Overcoming Challenges in Continuous Testing
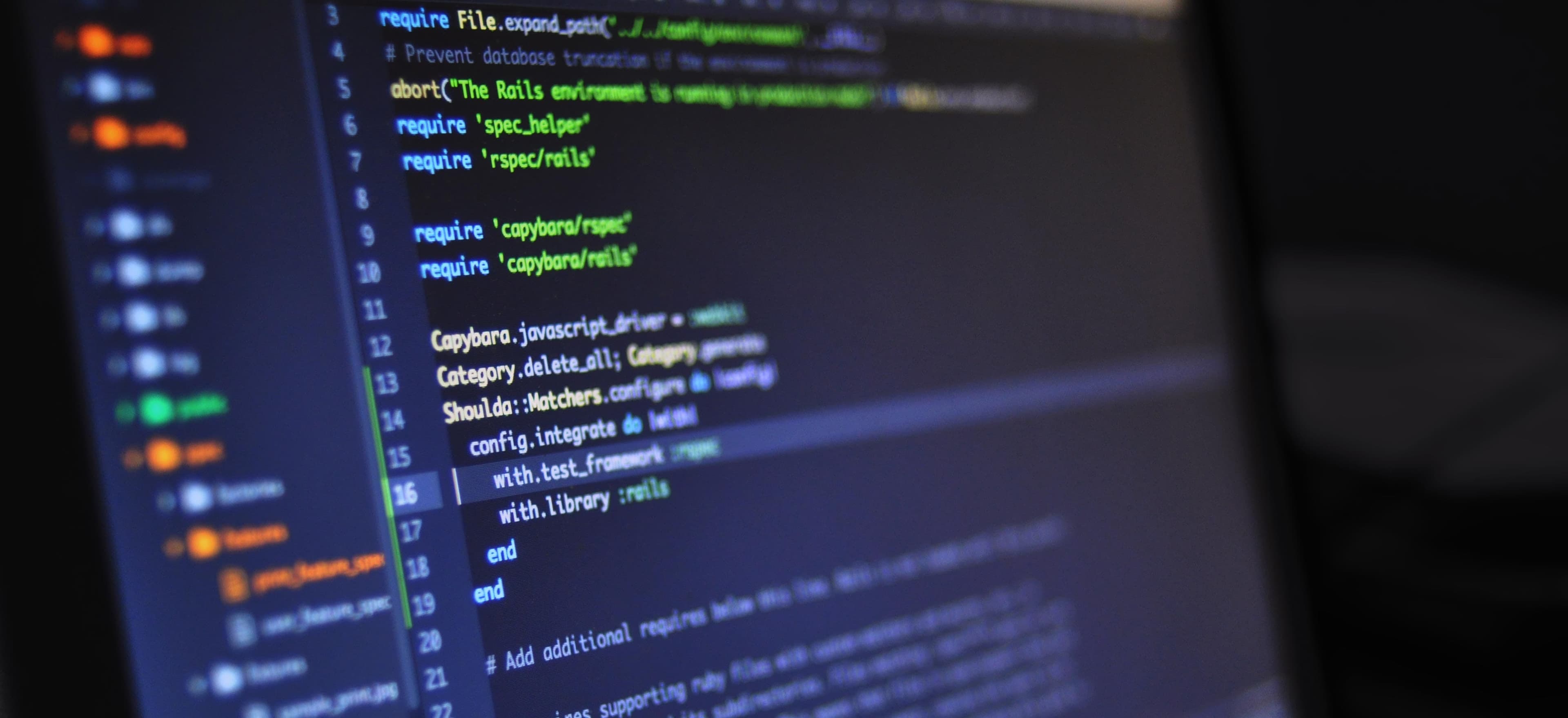
- Published on
The Importance of Continuous Testing in Java Development
In the world of Java development, continuous testing plays a pivotal role in ensuring the quality, reliability, and performance of software applications. By incorporating continuous testing into the development process, developers can identify and address issues early on, leading to higher quality code and faster time to market. In this article, we'll delve into the challenges that developers often face when implementing continuous testing in Java, and explore strategies to overcome these obstacles.
Challenges in Continuous Testing
1. Test Flakiness
One of the most common challenges in continuous testing is test flakiness. This occurs when automated tests produce inconsistent results, leading to false positives or false negatives. Test flakiness can be caused by a variety of factors, such as timing issues, non-deterministic code, or environmental dependencies.
To address test flakiness, developers can employ various techniques such as using explicit wait mechanisms, minimizing reliance on external dependencies, and employing strategies like test retry mechanisms to mitigate the impact of flaky tests.
@Test
public void testAccountBalanceUpdate() {
// Retry the test in case of failure
int maxRetries = 3;
for (int i = 0; i < maxRetries; i++) {
// Test logic here
// Assert statements here
}
}
2. Slow Test Execution
Another challenge in continuous testing is the slow execution of tests, which can significantly impede the feedback loop in the development process. Slow tests can be a result of complex setups, time-consuming database operations, or inefficient test design.
To tackle slow test execution, developers can utilize techniques such as parallel test execution, test data optimization, and leveraging mock objects to simulate external dependencies.
@Test
public void testOrderProcessingTime() {
// Use mock objects to simulate dependencies for faster execution
// Test logic here
// Assert statements here
}
3. Maintaining Test Data
Managing test data, especially in integration testing, poses a considerable challenge for Java developers. Creating and maintaining test data that accurately represents real-world scenarios can be labor-intensive and error-prone.
To address this challenge, developers can employ techniques such as using data generation libraries, leveraging in-memory databases for faster data setup, and employing data reset mechanisms to ensure test data consistency.
@Before
public void setUpTestData() {
// Use data generation libraries to create realistic test data
// Set up test data in an in-memory database
}
Strategies for Overcoming Continuous Testing Challenges
1. Prioritize Test Refactoring
Continuous testing challenges can often be attributed to poorly designed or outdated test suites. By prioritizing test refactoring and regularly reviewing and updating test cases, developers can improve the maintainability and reliability of their test suites.
2. Embrace Automated Test Orchestration
Automated test orchestration tools can help streamline and manage the execution of automated tests, allowing for parallel execution, result aggregation, and integration with continuous integration pipelines. By embracing automated test orchestration, developers can optimize test execution and gain valuable insights into test results.
3. Leverage Containerization for Test Environments
Containerization technologies such as Docker can be leveraged to create consistent and isolated test environments. By containerizing test dependencies and environments, developers can ensure reproducibility and consistency across different testing stages, ultimately mitigating environmental inconsistencies.
Wrapping Up
In the realm of Java development, continuous testing is indispensable for maintaining code quality and delivering high-performance, reliable software. By acknowledging and addressing the challenges associated with continuous testing, developers can foster a culture of quality and agility within their development processes.
Integrating strategies such as prioritizing test refactoring, embracing automated test orchestration, and leveraging containerization can empower Java developers to overcome the hurdles of continuous testing, ultimately contributing to the delivery of robust and resilient software applications.
In conclusion, continuous testing is not without its challenges, but with the right approach and strategies in place, developers can navigate these obstacles and reap the benefits of a solid and efficient testing process.