Handling Image Storage in MySQL Using Struts2 and Hibernate
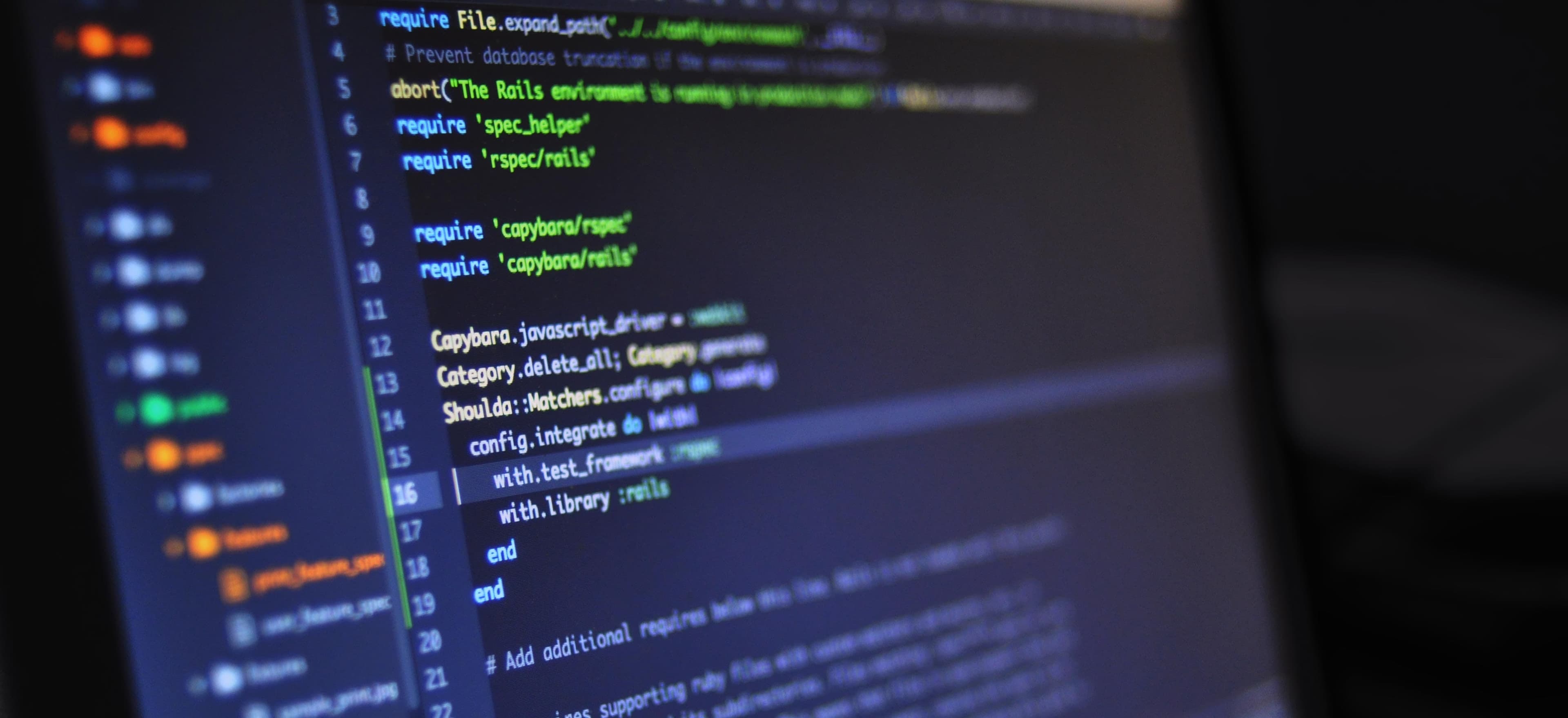
- Published on
Efficient Image Storage in MySQL Database Using Struts2 and Hibernate
In today's web applications, effective image storage and retrieval are a common requirement. Storing images in a MySQL database while using the Struts2 framework and Hibernate ORM can be a powerful combination. By implementing this approach, you can efficiently manage image storage and retrieval while maintaining a structured database. In this blog post, we'll explore how to handle image storage in MySQL using Struts2, Hibernate, and provide code snippets to demonstrate the practical implementation.
Understanding the Requirements
Before diving into the technical implementation, it's crucial to understand the requirements for image storage in a MySQL database:
- Efficient Storage: Images should be stored efficiently in the database to optimize storage and retrieval performance.
- Maintaining Relationships: In a real-world scenario, images are often associated with other entities, such as users, products, or articles. It's essential to maintain these relationships in the database.
- Seamless Retrieval: Retrieving and rendering images should be seamless and performant within the web application.
Setting Up the Environment
To begin with, let's consider a scenario where we have a web application built using the Struts2 framework and Hibernate for ORM. We'll assume that the application already has the necessary configurations for Struts2 and Hibernate.
Database Schema
Before we start, let's define a basic database schema for storing images. For this example, we'll create a table images
with the following schema:
CREATE TABLE images (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
content MEDIUMBLOB,
associated_entity_id INT,
associated_entity_type VARCHAR(50)
-- Additional columns as per specific requirements
);
In this schema, content
is the column where the actual image data will be stored as a MEDIUMBLOB
, and associated_entity_id
along with associated_entity_type
are used to maintain the relationship with other entities in the application.
Storing Images in the Database
Now, let's dive into the code implementation for storing images in the MySQL database using Struts2 and Hibernate.
Handling Image Upload in Struts2
First, we'll create an action class in Struts2 to handle the image upload. We'll use a form to upload the image and then process it in the action class.
public class ImageUploadAction extends ActionSupport {
private File image;
private String imageContentType;
private String imageFileName;
// Getters and Setters
public String execute() {
// Process the uploaded image
return SUCCESS;
}
}
In the JSP, we'll have a form to upload the image:
<s:form action="imageUpload" method="post" enctype="multipart/form-data">
<s:file name="image" label="Image" />
<s:submit value="Upload" />
</s:form>
Once the image is uploaded, we can handle it in the ImageUploadAction
by accessing the image
file and its metadata. We can then pass this data to the service layer for further processing.
Storing Images Using Hibernate
In the service layer, we can utilize Hibernate to store the image in the database. We'll create a method to handle the image storage using Hibernate's session and entity management.
@Transactional
public class ImageService {
public void saveImage(File image, String imageName, Long entityId, String entityType) {
try {
byte[] imageData = Files.readAllBytes(image.toPath());
ImageEntity imageEntity = new ImageEntity();
imageEntity.setName(imageName);
imageEntity.setContent(imageData);
imageEntity.setAssociatedEntityId(entityId);
imageEntity.setAssociatedEntityType(entityType);
sessionFactory.getCurrentSession().save(imageEntity);
} catch (IOException e) {
// Handle the exception
}
}
}
In the above code, we read the content of the uploaded image file using Files.readAllBytes()
and then create an ImageEntity
object to store the image data along with associated metadata.
Retrieving and Displaying Images
Retrieving and displaying images in the web application is equally important. Let's see how we can achieve this using Struts2 and Hibernate.
Fetching Images Using Hibernate
To retrieve images from the database, we can create a method in the ImageService
to fetch the image data based on the associated entity.
public class ImageService {
// ... existing code
public ImageEntity getImageByEntity(Long entityId, String entityType) {
Criteria criteria = sessionFactory.getCurrentSession().createCriteria(ImageEntity.class);
criteria.add(Restrictions.eq("associatedEntityId", entityId));
criteria.add(Restrictions.eq("associatedEntityType", entityType));
return (ImageEntity) criteria.uniqueResult();
}
}
Here, we use the Hibernate Criteria API to fetch the image based on the associated entity ID and type.
Displaying Images in Struts2
In the Struts2 action or JSP, we can use the retrieved image data to render it on the web page.
public class ImageDisplayAction extends ActionSupport {
private Long entityId;
private String entityType;
private byte[] imageData;
// Other getters and setters
public String execute() {
ImageService imageService = new ImageService();
ImageEntity imageEntity = imageService.getImageByEntity(entityId, entityType);
if (imageEntity != null) {
imageData = imageEntity.getContent();
return SUCCESS;
}
return ERROR;
}
}
In the JSP, we can use the retrieved image data to display the image:
<img src="data:image/jpeg;base64, <s:property value="imageData" escape="false" />" />
Using the data:image
URI scheme, we can directly embed the image data within the img
tag.
The Last Word
In this blog post, we have explored the efficient storage of images in a MySQL database using Struts2 and Hibernate. We've discussed the requirements, database schema, image upload handling, image storage using Hibernate, and retrieval and display strategies within a Struts2 web application. By utilizing these techniques, you can effectively manage image storage while maintaining a structured database and seamless image retrieval within your web application. With careful consideration of the database schema and efficient utilization of Hibernate for image storage and retrieval, you can build a robust solution for managing images in your web application.
Remember, the efficient storage and retrieval of images play a crucial role in delivering a rich user experience, making it imperative to implement these techniques thoughtfully in your projects.
As technology and frameworks evolve, it's essential to stay updated with the latest best practices for image handling in web applications. Seamlessly integrating image management with the overall application architecture will contribute to a compelling user experience and optimized performance.
Now that you've gained insights into efficient image storage in MySQL using Struts2 and Hibernate, feel free to implement and further enhance these techniques in your own web applications.
Happy coding!