NFC Tag Not Detected on Android: Troubleshooting Guide
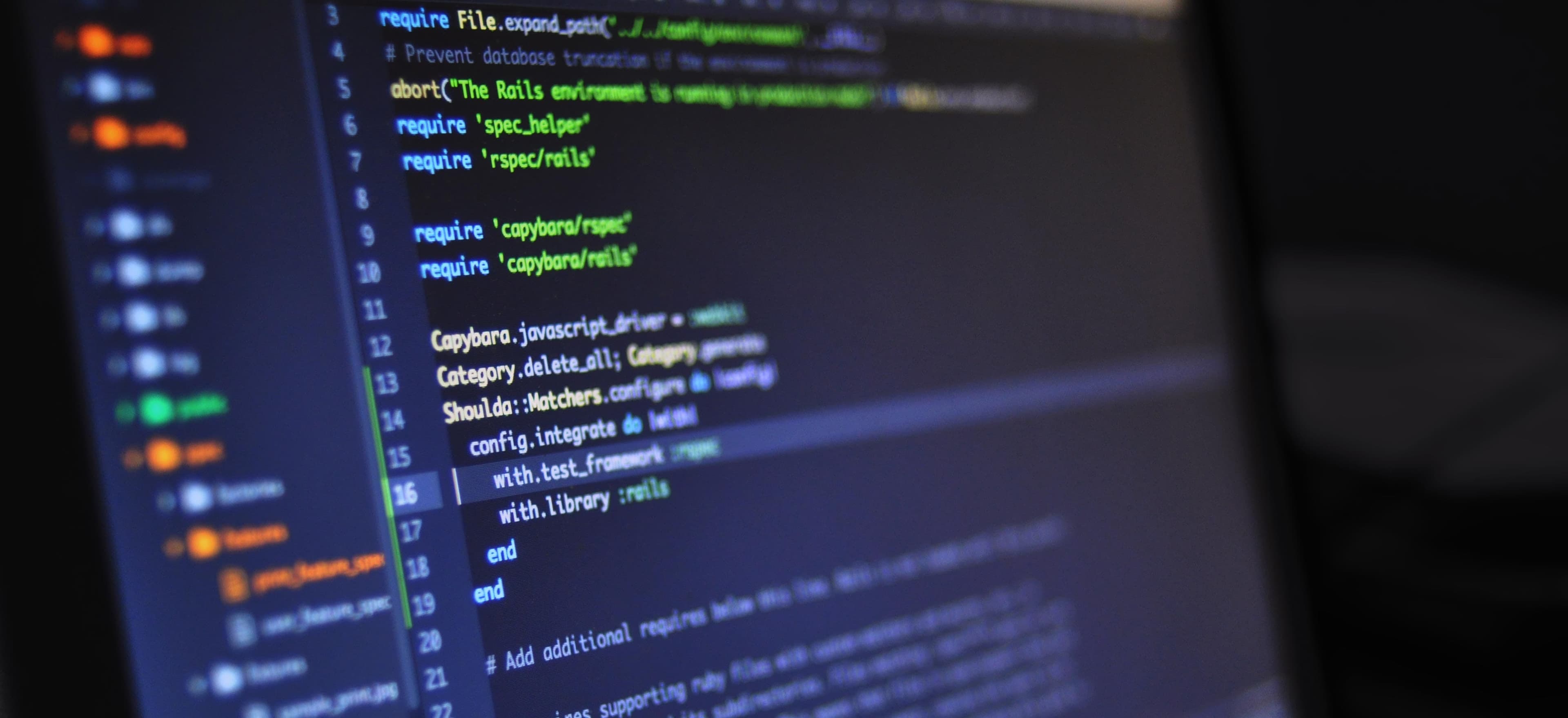
- Published on
Troubleshooting Guide: NFC Tag Not Detected on Android
Near Field Communication (NFC) is a set of communication protocols that enable two electronic devices, one of which is usually a portable device such as a smartphone, to establish communication by bringing them within 4 cm of each other. When developing NFC applications for Android, encountering issues with tag detection is not uncommon. In this troubleshooting guide, we will discuss common reasons why NFC tags may not be detected on Android devices and how to address them.
1. Check NFC Support
One of the initial steps is to ensure that the Android device being used for testing supports NFC. A quick online search or checking the device's specifications can confirm this. Additionally, the NFC feature should be enabled in the device settings.
NfcAdapter nfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (nfcAdapter == null) {
// NFC is not supported
} else {
if (!nfcAdapter.isEnabled()) {
// NFC is supported but not enabled
}
}
2. Verify NFC Tags Placement
Sometimes, the issue with tag detection can simply be related to how the NFC tags are being placed in proximity to the device. It's important to ensure that the tags are positioned within the recommended range and orientation for detection.
3. Handle NDEF Messages Appropriately
When working with NFC tags, especially for handling NDEF (NFC Data Exchange Format) messages, it's crucial to structure and handle the data appropriately. Ensure that the NDEF message is formatted correctly and that the data you are trying to read from the tag is indeed present.
@Override
protected void onNewIntent(Intent intent) {
if (NfcAdapter.ACTION_NDEF_DISCOVERED.equals(intent.getAction())) {
Parcelable[] rawMessages = intent.getParcelableArrayExtra(NfcAdapter.EXTRA_NDEF_MESSAGES);
if (rawMessages != null) {
NdefMessage[] messages = new NdefMessage[rawMessages.length];
for (int i = 0; i < rawMessages.length; i++) {
messages[i] = (NdefMessage) rawMessages[i];
}
}
}
}
4. Check for Interference
External factors such as metal objects or other electronic devices in close proximity can interfere with NFC communication. Ensure that the testing environment is free from such interference that could potentially hamper tag detection.
5. Handle Android Beam Intents
If your application involves Android Beam for transferring data between devices, make sure that the devices being used support Android Beam and that it is enabled. Handling the Android Beam intents correctly is vital for smooth communication between devices.
@Override
public void onResume() {
super.onResume();
NfcAdapter nfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (nfcAdapter != null) {
nfcAdapter.setNdefPushMessageCallback(this, this);
}
}
6. Utilize Foreground Dispatch
Utilizing the foreground dispatch system can help in prioritizing NFC intents over other types of communication, potentially resolving issues related to tag detection.
@Override
public void onResume() {
super.onResume();
nfcAdapter.enableForegroundDispatch(this, pendingIntent, null, null);
}
7. Test with Different NFC Tags
In some cases, the issue may not lie with the code or device, but with the NFC tags themselves. Testing with different tags and ensuring they are functioning properly can help identify any tag-specific issues.
A Final Look
NFC technology presents numerous possibilities for seamless communication and interaction with physical objects, and troubleshooting tag detection issues is crucial for delivering a smooth user experience. By following the troubleshooting steps outlined in this guide and paying attention to the nuances of NFC implementation in Android, developers can mitigate tag detection issues effectively.
Remember, thorough testing and robust error handling are vital components of a successful NFC-based application on the Android platform.
For further insights and best practices, refer to Google's NFC developer guide and stay updated with the latest advancements in NFC technology.