Fixing Deadlocks with ReentrantLock in Java
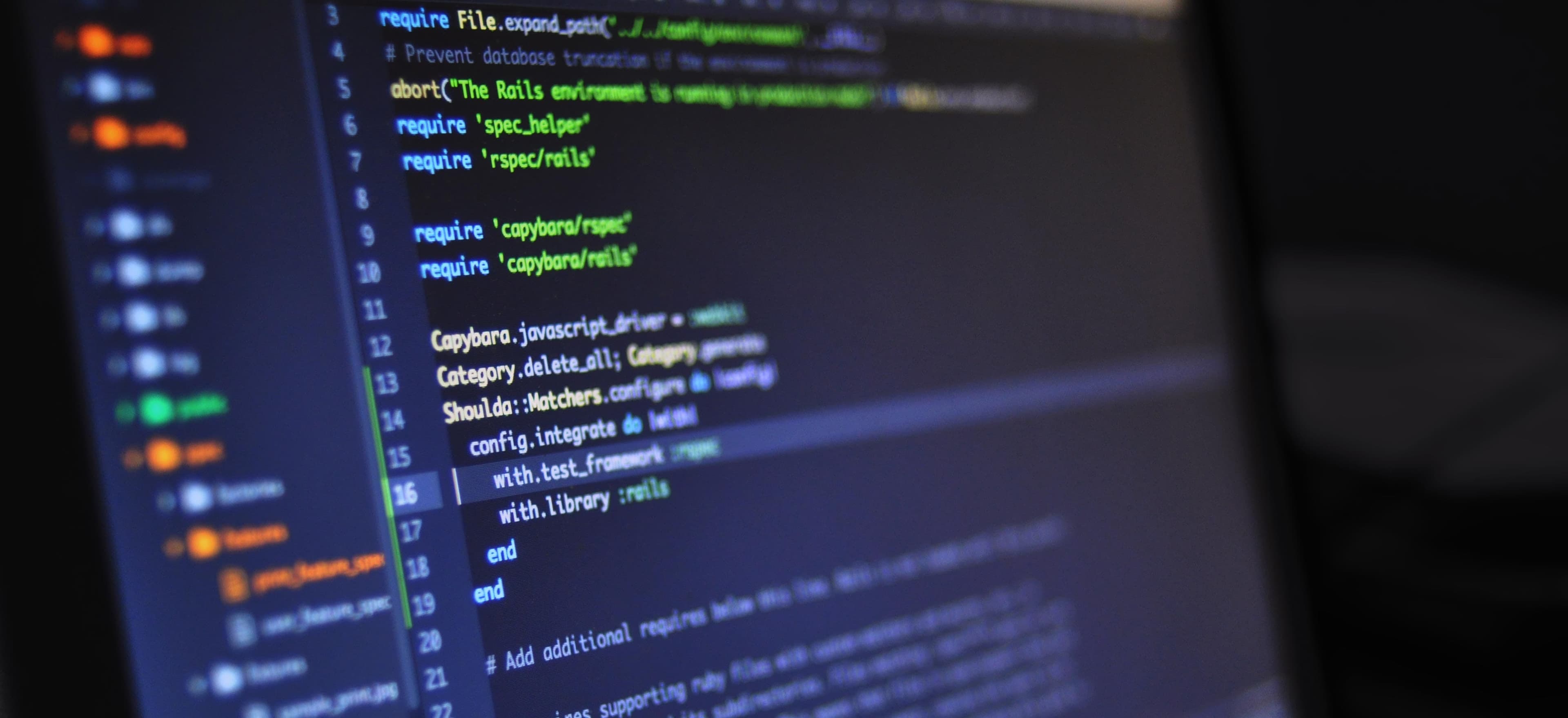
- Published on
Fixing Deadlocks with ReentrantLock in Java
Deadlocks are a common problem in multi-threaded applications. They occur when two or more threads are blocked forever, waiting for each other. In Java, deadlocks can be caused by synchronization issues when using the synchronized
keyword. One way to prevent deadlocks is by using the ReentrantLock
class, which provides a flexible locking mechanism.
In this article, we will explore how to use ReentrantLock
to fix deadlocks in Java applications.
Understanding the Problem
Deadlocks occur when two or more threads are stuck in a circular wait, where each thread is waiting for a resource that's held by another thread. This creates a situation where none of the threads can make progress, leading to a deadlock.
Using ReentrantLock to Prevent Deadlocks
In Java, the ReentrantLock
class provides a more flexible alternative to intrinsic locking with the synchronized
keyword. It allows finer-grained control over locking and can help prevent deadlocks.
Initializing ReentrantLock
import java.util.concurrent.locks.ReentrantLock;
public class DeadlockExample {
private final ReentrantLock lock = new ReentrantLock();
public void performTask() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
}
In the above code, we create a ReentrantLock
instance and use its lock()
and unlock()
methods to control access to the critical section of the code. The lock()
method is called to acquire the lock, and the unlock()
method is called in a finally
block to release the lock, ensuring that the lock is always released even if an exception occurs.
Using tryLock()
One advantage of ReentrantLock
over synchronized blocks is the ability to use the tryLock()
method, which acquires the lock only if it is not held by another thread, avoiding potential deadlocks.
public void performTask() {
if (lock.tryLock()) {
try {
// Critical section
} finally {
lock.unlock();
}
} else {
// Handle the case when the lock couldn't be acquired
}
}
In this example, tryLock()
is used to attempt to acquire the lock. If the lock is not available, the code can handle this situation gracefully, preventing potential deadlocks.
Using Lock Timeout
Another useful feature of ReentrantLock
is the ability to specify a timeout when attempting to acquire the lock, using the tryLock(long time, TimeUnit unit)
method.
public void performTask() {
try {
if (lock.tryLock(2, TimeUnit.SECONDS)) {
try {
// Critical section
} finally {
lock.unlock();
}
} else {
// Handle the case when the lock couldn't be acquired within the specified time
}
} catch (InterruptedException e) {
// Handle the interruption
}
}
By specifying a timeout, we can prevent threads from being blocked indefinitely, mitigating the risk of deadlocks.
To Wrap Things Up
In conclusion, deadlocks are a common issue in multi-threaded applications, but using the ReentrantLock
class in Java can help prevent them. By providing finer-grained control over locking, along with features such as tryLock()
and lock timeouts, ReentrantLock
offers a more flexible and robust solution for managing concurrent access to shared resources.
Let's strive to write code that is deadlock-free and enables smooth execution of multi-threaded applications by leveraging the power of ReentrantLock
in Java.