Improper Usage of Java 14 Pattern Matching
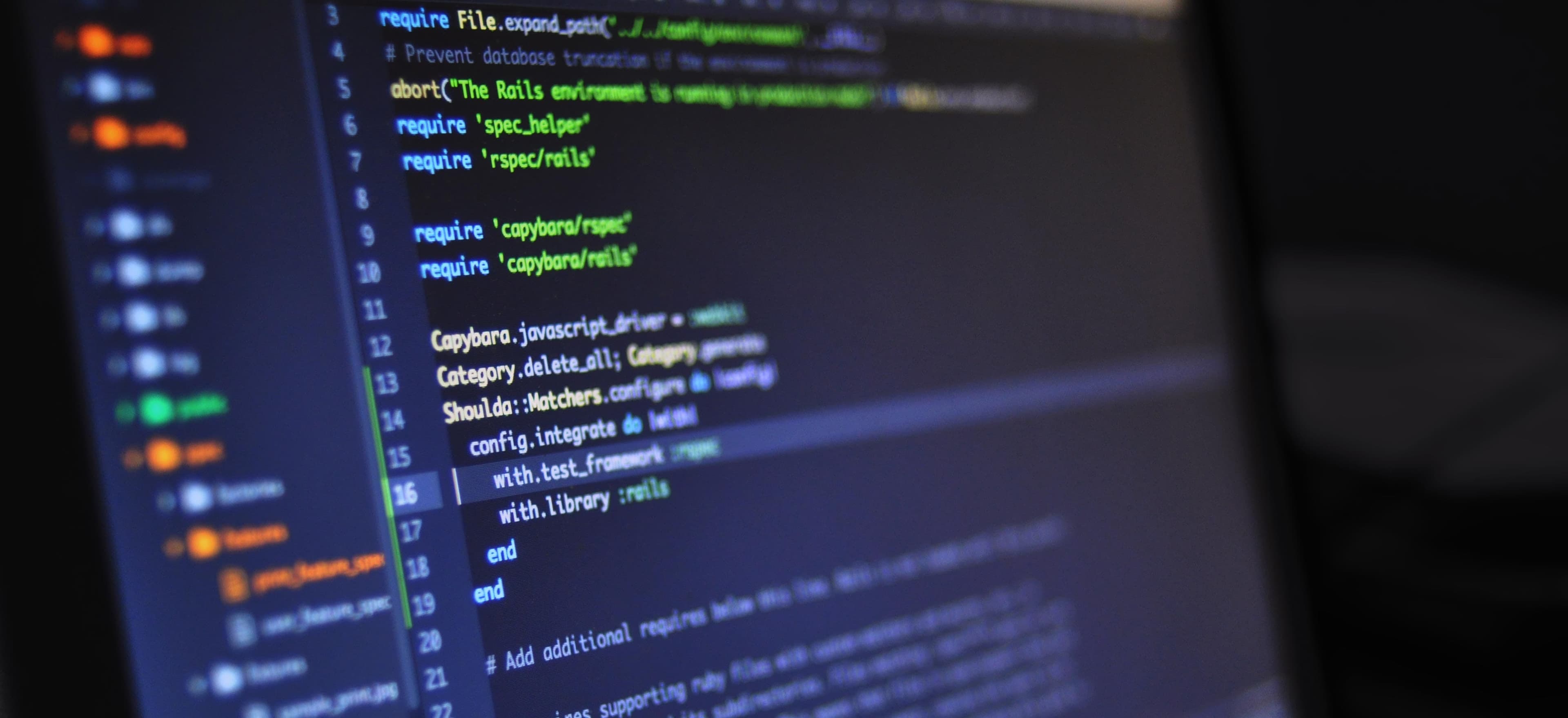
- Published on
Understanding Java 14 Pattern Matching and Its Common Pitfalls
Java 14 introduced pattern matching for the instanceof
operator as a feature to simplify common programming tasks. Pattern matching allows for inspecting data and extracting components of objects with more concise and readable code.
Despite its convenience, pattern matching in Java 14 can lead to errors and misunderstandings if not used properly. In this post, we'll delve into the proper usage of Java 14 pattern matching and highlight its common pitfalls.
Pattern Matching in instanceof
In previous versions of Java, checking the type of an object and then casting it to that type required multiple lines of code. With pattern matching in Java 14, this can be achieved in a single line. For example:
if (obj instanceof String str) {
// Access 'str' as a String type
System.out.println(str.length());
}
Here, the variable str
is assigned the value of obj
if obj
is an instance of String
. This eliminates the need for an additional cast and allows for immediate access to the object as the specified type.
Pitfalls of Improper Usage
1. Misuse of Variables
One common pitfall is the misuse of the variable introduced in the pattern matching expression. It's important to remember that the scope of the introduced variable is limited to the if
block where the pattern matching occurs. Attempting to access the variable outside of this block will result in a compilation error.
2. Overuse of Pattern Matching
While pattern matching can make code more compact and readable, overusing it in situations where traditional instanceof
checks suffice can lead to decreased code clarity. It's essential to use pattern matching judiciously, applying it where it provides a clear benefit in terms of code simplicity and readability.
3. Unexpected Behavior with Null Checks
Pattern matching in Java 14 can exhibit unexpected behavior when used in combination with null checks. For instance, consider the following code:
Object obj = null;
if (obj instanceof String str) {
// This block will not be executed, but 'str' will still be in scope
System.out.println(str.length());
}
In this scenario, the if
block will not be executed, yet the variable str
will be in scope, leading to potential confusion and bugs.
Best Practices for Pattern Matching
To leverage pattern matching effectively and avoid the common pitfalls, it's crucial to follow best practices:
1. Limit Variable Scope
Always ensure that the variable introduced in a pattern matching expression is used within the scope of the if
block where the pattern matching occurs. This helps to prevent accidental misuse of the introduced variable and enhances code clarity.
2. Use Pattern Matching Sparingly
Exercise discretion when applying pattern matching. Evaluate whether its use contributes to code simplification and readability in a given context. In cases where traditional instanceof
checks suffice, sticking to traditional syntax may be more appropriate.
3. Handle Null Checks
When employing pattern matching in scenarios involving potential null values, it's important to explicitly handle null checks to avoid unexpected behavior. This can be accomplished by combining pattern matching with null checks to ensure consistent and predictable program flow.
In Conclusion, Here is What Matters
Pattern matching in Java 14 introduces a powerful feature for simplifying type checks and casts. By understanding its proper usage and being mindful of potential pitfalls, developers can harness pattern matching to write clearer and more concise code.
To delve deeper into the intricacies of pattern matching and learn about its application in real-world scenarios, consider exploring official documentation and relevant tutorials. Additionally, practicing caution and incorporating best practices will pave the way for effectively integrating pattern matching into Java codebases.