Using Kotlin Classes for 2D Platformer Prototyping
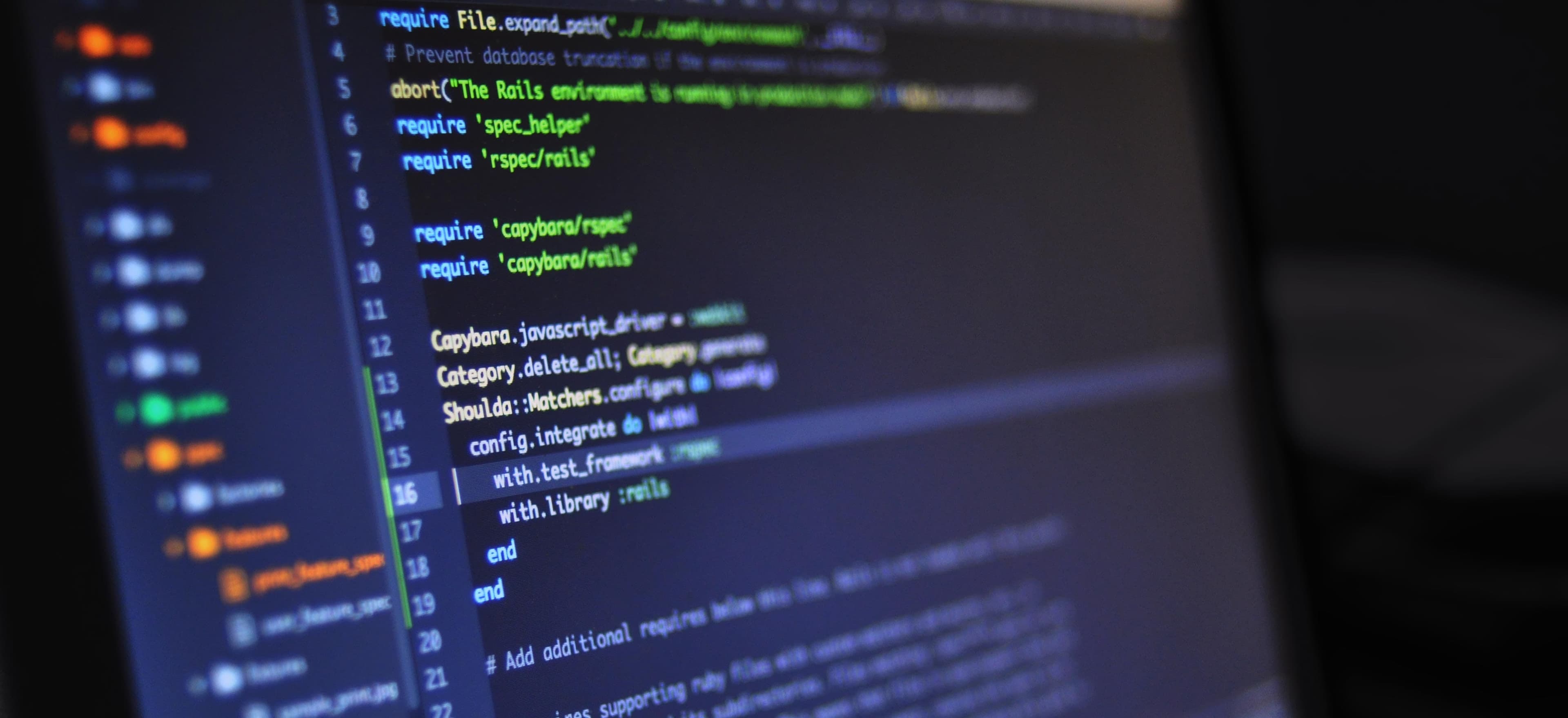
- Published on
Prototyping a 2D Platformer Using Kotlin Classes
When it comes to game development, creating a prototype for your game idea is crucial. Prototyping allows you to quickly test out mechanics, gameplay loops, and overall feel without investing a significant amount of time and effort into the final game. In this article, we'll explore how to use Kotlin classes to create a simple 2D platformer prototype. Kotlin is a modern, expressive language that runs on the Java Virtual Machine, making it a great choice for game development.
Setting Up the Project
Before we dive into the code, let's set up our project. We'll need a Java development environment, such as IntelliJ IDEA, and the Kotlin plugin installed. Once we have our environment ready, we can create a new Kotlin project.
Creating the Player Class
The player is a crucial element in any platformer game. We'll start by creating a Player
class that will represent our player character.
class Player(var x: Int, var y: Int) {
fun move(dx: Int, dy: Int) {
x += dx
y += dy
}
}
In this Player
class, we define two properties x
and y
to represent the player's position. We also have a move
function that allows the player to move by a specified amount in the x and y directions.
Creating the Platform Class
Next, we'll create a Platform
class to represent the ground or platforms in our game.
class Platform(val x: Int, val y: Int, val width: Int, val height: Int) {
fun isCollidingWith(player: Player): Boolean {
return player.x < x + width &&
player.x + PLAYER_WIDTH > x &&
player.y < y + height &&
player.y + PLAYER_HEIGHT > y
}
}
In the Platform
class, we define properties x
, y
, width
, and height
to represent the position and dimensions of the platform. We also have a isCollidingWith
function that checks if the player is colliding with the platform. This function uses basic collision detection logic to determine if the player is overlapping with the platform.
Putting It All Together
Now that we have our Player
and Platform
classes, let's put them together in a simple main function to test our prototype.
fun main() {
val player = Player(100, 300)
val platform = Platform(50, 350, 200, 20)
if (platform.isCollidingWith(player)) {
println("Player is colliding with the platform!")
} else {
println("Player is not colliding with the platform.")
}
player.move(5, 0)
if (platform.isCollidingWith(player)) {
println("Player is colliding with the platform!")
} else {
println("Player is not colliding with the platform.")
}
}
In the main
function, we create an instance of the Player
class and an instance of the Platform
class. We then test for collision between the player and the platform using the isCollidingWith
function. After that, we move the player and test for collision again.
The Closing Argument
In this article, we've used Kotlin classes to create a simple 2D platformer prototype. We've created classes to represent the player and platforms, implemented basic collision detection, and tested our prototype in a simple main function.
Creating prototypes is an essential part of game development as it allows us to iterate quickly on our ideas and test out gameplay mechanics. With Kotlin's expressive syntax and powerful features, it's a great choice for prototyping and developing games.
If you're interested in learning more about game development in Kotlin, check out Korge, a Kotlin-based multiplatform game library, for building games with ease.
Start prototyping your own game today and have fun exploring the world of game development with Kotlin!