Comparing Two Lists for Equality in Java
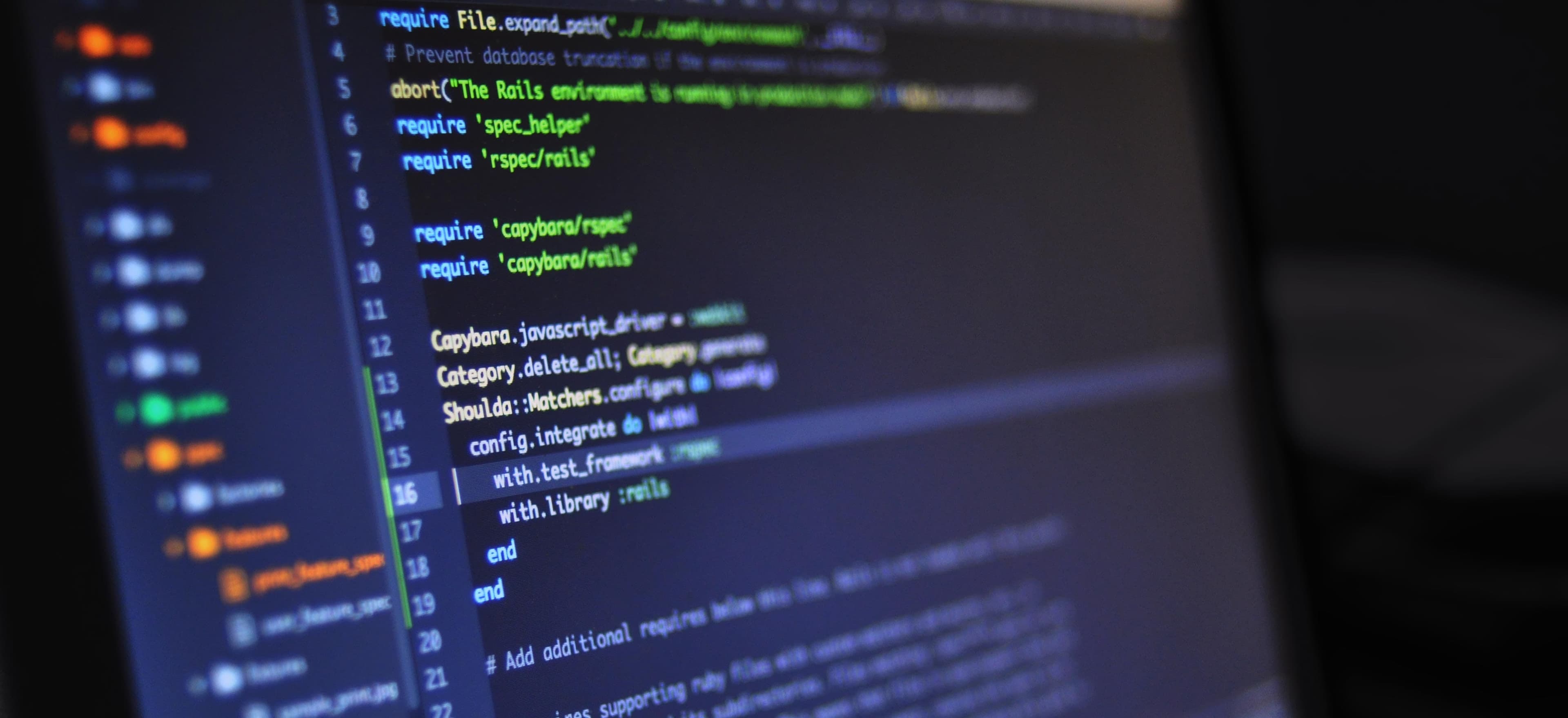
- Published on
In the realm of Java development, there often arises the need to compare two lists to check for equality. Whether you're working with database queries, handling user input, or manipulating collections, the task of comparing lists is a common necessity. In this guide, we'll explore various approaches to compare two lists for equality in Java, and discuss the pros and cons of each method.
Comparing by Elements
One straightforward approach to compare two lists is by examining their individual elements. We can start off by writing a method that iterates through both lists and compares each element at the corresponding index. Let's see an illustrative example.
public boolean compareListsByElements(List<String> list1, List<String> list2) {
if (list1.size() != list2.size()) {
return false;
}
for (int i = 0; i < list1.size(); i++) {
if (!list1.get(i).equals(list2.get(i))) {
return false;
}
}
return true;
}
This approach is intuitive and gives us a clear view of how the comparison works. We compare the size of the lists, and then iterate through their elements, checking for equality at each index. However, this method has a downside: it doesn't account for lists with the same elements but in a different order. For example, lists [A, B]
and [B, A]
would be considered unequal, even though they contain the same elements.
Using Collection Methods
Java provides built-in methods in the Collections
class to simplify the process of comparing lists. The containsAll()
method is particularly useful in this scenario, as it allows us to check if one list contains all the elements of another list, regardless of their order.
Let's see how we can utilize this method to compare two lists for equality.
public boolean compareListsUsingCollectionMethods(List<String> list1, List<String> list2) {
return list1.size() == list2.size() && list1.containsAll(list2);
}
In this implementation, we first compare the sizes of the lists to ensure they're equal. Then, we use the containsAll()
method to check if all elements of list2
are present in list1
. This method is concise and efficient, and it effectively handles scenarios where the order of elements doesn't matter.
However, it's important to note that this approach still falls short if the lists contain duplicate elements. The containsAll()
method doesn't consider the frequency of elements, so it might inaccurately deem lists with different element frequencies as equal.
Leveraging Java 8 Streams
With the advent of Java 8, we gained access to powerful stream operations that provide elegant solutions for list comparisons. Utilizing streams, we can compare two lists using a combination of sorting and equality checks. Let's delve into the code to see the stream-based approach in action.
import java.util.stream.Collectors;
public boolean compareListsWithStreams(List<String> list1, List<String> list2) {
return list1.size() == list2.size() &&
list1.stream().sorted().collect(Collectors.toList())
.equals(list2.stream().sorted().collect(Collectors.toList()));
}
In this implementation, we leverage Java 8 streams to first sort both lists and then check for equality using the equals()
method. The use of streams adds a functional programming flavor to the comparison process, and the sorting ensures that the order of elements doesn't affect the equality check.
This approach is concise and handles scenarios involving unordered lists. However, it incurs the overhead of sorting the lists, which may impact performance for very large collections.
Apache Commons Collections
Another noteworthy approach involves using the Apache Commons Collections library, which provides a ListUtils
class with a convenient method for comparing lists. While this method introduces an external dependency, it can be a valuable addition for projects where the library is already in use.
Let's explore how we can employ Apache Commons Collections to compare two lists for equality.
import org.apache.commons.collections4.ListUtils;
public boolean compareListsWithApacheCommons(List<String> list1, List<String> list2) {
return ListUtils.isEqualList(list1, list2);
}
In this concise implementation, we use the isEqualList()
method provided by Apache Commons Collections to directly compare the equality of the two lists. This method internally considers the frequency and order of elements, providing a comprehensive comparison.
The use of Apache Commons Collections can be beneficial for projects that already utilize the library or that require additional functionality beyond basic list comparisons. However, introducing an external dependency solely for list comparison might be considered excessive in some contexts.
The Closing Argument
In the realm of Java development, the need to compare lists for equality is a common requirement. We've explored several approaches, each with its own strengths and limitations. The choice of method depends on the specific needs of a project, considering factors such as performance, element order, and external dependencies.
Whether you opt for the simplicity of element-by-element comparison, the elegance of Java 8 streams, the convenience of collection methods, or the comprehensive features of external libraries, it's crucial to select an approach that aligns with the unique demands of the task at hand. By understanding these comparison methods, Java developers can make informed decisions in handling list comparisons efficiently and effectively.
Checkout our other articles