dependencies.
Maven Approach
If you're using Maven, you can create a Fat JAR by running the package
phase:
mvn package
Once the build is complete, the Fat JAR will be available in the target
directory with a name like my-app-1.0-SNAPSHOT.jar
. You can run this JAR using the java -jar
command:
java -jar target/my-app-1.0-SNAPSHOT.jar
Gradle Approach
If you're using Gradle, you can create a Fat JAR by running the shadowJar
task:
./gradlew shadowJar
Similar to the Maven approach, the Fat JAR will be generated in the build/libs
directory with a name like my-app-1.0-SNAPSHOT-all.jar
. You can execute this JAR using the java -jar
command just like with the Maven-generated Fat JAR.
Final Considerations
In this tutorial, we've explored the concept of Fat JARs and discussed the benefits of using them for Java applications. We've also delved into the tools and techniques for creating Fat JARs, including the Maven Shade Plugin and the Gradle Shadow Plugin. Lastly, we walked through the process of creating a Fat JAR for a sample Java application using both Maven and Gradle.
By mastering the creation of Fat JARs, you can simplify the deployment process for your Java applications and make them more portable and self-contained. Whether you're working on a small project or a large-scale application, understanding and utilizing Fat JARs can significantly streamline your development workflow and enhance the overall user experience.
Now that you have the knowledge and tools to create Fat JARs, give it a try with your own Java project and experience the benefits firsthand. Happy coding!
For more in-depth information on Fat JARs and Java application deployment, you can refer to the official Maven Shade Plugin documentation and the Gradle Shadow Plugin documentation.