Overcoming Latency Issues in Cloud IDEs for J2EE Development
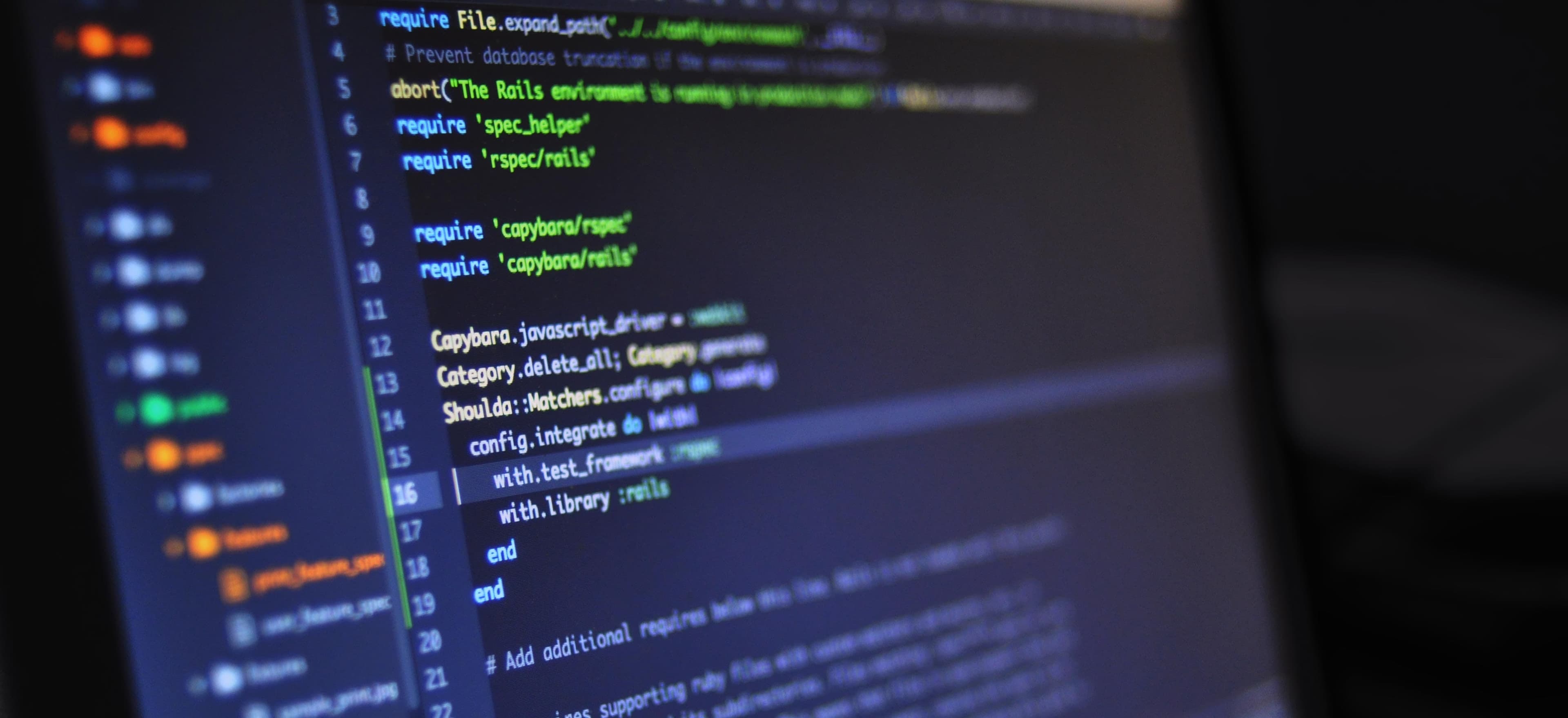
- Published on
Overcoming Latency Issues in Cloud IDEs for J2EE Development
In today's fast-evolving software landscape, many developers are leaning towards Cloud Integrated Development Environments (IDEs). However, a significant challenge remains: latency. This is especially true for Java EE (J2EE) developers who rely on robust frameworks and libraries. In this post, we’ll dive into understanding latency issues specific to Cloud IDEs and discuss practical strategies to mitigate these problems.
Understanding Latency in Cloud IDEs
Latency refers to the time it takes for data to travel from one point to another. In the context of Cloud IDEs, it can originate from various sources:
- Network Issues: The most obvious culprit. Data must travel through the internet, which can introduce delays.
- Processing Delays: Servers in cloud data centers may be processing multiple requests simultaneously.
- Resource Allocation: Insufficient resources allocated to development environments can cause slow response times.
The impact of latency is particularly pronounced in J2EE development. Large applications often require significant resources and continuous interactions between components. As a result, even small delays can significantly disrupt workflows.
Factors Contributing to Latency in Cloud IDEs
To effectively reduce latency, it’s essential to understand its contributing factors. Here are several considerations that every J2EE developer should keep in mind:
1. Distance to Server
The geographical location of the cloud server can dramatically affect latency. The further the data must travel, the longer it takes to reach the destination.
2. Bandwidth Limitations
Internet bandwidth can either constrain or enhance your experience. A slower connection leads to longer load times and affects the performance of IDE features like auto-completion and real-time collaboration.
3. Complex Build Processes
J2EE applications typically involve extensive build processes, which can become a bottleneck if the Cloud IDE doesn't execute builds efficiently.
4. Resource Allocation
Using smaller instances in a cloud environment may save money but can also compromise performance during intensive development activities.
Strategies to Overcome Latency Issues
Here are some effective strategies for mitigating latency when working with Cloud IDEs, specifically tailored for J2EE development.
1. Optimize Your Internet Connection
The speed of development is tightly coupled with the speed of your internet connection. Consider upgrading to a higher bandwidth plan or switching to a wired connection.
2. Choose the Right Cloud Provider
Selecting a cloud provider geographically closer to your workspace can significantly decrease latency. Many providers allow you to choose server locations upon setting up your environment.
3. Employ a Local Development Setup
On occasions when latency becomes a bottleneck, consider a hybrid approach. Use local IDE setups (like IntelliJ IDEA or Eclipse) for heavy lifting tasks and synchronize with the cloud only when necessary. This allows you to benefit from local performance while still taking advantage of the cloud's collaborative features.
// Example: Local development with Git integration for cloud sync.
// Use git to push changes to the cloud once development is stable.
git add .
git commit -m "Your commit message here"
git push origin main
4. Efficiently Configure Dependencies
J2EE applications often have multiple external dependencies. JSON, XML, or various other libraries can exacerbate latency. Tools like Maven or Gradle help in managing these dependencies efficiently. Here's how to properly set up a Maven pom.xml
file:
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
- Why? By using
provided
, it ensures that the dependency is not bundled within the build artifact but will be available on the server, reducing unnecessary upload times.
5. Use Code Optimization Techniques
Efficient code management can also minimize latency. For instance, avoid blocking calls and leverage asynchronous programming. This allows your application to perform tasks non-blocking, hence enhancing responsiveness.
import java.util.concurrent.CompletableFuture;
// Asynchronously fetching data using CompletableFuture
public CompletableFuture<String> fetchData() {
return CompletableFuture.supplyAsync(() -> {
// Simulate long-running task
return "Data fetched!";
});
}
- Why? Non-blocking calls free up resources and allow other operations to continue, leading to faster overall application performance.
6. Leverage Caching Strategies
Implement caching at various levels (client, server, and database). Proper caching strategies reduce the need for repetitive data fetching, significantly improving latency.
import javax.cache.Cache;
import javax.cache.Caching;
// Sample code demonstrating caching
Cache<String, String> cache = Caching.getCache("dataCache");
cache.put("key", "value");
String value = cache.get("key");
- Why? Caching allows for quicker data retrieval, expediting the development process by reducing redundant server calls.
7. Regularly Monitor Performance and Latency
Use tools like New Relic or Google Cloud Monitoring to keep tabs on performance. By analyzing latency trends over time, you can identify bottlenecks and address them preemptively.
Key Takeaways
While latency in Cloud IDEs presents a significant challenge for J2EE developers, there are multiple strategies for overcoming these hurdles. By optimizing internet connections, choosing the right cloud provider, utilizing local development setups, and implementing efficient coding practices, you can create a smoother development experience.
As technology continues to evolve, being proactive in your approach will not only enhance your current workflows but also prepare you for future challenges. The cloud offers remarkable potential; it is up to developers to harness its power effectively.
For further reading, explore resources on Java EE development best practices and cloud performance optimization. Happy coding!