Enhancing Type Safety with the Checker Framework
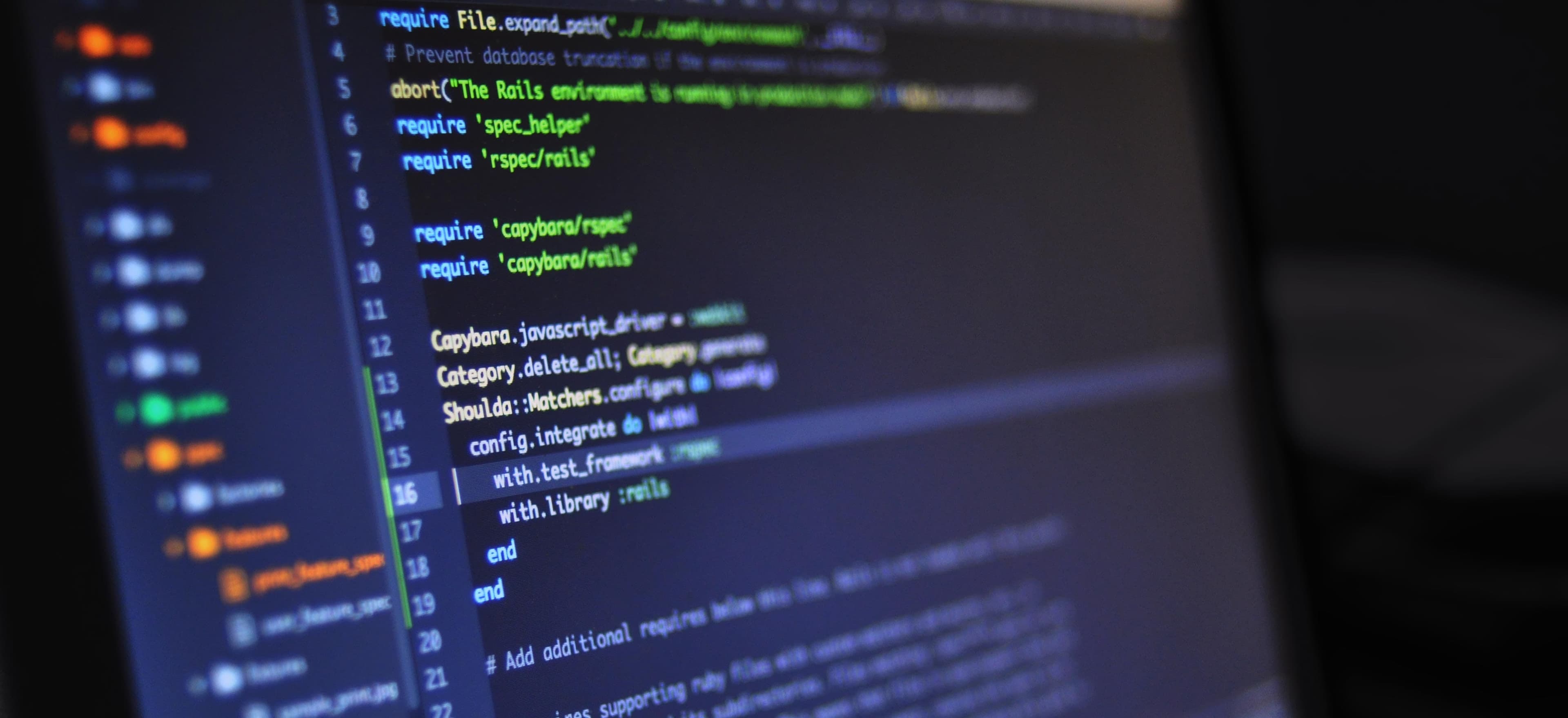
- Published on
Enhancing Type Safety with the Checker Framework
When it comes to writing Java code, type safety is crucial for ensuring the reliability and maintainability of your software. The Java language provides a strong static type system that helps catch many errors at compile-time. However, there are cases where the type system’s built-in checks are not sufficient to capture certain constraints or invariants in your code. This is where the Checker Framework comes in.
What is the Checker Framework?
The Checker Framework is an open-source tool that enhances the Java type system to provide stronger static checking for your code. It allows you to write custom pluggable type checkers that enforce additional compile-time constraints beyond what the Java compiler provides out of the box.
How Does the Checker Framework Work?
At its core, the Checker Framework operates as a compilation-time plugin that extends the Java compiler (javac
) to enforce custom static checks. It leverages Java’s annotation processing capabilities to define and apply custom type qualifiers to your code.
By expressing additional constraints through custom type qualifiers and writing corresponding type checker plugins, you can catch a wider range of errors at compile-time. This ensures that certain properties, such as null safety, resource management, or other domain-specific invariants, are upheld throughout your codebase.
Advantages of Using the Checker Framework
1. Enhanced Type Safety
By leveraging the Checker Framework, you can express and enforce finer-grained constraints in your codebase, leading to enhanced type safety. This allows you to catch a broader range of bugs and potential issues at compile-time, reducing the likelihood of runtime errors.
2. Customizability
The Checker Framework provides a flexible and customizable way to define and enforce your own static checking rules. This enables you to tailor the type system to the specific needs and constraints of your projects, making it a powerful tool for expressing domain-specific invariants.
3. Interoperability
The Checker Framework is designed to work seamlessly with existing Java codebases. You can introduce it gradually into your projects and benefit from its enhanced type checking without having to rewrite large portions of your code.
Getting Started with the Checker Framework
To start leveraging the Checker Framework in your Java projects, follow these steps:
Step 1: Add Checker Framework Dependencies
Include the Checker Framework dependencies in your project’s build configuration. You can typically do this by adding the Checker Framework libraries to your build tool's classpath or as dependencies in your build configuration file.
Step 2: Annotate Your Code
Apply the provided annotations and create custom type qualifiers to express the additional constraints that you want to enforce in your code. For example, you can use annotations to indicate non-null references, resources that require explicit management, or any other domain-specific properties.
Step 3: Write Custom Type Checkers
If the built-in type checkers provided by the Checker Framework do not cover your specific needs, you can write your own custom type checkers. These type checkers will enforce the constraints expressed through your custom type qualifiers.
Step 4: Build and Compile
With the Checker Framework integrated into your project and your code annotated with custom type qualifiers, compile your code using the enhanced Java compiler. The custom type checkers will then verify that your code adheres to the specified constraints.
Example Usage of the Checker Framework
Suppose we have a simple Java class representing a bank account. We want to ensure that the account balance is always non-negative to prevent overdrawing. Using the Checker Framework, we can create a custom type qualifier and a corresponding type checker to enforce this constraint at compile-time.
Example Class with Custom Type Qualifier
import org.checkerframework.checker.index.qual.*;
public class BankAccount {
@NonNegative
private int balance;
public void deposit(@NonNegative int amount) {
// Deposit implementation
balance += amount;
}
public void withdraw(@NonNegative int amount) {
// Withdrawal implementation
if (balance >= amount) {
balance -= amount;
} else {
// Handle insufficient funds error
}
}
}
In this example, we have used the @NonNegative
custom type qualifier to annotate the balance
field and method parameters representing monetary amounts. This indicates that these values should always be non-negative.
Custom Type Checker
We can create a custom type checker that ensures the @NonNegative
constraint is upheld throughout the codebase. For example, the type checker will verify that no method decrements the balance to a negative value and that all arguments passed to methods annotated with @NonNegative
are non-negative.
By following this approach, we can prevent potential runtime errors related to negative account balances at compile-time.
My Closing Thoughts on the Matter
The Checker Framework provides a powerful way to enhance type safety in your Java codebase. By leveraging custom type qualifiers and type checkers, you can express and enforce domain-specific constraints that go beyond the capabilities of the standard Java type system.
It is important to note that while the Checker Framework can greatly improve the reliability and correctness of your code, it is not a silver bullet. Like any static analysis tool, it has its limitations, and it is crucial to use it as part of a comprehensive testing and validation strategy.
In summary, the Checker Framework offers a valuable tool for developers seeking to bolster their code’s type safety and ensure that it adheres to specific domain constraints.
To delve deeper into this topic, you can explore the Checker Framework’s official documentation and sample projects that demonstrate its capabilities.
Remember, type safety matters, and the Checker Framework is here to help you take it to the next level. Happy coding!