Unsynchronized Persistence Context in JPA 2.1
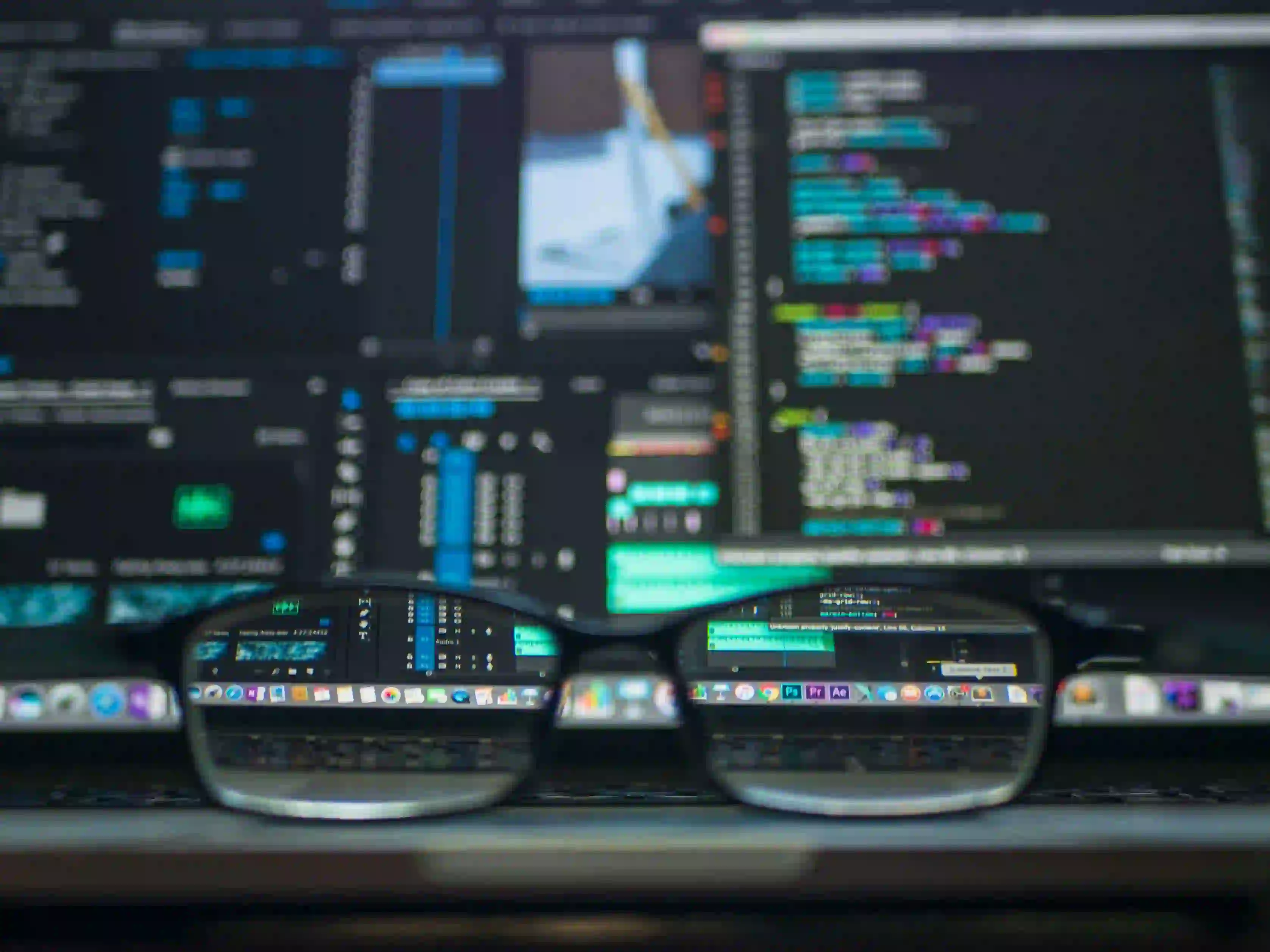
Understanding Unsynchronized Persistence Context in JPA 2.1
In the world of Java development, Java Persistence API (JPA) plays a pivotal role in managing entities, relationships, and persistence in Java applications. With the release of JPA 2.1, a new feature called Unsynchronized Persistence Context was introduced. This feature brings about a significant shift in managing the persistence context and provides developers with more flexibility and control.
What is a Persistence Context?
In JPA, a persistence context represents a set of managed entities, which are typically associated with a database transaction. It acts as a first-level cache for the entity instances, ensuring that changes made to these entities are eventually synchronized with the database.
The Need for Unsynchronized Persistence Context
Traditionally, in a Java EE environment, the persistence context is automatically synchronized with the database transaction boundaries. This means that any change made to the managed entities within a transaction is automatically reflected in the database upon transaction commit. However, in certain scenarios, this automatic synchronization may not be desirable.
Consider a situation where you need to make changes to the entity instances within a transaction, but you do not want these changes to be propagated to the database immediately. This is where the Unsynchronized Persistence Context comes into play. It allows you to decouple the synchronization of the persistence context from the transaction boundaries, giving you more control over when the changes are propagated to the database.
Working with Unsynchronized Persistence Context
To leverage the Unsynchronized Persistence Context in JPA 2.1, you can use the SynchronizationType
enum when declaring the persistence context in your entity manager.
@PersistenceContext(synchronization = SynchronizationType.UNSYNCHRONIZED)
private EntityManager entityManager;
By specifying SynchronizationType.UNSYNCHRONIZED
, you indicate that the persistence context associated with the injected EntityManager
will not be automatically synchronized with the database transaction boundaries.
Why Use Unsynchronized Persistence Context?
The Unsynchronized Persistence Context can be beneficial in various scenarios, such as:
-
Long-Running Conversations: In a web application, you may have long-running conversations where you want to keep the entity changes within the conversation scope without immediately persisting them to the database.
-
Batch Processing: During batch processing, you may need to perform a large number of entity updates within a single transaction. With unsynchronized persistence context, you can delay the database synchronization until the end of the batch process, improving performance and efficiency.
-
Complex Business Logic: In complex business logic, you may need to make intermediate changes to entities without committing them to the database until certain conditions are met.
Using Unsynchronized Persistence Context enables you to handle these scenarios effectively without being bound by the automatic synchronization behavior of the traditional persistence context.
Managing Entity State with Unsynchronized Persistence Context
When working with an Unsynchronized Persistence Context, it's essential to understand how the entity state is managed within the context. Any changes made to the managed entities will not be automatically propagated to the database, unlike in a synchronized persistence context.
To persist the changes to the database, you can explicitly call the EntityManager
's flush
method. This triggers the synchronization of the managed entity state with the database.
entityManager.persist(newEntity);
// Other operations...
entityManager.flush(); // Synchronize changes with the database
By explicitly calling flush
, you have full control over when the changes should be synchronized with the database, allowing you to manage the entity state according to the specific requirements of your application.
Caveats of Unsynchronized Persistence Context
While the Unsynchronized Persistence Context offers greater flexibility, it also comes with certain considerations:
-
Data Consistency: Since the changes are not automatically synchronized with the database, you must ensure data consistency by explicitly calling
flush
at the appropriate time to avoid inconsistencies. -
Memory Consumption: The unsynchronized persistence context may lead to increased memory consumption, especially when managing a large number of entity instances within a single transaction.
-
Transaction Management: You need to carefully manage the transaction boundaries and ensure that the changes are synchronized with the database at the right juncture to maintain data integrity.
Lessons Learned
In the realm of JPA 2.1, the addition of Unsynchronized Persistence Context brings forth a powerful mechanism for decoupling the synchronization of persistence context from the transaction boundaries. By using this feature, developers can effectively manage long-running conversations, optimize batch processing, and handle complex business logic with greater control over entity state management.
Understanding the intricacies of Unsynchronized Persistence Context and its implications is crucial for making informed decisions when designing and implementing JPA-based applications.
In summary, the Unsynchronized Persistence Context empowers developers to wield greater control over entity state management and database synchronization, paving the way for more flexible and efficient JPA-based solutions.
For deeper insights into JPA and persistence context management, you can explore the official documentation and additional resources to enhance your understanding of JPA best practices and advanced features.