Drools Business Rule Engine Optimization Techniques
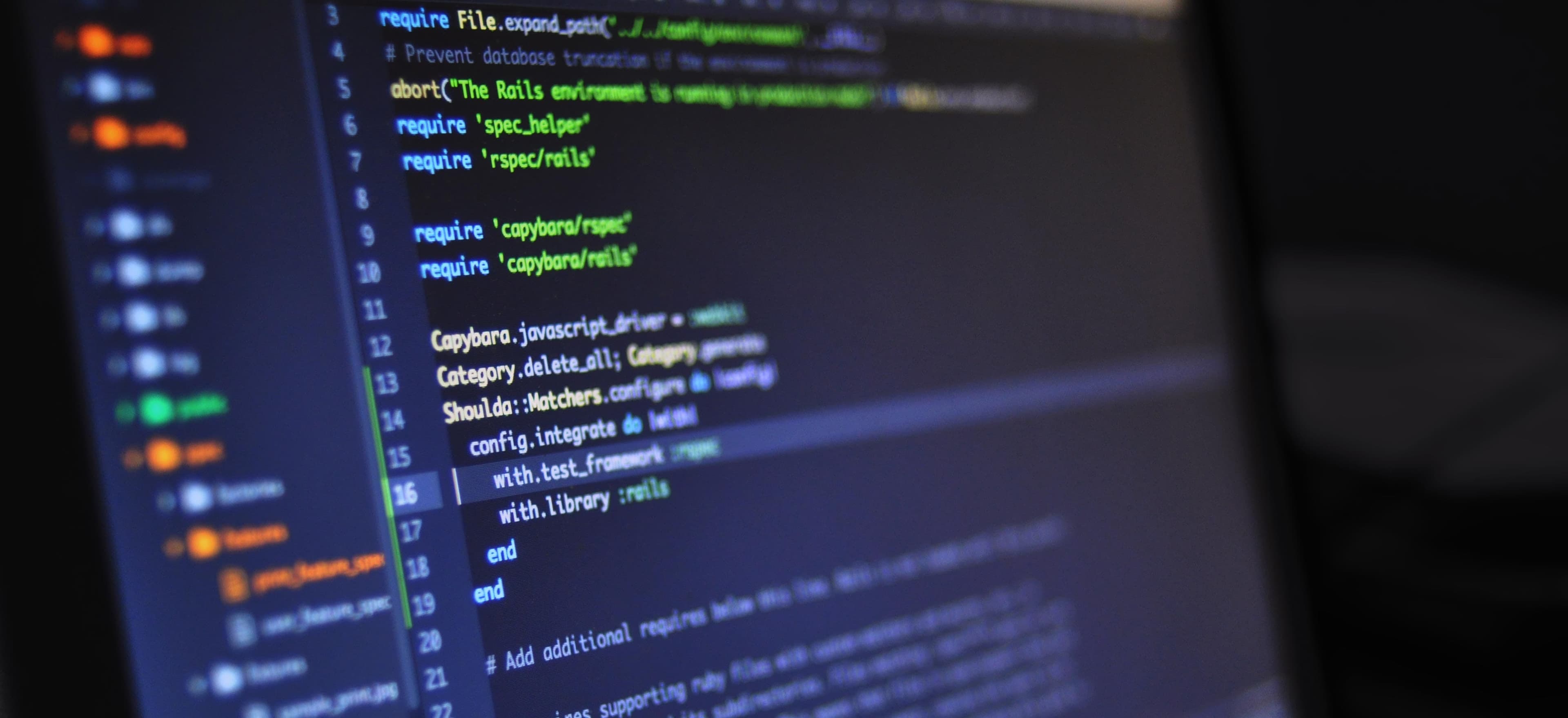
- Published on
Optimizing Drools Business Rule Engine in Java
When it comes to implementing business rules and decision logic in a Java application, Drools Rule Engine stands out as a powerful and flexible solution. However, to ensure optimal performance and maintainability, it's essential to employ certain optimization techniques. In this post, we'll explore some effective strategies to optimize the Drools Business Rule Engine in Java.
Understanding Drools Rule Engine
Before diving into optimization techniques, let's have a brief understanding of Drools Rule Engine. Drools is a widely-used open-source rules engine that allows developers to define and execute business rules in their applications. These rules are typically defined using a domain-specific language (DSL) or with the Drools Rule Language (DRL).
Rule Compilation
One of the key optimization techniques for Drools is rule compilation. By pre-compiling rules, you can significantly reduce the overhead of parsing and interpreting rules every time they are executed. This can be achieved using the Drools compiler, which translates the rule definitions into executable objects. Let's take a look at an example of how to compile rules in Drools:
KieServices kieServices = KieServices.Factory.get();
KieFileSystem kfs = kieServices.newKieFileSystem();
kfs.write("src/main/resources/myrules.drl", myRulesDefinition);
KieBuilder kieBuilder = kieServices.newKieBuilder(kfs).buildAll();
In this example, we use the KieServices
to create a new KieFileSystem
and write the rule definitions to it. Then, we use the KieBuilder
to build all the resources in the file system, which triggers the compilation process. By pre-compiling rules during the build phase, you can ensure faster rule execution at runtime.
Fact Model Optimization
Another important aspect of optimizing Drools is to carefully design the fact model. The fact model represents the objects or data on which the rules are applied. By optimizing the fact model, you can minimize the complexity and improve the efficiency of rule evaluation. Here are some tips for optimizing the fact model:
- Use immutable objects to ensure thread safety and avoid unnecessary state changes.
- Implement proper hashCode and equals methods for fact objects to enhance rule matching performance.
- Avoid deeply nested object graphs, as they can lead to increased memory consumption and slower rule evaluation.
Indexing
Indexing plays a crucial role in improving the performance of rule execution. Drools provides the concept of indexing to quickly locate relevant facts during rule evaluation. By defining indexes on fields that are frequently used in rule conditions, you can speed up the matching process. Let's consider an example of indexing in Drools:
rule "HighRiskCustomer"
when
$customer : Customer(age > 65)
then
// Rule actions
end
In this example, indexing the 'age' field of the Customer
object can improve the efficiency of the rule by allowing Drools to quickly locate customers matching the specified age criterion.
Monitoring and Profiling
To ensure the effectiveness of optimization efforts, it's essential to monitor and profile the performance of the Drools Rule Engine. This can be achieved using various profiling tools and monitoring techniques. By identifying the most time-consuming and resource-intensive aspects of rule execution, you can pinpoint areas for further optimization. Tools like VisualVM, YourKit, and Java Mission Control can be valuable for profiling and optimizing the performance of Drools-based applications.
Closing Remarks
Optimizing the Drools Rule Engine in Java is crucial for achieving high-performance and efficient rule execution. By employing techniques such as rule compilation, fact model optimization, indexing, and monitoring, developers can ensure that their Drools-based applications deliver optimal performance and scalability. With careful attention to these optimization strategies, you can harness the full potential of Drools Rule Engine in your Java applications.
Incorporating Drools into your Java projects can greatly enhance decision-making capabilities. However, it's important to consider optimization techniques such as rule compilation, fact model optimization, and indexing to ensure the best performance. By understanding these key principles and implementing them effectively, you can maximize the efficiency and maintainability of your Drools-based applications.
Now that you've learned about optimizing Drools Rule Engine in Java, you might want to explore more about Drools and its applications in real-world scenarios. You can read more about the best practices and real-world use cases of Drools on Drools official documentation.
In addition to exploring the official documentation, you may also find it helpful to dive into in-depth tutorials and case studies on Drools. One excellent resource for this is Baeldung's guide to Drools. This guide offers comprehensive tutorials and examples that can further enhance your understanding of Drools and its optimization techniques.
By incorporating these optimization techniques into your Drools-based applications, you can unlock the full potential of the Rule Engine and ensure optimal performance for your business rules.