Streamlining E2E Testing With Effective Tools
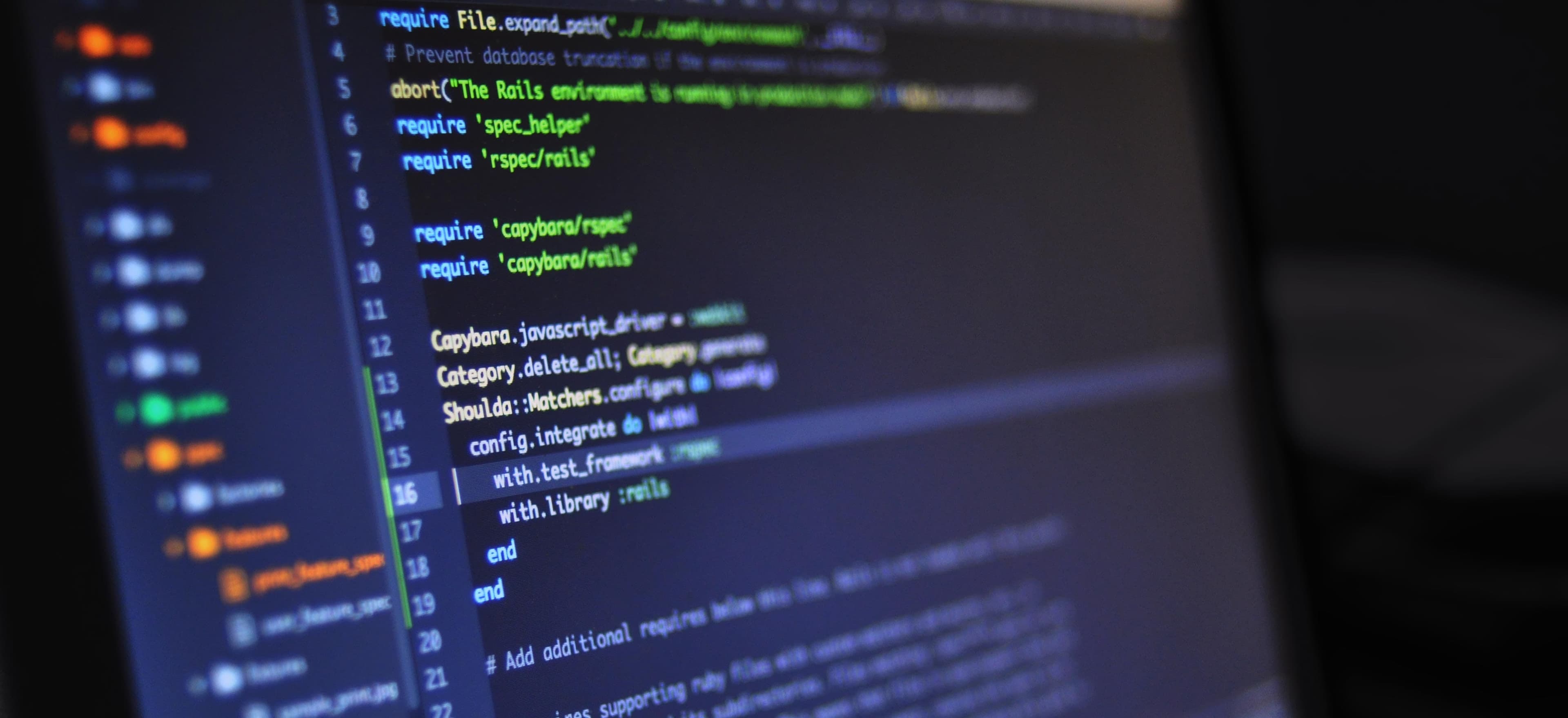
- Published on
The Importance of Effective E2E Testing in Java
End-to-End (E2E) testing is crucial for validating the behavior of an application from start to finish. In the world of Java development, E2E testing plays a pivotal role in ensuring the quality and reliability of software applications. However, conducting E2E testing can be a complex and time-consuming process, especially when done manually.
This is where the utilization of effective tools becomes essential. In this article, we will explore the significance of E2E testing in Java and discuss how leveraging the right tools can streamline the E2E testing process, leading to increased efficiency and higher confidence in the application's functionality.
Understanding E2E Testing in Java
E2E testing involves testing an application's workflow from the beginning to the end, just as a user would experience it. In the Java ecosystem, E2E testing typically encompasses testing the frontend user interface, the backend API interactions, and the integration of various components within the application.
The Challenges of Manual E2E Testing
Manual E2E testing is not only labor-intensive but also prone to human error. As applications grow in complexity, manually testing every possible user interaction and workflow becomes unmanageable and unsustainable. Additionally, manual testing may not cover the entire application surface, leading to potential blind spots in testing coverage.
Streamlining E2E Testing with Automation
Automation is the key to addressing the challenges posed by manual E2E testing. By automating E2E tests, developers can ensure thorough test coverage, improve testing reliability, and significantly reduce the time and effort required for testing.
Leveraging Effective Tools for E2E Testing in Java
Selenium
Selenium is a widely used open-source tool for automating web browsers. It provides a rich set of tools and APIs for interacting with web elements, simulating user actions, and performing E2E testing of web applications. Selenium WebDriver, in particular, enables developers to write automated tests that simulate user interactions across different browsers.
Sample Selenium WebDriver Code Snippet in Java:
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
WebElement element = driver.findElement(By.id("elementId"));
element.sendKeys("sample_text");
The above code snippet demonstrates the use of Selenium WebDriver in Java to open a webpage, locate an element by its ID, and perform a simulated user action of entering text into the element.
Cucumber
Cucumber is a powerful tool for behavior-driven development (BDD) that enables writing E2E tests in a human-readable, domain-specific language. It allows for collaboration between non-technical and technical stakeholders by expressing test scenarios in plain language using Gherkin syntax.
Sample Cucumber Feature File:
Feature: Login Functionality
As a registered user
I want to be able to log in to the system
Scenario: Successful Login
Given I am on the login page
When I enter my username and password
And click on the login button
Then I should be redirected to the dashboard
The above feature file outlines a test scenario for the login functionality using Cucumber's Gherkin syntax, making it readable and understandable for all stakeholders.
RestAssured
For E2E testing of RESTful APIs in Java, RestAssured is a popular library that simplifies API testing by providing a fluent interface for making HTTP requests and validating responses. It integrates seamlessly with existing Java testing frameworks such as JUnit and TestNG.
Sample RestAssured Test in Java:
@Test
public void verifyStatusCode() {
given()
.when().get("https://api.example.com/users")
.then()
.assertThat()
.statusCode(200);
}
The above test uses RestAssured in Java to send a GET request to the specified API endpoint and validate that the response status code is 200.
By incorporating tools like Selenium, Cucumber, and RestAssured into the E2E testing strategy, Java developers can establish a robust testing infrastructure that covers both the frontend and backend aspects of their applications, ensuring comprehensive test coverage and early detection of issues.
In Conclusion, Here is What Matters
Effective E2E testing is paramount in Java application development to deliver high-quality, reliable software. By embracing automation and leveraging powerful tools like Selenium, Cucumber, and RestAssured, developers can streamline the E2E testing process, minimize manual effort, and enhance the overall quality and robustness of their applications. As the software industry continues to evolve, the role of E2E testing and the tools supporting it will remain instrumental in delivering exceptional user experiences and maintaining the integrity of Java applications.
Checkout our other articles