Understanding the Impact of Java Bytecode on Developers
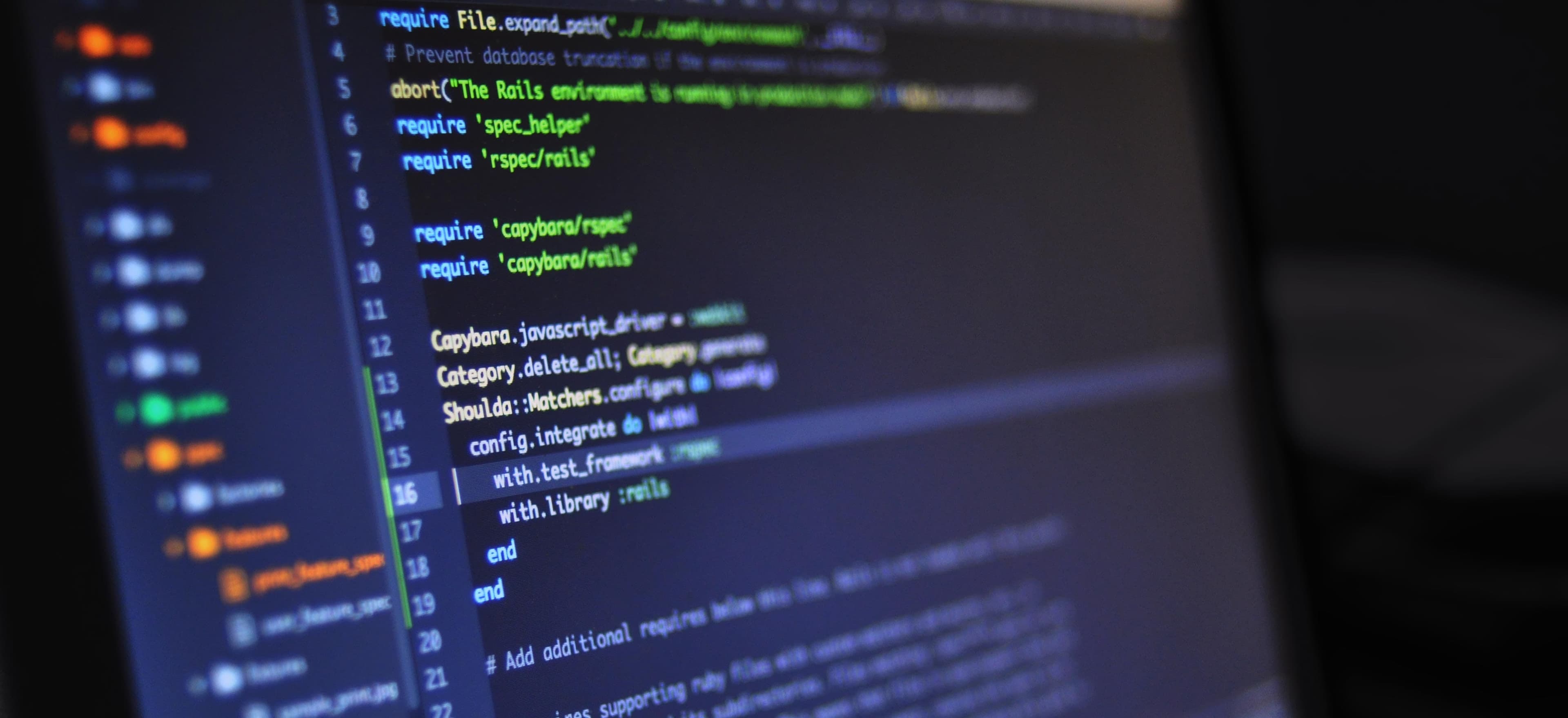
- Published on
Understanding the Impact of Java Bytecode on Developers
When Java was first introduced by Sun Microsystems in 1995, it brought with it a groundbreaking concept - "write once, run anywhere". This was made possible through the use of Java bytecode, an intermediate representation of Java source code that allows Java programs to be executed on any device or platform that has a Java Virtual Machine (JVM) installed.
In this blog post, we'll delve into the world of Java bytecode, exploring its impact on developers and the broader Java ecosystem.
What is Java Bytecode?
Java bytecode is the instruction set of the Java virtual machine. When a Java source file is compiled, it is translated into bytecode rather than machine code. This bytecode is platform-independent, allowing it to be executed on any device that has a compatible JVM.
For example, consider the following Java code snippet:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
When this code is compiled, it is converted into bytecode, which looks something like this:
0: getstatic #2
3: ldc #3
5: invokevirtual #4
8: return
This bytecode can then be executed by any JVM, regardless of the underlying architecture or operating system.
Impact on Development
Portability
One of the most significant impacts of Java bytecode is its ability to make Java programs highly portable. Developers can write code on one platform and be confident that it will run on any other platform with a compatible JVM. This "write once, run anywhere" capability has been a cornerstone of Java's success and has simplified the development and deployment of Java applications.
Security
Java bytecode also plays a critical role in Java's security model. The JVM can verify and validate bytecode before executing it, helping to prevent a wide range of security vulnerabilities such as buffer overflows and memory corruption. This provides a layer of protection for both developers and end users, making Java a popular choice for building secure applications.
Performance
While there was a time when the performance of interpreted bytecode was a concern, modern JVMs use sophisticated techniques such as dynamic adaptive optimization to achieve performance levels comparable to natively compiled languages. This means that developers can write high-level, platform-independent code without sacrificing performance.
Working with Java Bytecode
Understanding Java bytecode can be valuable for Java developers, especially when it comes to optimizing and troubleshooting their applications. Let's take a look at a few scenarios where knowledge of bytecode comes in handy.
Performance Tuning
By examining the bytecode generated from their Java source code, developers can gain insights into how their code is being translated and executed by the JVM. This understanding can help them make informed decisions about performance optimizations, such as reducing object creation or minimizing costly method invocations.
For example, let's consider the following Java code snippet:
public class SimpleMath {
public int add(int a, int b) {
return a + b;
}
}
When compiled and disassembled into bytecode, the add
method might look like this:
0: iload_1
1: iload_2
2: iadd
3: ireturn
By analyzing this bytecode, a developer can see the low-level operations performed by the JVM when executing the add
method. This insight can inform decisions about optimizing arithmetic operations for better performance.
Debugging and Diagnosing Issues
In some cases, troubleshooting complex issues in Java applications can be challenging, especially when using high-level debugging tools. By examining the bytecode of specific methods or classes, developers can gain a deeper understanding of how their code is behaving at the JVM level, helping to pinpoint the root causes of issues more effectively.
Tooling and Libraries
There are several tools and libraries available for working with Java bytecode. For example, libraries like ASM and Byte Buddy provide APIs for generating and transforming bytecode, offering developers the flexibility to manipulate bytecode at runtime for various purposes such as AOP (aspect-oriented programming) and dynamic code generation.
Bringing It All Together
Java bytecode is a cornerstone of the Java platform, enabling portability, security, and performance while empowering developers with powerful tools for optimization and troubleshooting. By understanding how bytecode works and how to work with it, Java developers can better harness the full potential of the JVM and build robust, efficient applications.
Whether it's optimizing performance-critical code, diving into the inner workings of a complex application, or leveraging bytecode manipulation libraries, a deeper understanding of Java bytecode empowers developers to take their Java skills to the next level.
To continue exploring Java bytecode and its impact, consider checking out the official Java Virtual Machine Specification here and the ASM bytecode manipulation framework here.