Debugging Java in Production: A Practical Guide
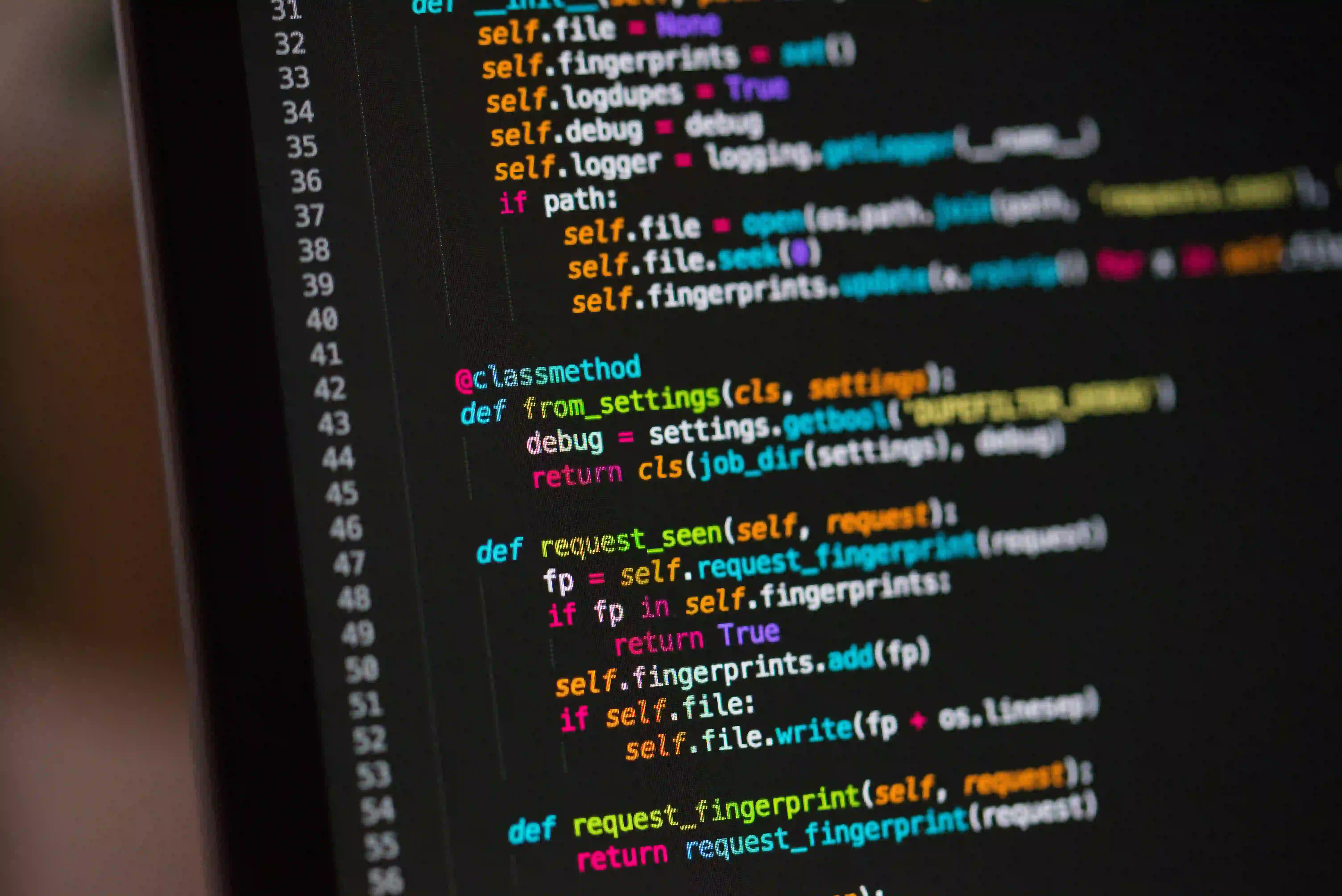
Debugging Java in Production: A Practical Guide
Debugging issues in a production environment can be a daunting task, especially when dealing with a high-traffic Java application. Fortunately, Java provides a range of tools and techniques to diagnose and resolve issues without disrupting the live system. In this guide, we'll explore best practices for debugging Java applications in production, including remote debugging, log analysis, and performance monitoring.
Remote Debugging
Remote debugging allows developers to connect to a live Java application and inspect its runtime behavior, even in a production environment. To enable remote debugging, you need to modify the application's startup parameters to include the appropriate JVM arguments.
java -agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=*:5005 -jar your-application.jar
-agentlib:jdwp
enables the Java Debug Wire Protocol (JDWP) agent for remote debugging.transport=dt_socket
specifies the transport type for the debugging connection.server=y
indicates that the JVM should listen for incoming debugger connections.suspend=n
ensures that the JVM does not suspend execution until a debugger is attached.address=*:5005
defines the port on which the debugger will listen for incoming connections.
Once the application is launched with remote debugging enabled, you can use your IDE or a standalone debugger, such as Eclipse or Visual Studio Code, to connect to the specified port and inspect the application's state, set breakpoints, and analyze the execution flow.
Log Analysis
Logging is a crucial aspect of production debugging, as it provides insights into the application's behavior and helps identify potential issues. In Java, logging is typically implemented using frameworks like Log4j or SLF4J.
By analyzing log files, you can gain visibility into application events, error messages, and performance metrics. Tools such as ELK Stack (Elasticsearch, Logstash, Kibana) and Splunk are commonly used for log aggregation, analysis, and visualization in production environments.
When diagnosing issues through log analysis, pay attention to:
- Error messages and stack traces
- Abnormal application behavior
- Performance degradation indicators
- Unusual or excessive log entries
By correlating log entries with specific application events, you can pinpoint the root cause of issues and take appropriate corrective actions.
Thread Dump Analysis
In a multi-threaded Java application, thread-related issues are a common source of problems in production environments. Thread dumps provide snapshots of the application's thread state at a specific point in time, allowing you to identify thread contention, deadlocks, and other concurrency-related issues.
To capture a thread dump from a running Java process, you can use tools like jstack
or visualVM. For example, using jstack
:
jstack <pid> > thread_dump.txt
Analyzing the captured thread dump can reveal insights into:
- Blocked or deadlocked threads
- Thread contention
- Thread pool saturation
- Long-running or stuck threads
Understanding the thread behavior can help you diagnose performance bottlenecks and resolve concurrency issues in a production environment.
Monitoring and Profiling
Continuous monitoring and profiling of a production Java application are essential for identifying performance bottlenecks, resource usage patterns, and potential scalability issues. Tools like JConsole, VisualVM, and New Relic offer comprehensive insights into the application's runtime behavior, memory usage, CPU utilization, and other performance metrics.
By monitoring key performance indicators, such as response times, throughput, and error rates, you can proactively detect anomalies and take corrective measures before they impact the user experience.
Profiling tools enable you to analyze the application's runtime behavior, method-level performance, memory allocations, and garbage collection patterns. This level of insight is invaluable for optimizing critical code paths and improving overall application performance in a production environment.
Bringing It All Together
In the high-stakes environment of production, effective debugging is a critical skill for Java developers. By leveraging remote debugging, log analysis, thread dump analysis, and monitoring tools, you can diagnose and resolve issues without disrupting the live system. Incorporating these best practices into your debugging toolkit will empower you to maintain the reliability and performance of your Java applications in any production scenario.