Effective Strategies for Spring Mock MVC Testing
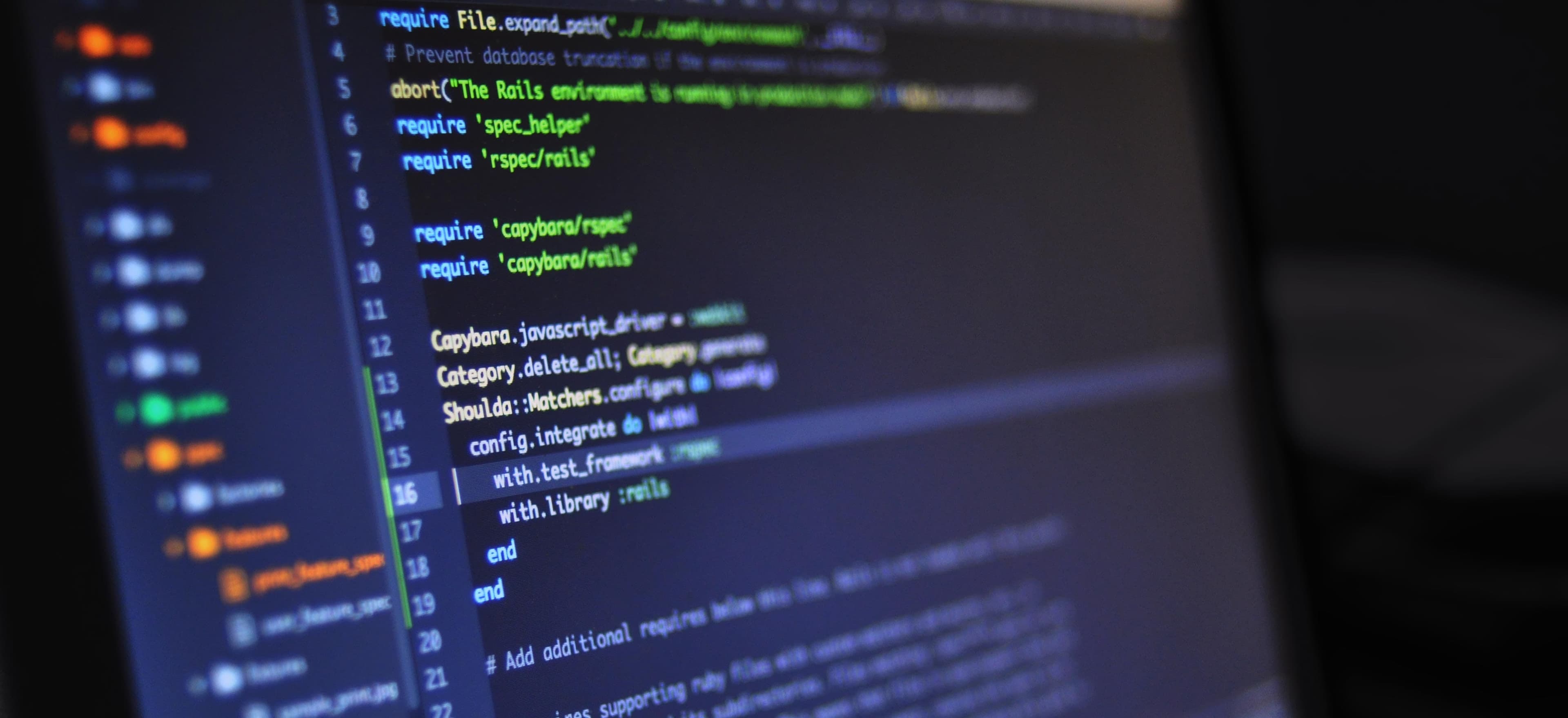
- Published on
Effective Strategies for Spring Mock MVC Testing
When it comes to testing Spring MVC applications, Spring Mock MVC provides a powerful and flexible toolset for validating the behavior of the controllers. With its expressive API and integration with the Spring Testing Framework, it allows for comprehensive testing of the MVC infrastructure. In this article, we will explore some effective strategies for writing robust and maintainable tests using Spring Mock MVC.
1. Understanding the Mock MVC Framework
Before diving into testing strategies, it's crucial to have a solid understanding of the Mock MVC framework. In essence, Mock MVC provides a way to test Spring MVC applications without deploying them to a server. Instead, it simulates the HTTP request-response cycle, allowing you to validate the behavior of your controllers in isolation.
// Example Mock MVC setup
mockMvc = MockMvcBuilders.standaloneSetup(new MyController()).build();
When setting up Mock MVC, you can either standalone setup a specific controller or leverage the full context configuration for comprehensive integration tests.
2. Writing Readable and Maintainable Tests
One of the key challenges in testing is maintaining readability and resilience as the codebase evolves. By following best practices for organizing your tests, you can ensure that they remain clear and understandable.
Utilize Given-When-Then Structure
When writing your test methods, it's beneficial to structure them using the given-when-then pattern. This helps in clearly defining the setup, execution, and assertion phases of the test, making it easier for anyone reading the test to understand its purpose.
@Test
public void givenValidUserId_whenGetUser_thenReturnUser() {
// Given
long userId = 1L;
// When
ResultActions result = mockMvc.perform(get("/users/{id}", userId));
// Then
result.andExpect(status().isOk())
.andExpect(jsonPath("$.name", is("John Doe")));
}
Naming Conventions
Applying descriptive and consistent naming conventions for your test methods and variables contributes significantly to the readability of your tests. This includes using clear and concise method names that reflect the intention of the test.
3. Leveraging Assertions and Matchers
Spring Mock MVC integrates seamlessly with Hamcrest matchers, allowing for expressive and readable assertions. Utilizing these matchers can significantly enhance the clarity and conciseness of your tests.
Example of Matcher Usage
ResultActions result = mockMvc.perform(get("/users/{id}", 1L));
result.andExpect(status().isOk())
.andExpect(jsonPath("$.name", is("John Doe")));
Here, we are using Hamcrest's is
matcher to assert that the returned user's name is "John Doe". This provides a clear and concise assertion compared to traditional assert methods.
4. Thorough Validation of HTTP Responses
In addition to validating the response status and body, it's crucial to thoroughly validate other aspects of the HTTP response, such as headers, cookies, and content types. This ensures that the controller is producing the expected output and adhering to the specified contract.
Validating Response Headers
ResultActions result = mockMvc.perform(get("/users/{id}", 1L));
result.andExpect(header().string("Content-Type", "application/json"))
.andExpect(cookie().exists("session"));
By validating response headers and cookies, you can ensure that the controller is correctly setting these attributes in the HTTP response.
5. Effective Use of Mocking and Stubbing
When testing controllers, it's common to interact with other components, such as services or repositories, which need to be mocked or stubbed. By effectively utilizing mocking frameworks like Mockito, you can isolate the behavior of the controller and focus specifically on testing its responsibilities.
Example of Mocking a Service
// Given
UserService userService = mock(UserService.class);
when(userService.getUserById(1L)).thenReturn(new User(1L, "John Doe"));
MyController myController = new MyController(userService);
// When
ResultActions result = mockMvc.perform(get("/users/{id}", 1L));
// Then
result.andExpect(status().isOk())
.andExpect(jsonPath("$.name", is("John Doe")));
Here, we are mocking the UserService
to return a predefined user when the controller invokes it during the test.
6. Integration with Spring Testing Framework
Spring Mock MVC seamlessly integrates with the Spring Testing Framework, providing access to a range of testing utilities and annotations. Leveraging these capabilities can simplify the testing process and enhance the overall test coverage.
Example of Using @WebAppConfiguration
@WebAppConfiguration
@ContextConfiguration(classes = {AppConfig.class, WebConfig.class})
public class MyControllerTest {
@Autowired
private WebApplicationContext wac;
private MockMvc mockMvc;
@Before
public void setup() {
this.mockMvc = MockMvcBuilders.webAppContextSetup(this.wac).build();
}
// Test methods
}
By using @WebAppConfiguration
and @ContextConfiguration
, you can configure the test to load the appropriate application context and perform full integration tests.
Final Thoughts
In conclusion, Spring Mock MVC offers a rich set of features for testing Spring MVC applications. By following these effective strategies, you can ensure that your tests are readable, maintainable, and comprehensive. Embracing best practices for test organization, assertion usage, validation, and integration with the Spring Testing Framework will elevate the quality of your tests and contribute to the stability and reliability of your Spring MVC applications.
By applying these strategies, you'll be well-equipped to write robust and effective tests for your Spring MVC controllers, contributing to the overall quality and reliability of your applications.
For more in-depth information on Spring Mock MVC, you can refer to the official Spring documentation and the Baeldung blog which offers comprehensive insights into using Spring Mock MVC effectively.