Understanding Java EE Concurrency Utilities
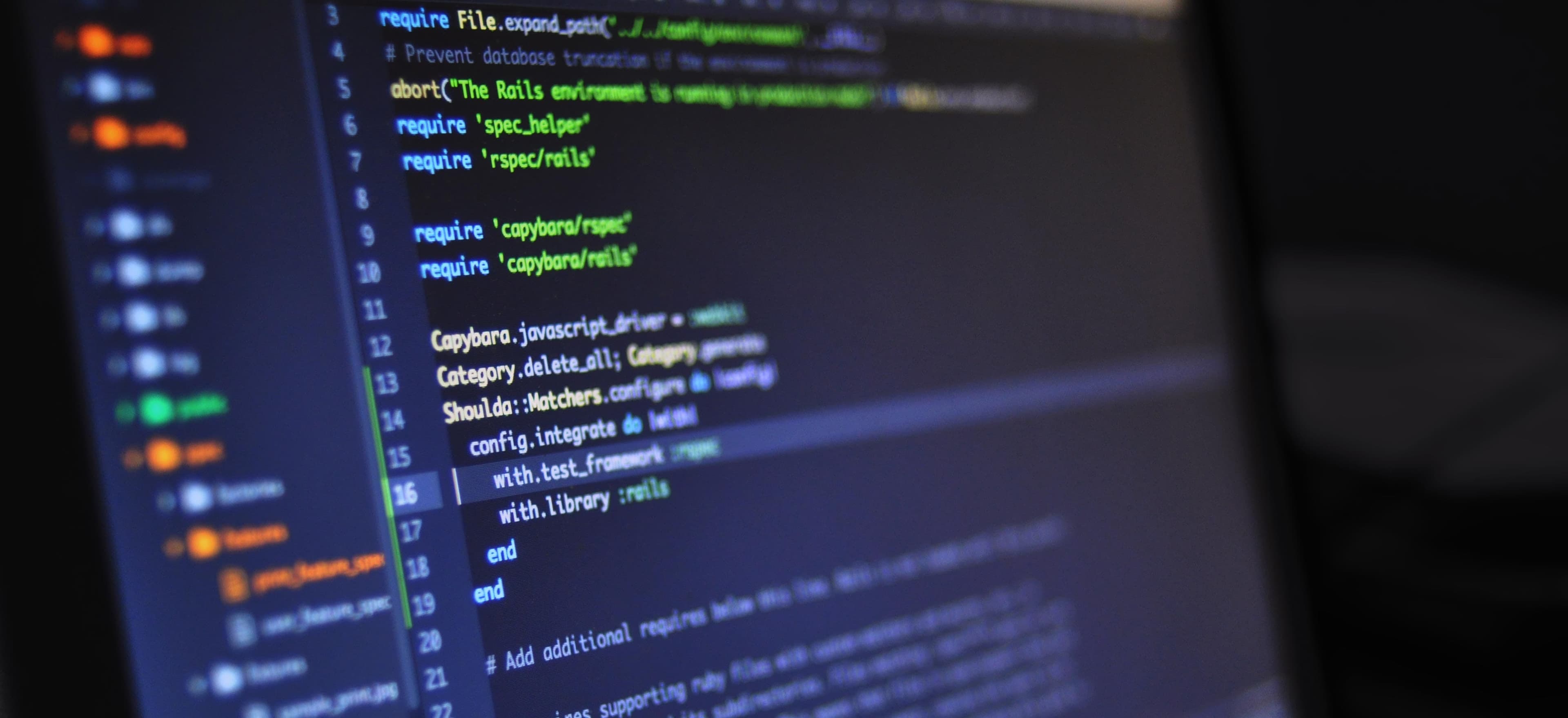
- Published on
Java EE Concurrency Utilities provide a powerful framework for managing concurrent processing in Java Enterprise applications. In this post, we'll explore the essentials of Java EE Concurrency Utilities, focusing on their role in multithreading, as well as how they simplify concurrent programming.
Essentials at a Glance to Java EE Concurrency Utilities
What are Concurrency Utilities?
Concurrency is the ability of a system to handle multiple tasks at the same time. In Java, concurrency is primarily achieved through multithreading. Java EE Concurrency Utilities, introduced in Java EE 7, provide a set of APIs and utilities for concurrent programming in enterprise applications.
Why Concurrency is Important
Concurrency is vital for developing responsive, scalable, and efficient enterprise applications. It allows applications to perform multiple tasks in parallel, leading to improved performance and better resource utilization.
Key Components of Java EE Concurrency Utilities
ManagedExecutorService
The ManagedExecutorService
is a key component of Java EE Concurrency Utilities. It provides an efficient way to execute asynchronous tasks in a managed environment. It is particularly useful in scenarios where the execution of tasks needs to be controlled and monitored within a Java EE environment.
@ManagedBean
public class MyManagedBean {
@Resource
private ManagedExecutorService executorService;
public void runTaskAsynchronously() {
executorService.execute(() -> {
// Asynchronous task logic
});
}
}
In this code snippet, the ManagedExecutorService
is injected using the @Resource
annotation, allowing the execution of tasks in a managed environment.
ContextService
The ContextService
API allows tasks to be executed with a specified context, such as security, transaction, and thread context. This enables tasks to inherit the context of the caller, simplifying the management of contextual information in concurrent operations.
ManagedThreadFactory
The ManagedThreadFactory
provides a way to create and configure threads in a managed environment. This is particularly useful in ensuring that thread creation and management follow the best practices within a Java EE context.
Asynchronous Methods
Java EE Concurrency Utilities introduce the ability to define asynchronous methods in Enterprise JavaBeans (EJB). This allows EJB methods to be executed asynchronously, providing a convenient way to perform non-blocking operations within the EJB environment.
@Stateless
public class MyEJB {
@Asynchronous
public void performAsyncOperation() {
// Asynchronous operation logic
}
}
By annotating a method with @Asynchronous
, the method execution is detached from the calling code, allowing it to run asynchronously.
Best Practices for Using Java EE Concurrency Utilities
Use Thread Pools
When using Java EE Concurrency Utilities, it's important to utilize thread pools provided by ManagedExecutorService
. Thread pools help manage and control the execution of tasks, preventing resource exhaustion and enhancing the overall performance of the application.
Context Propagation
Utilize the ContextService
to propagate the context of the caller when executing tasks asynchronously. This ensures that contextual information, such as security and transaction details, are properly inherited by the asynchronous tasks.
Monitor and Manage Execution
Java EE Concurrency Utilities provide mechanisms to monitor and manage the execution of tasks. It's essential to utilize these capabilities to track the progress of asynchronous tasks, handle exceptions, and manage the lifecycle of threads.
The Last Word
Java EE Concurrency Utilities offer a comprehensive set of tools for developing concurrent applications within the Java EE environment. By leveraging these utilities, developers can effectively manage and control concurrent processing, leading to more efficient and responsive enterprise applications.
In this post, we've explored the key components of Java EE Concurrency Utilities and highlighted best practices for their usage. Embracing these utilities can significantly enhance the performance, scalability, and responsiveness of Java EE applications.
For further reading and in-depth understanding, you can refer to the official Java EE Concurrency Utilities documentation on Oracle's website.
Happy coding!