Choosing the Right Automation Testing Tool: Key Considerations
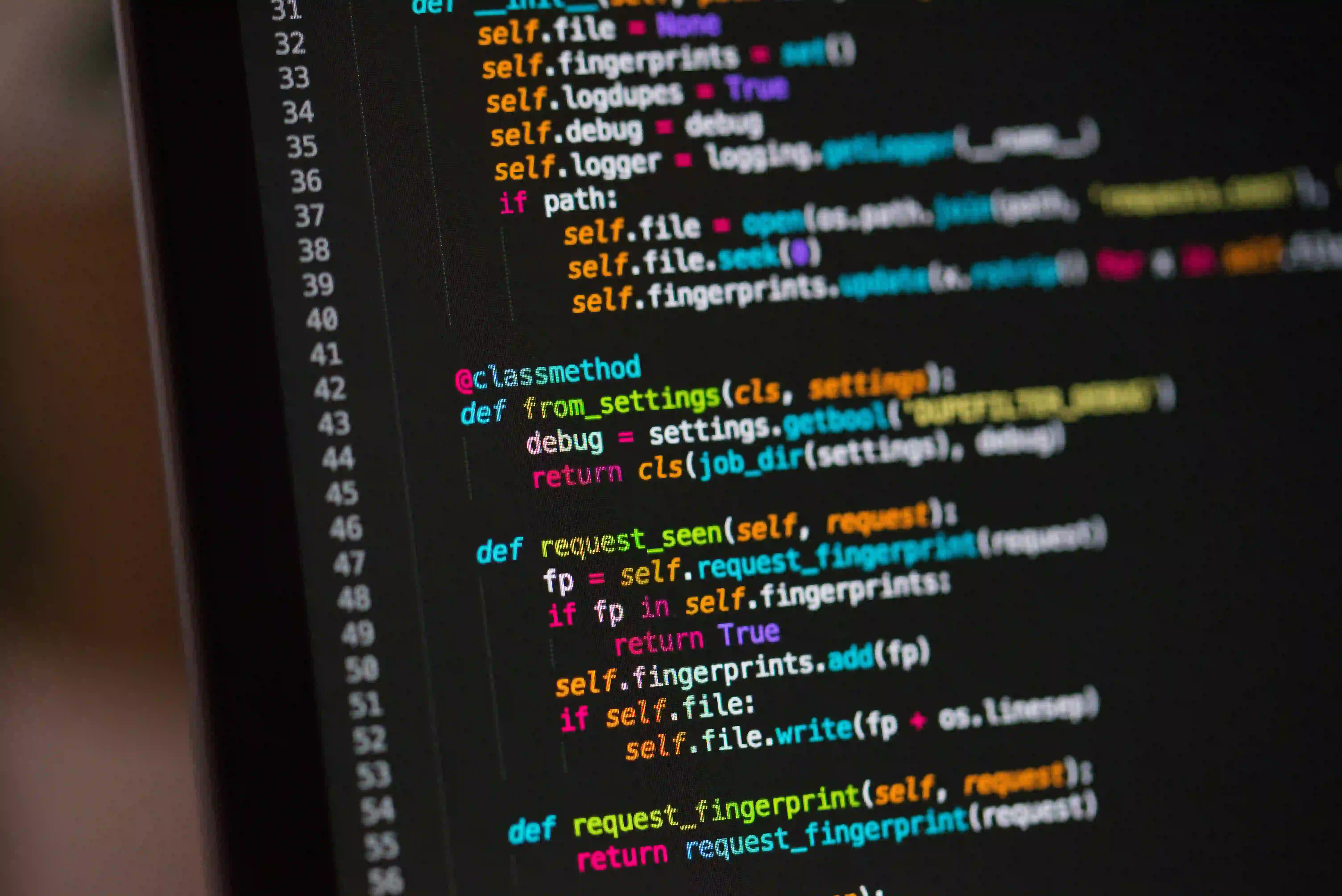
Choosing the Right Automation Testing Tool: Key Considerations
In the world of software development, the importance of automation testing cannot be overstated. It not only helps in saving time but also ensures the efficiency and reliability of the software. When it comes to automation testing, selecting the right tool is crucial for the success of the testing process. There are numerous automation testing tools available in the market, each with its own set of features and capabilities. In this blog post, we will discuss the key considerations for choosing the right automation testing tool, with a focus on Java-based tools.
Understanding the Requirements
The first step in choosing the right automation testing tool is to understand the specific requirements of the project. Consider factors such as the type of application being tested (web, mobile, desktop), compatibility with different operating systems and browsers, support for different testing frameworks, integration with CI/CD pipelines, and scalability. By clearly defining the requirements, you can narrow down the list of potential automation testing tools.
Language Support
For Java-based projects, it is essential to choose an automation testing tool that supports Java as the programming language. Tools such as Selenium and Appium are popular choices for automation testing of web and mobile applications, respectively, and provide extensive support for Java. Java’s widespread usage in the industry makes it a sensible choice for test automation, as it offers a rich ecosystem of libraries and frameworks.
@Test
public void verifyHomePageTitle() {
// Instantiate a new WebDriver
WebDriver driver = new ChromeDriver();
// Open the website
driver.get("https://www.example.com");
// Get the title of the webpage
String title = driver.getTitle();
// Verify the title
assertEquals("Example Domain", title);
// Close the browser
driver.quit();
}
In the code snippet above, we can see the simplicity and readability of Java when used with Selenium for verifying the title of a webpage.
Community Support and Documentation
An important factor to consider is the availability of a strong community and extensive documentation for the automation testing tool. A tool with a large user community often means more support, resources, and a vibrant ecosystem of plugins and extensions. Comprehensive documentation is also crucial for quick adoption and troubleshooting. Tools like JUnit and TestNG, which are widely used in the Java community, have robust documentation and active user forums.
Integration Capabilities
Automation testing tools should seamlessly integrate with other tools and technologies in the software development lifecycle. Integration with popular CI/CD platforms such as Jenkins, Travis CI, and TeamCity is a common requirement. Additionally, the ability to integrate with version control systems like Git for test code management is essential. Tools like JUnit and TestNG have excellent integration capabilities with CI/CD tools, making them suitable choices for Java projects.
@AfterClass
public static void tearDown() {
// Perform cleanup activities such as closing browser sessions
// This method will be executed after all test methods have run
}
The @AfterClass
annotation in TestNG allows for the execution of cleanup activities after all test methods have been executed, showcasing its integration capabilities within the testing framework.
Parallel Execution Support
In modern software development, the ability to run tests in parallel is crucial for reducing test execution time and achieving faster feedback. An automation testing tool that supports parallel execution of tests across different browsers and devices can significantly improve the overall testing process. TestNG provides built-in support for parallel execution, allowing tests to run concurrently and accelerating the test suite’s completion.
Reporting and Analytics
Effective reporting and analytics are essential for gaining insights into the test results and identifying potential areas of improvement. Automation testing tools with built-in reporting capabilities, such as generating HTML reports with detailed test outcomes, help in tracking the test execution and identifying any issues. Additionally, integration with analytics tools or platforms for monitoring test coverage and performance is valuable for continuous improvement. Tools like ExtentReports in the Java ecosystem provide customizable reporting features for test results visualization.
ExtentReports extent = new ExtentReports("E:\\ExtentReports\\TestReport.html", true);
ExtentTest test = extent.startTest("Test Title", "Test Description");
test.log(Status.PASS, "Step details...");
extent.endTest(test);
extent.flush();
The code snippet above demonstrates the use of ExtentReports to create detailed HTML test reports, providing valuable insights into the test execution process.
Cost and Licensing
While there are many open-source automation testing tools available, there are also commercial tools with advanced features and support. It is important to consider the cost and licensing model of the chosen automation testing tool, especially for enterprise-level projects. Additionally, understanding the support and maintenance services offered by commercial vendors is crucial for long-term sustainability.
To Wrap Things Up
In conclusion, choosing the right automation testing tool is a critical decision that can significantly impact the quality and efficiency of the software testing process. For Java-based projects, factors such as language support, community backing, integration capabilities, parallel execution support, reporting features, and cost considerations play a key role in the selection process. By carefully evaluating these factors and aligning them with the specific requirements of the project, teams can make an informed decision in selecting the most suitable automation testing tool.
Remember, the ultimate goal of automation testing is to enhance the overall software quality and accelerate the delivery of reliable software products. With the right automation testing tool in place, teams can achieve these goals effectively and efficiently.
To learn more about automation testing with Java, check out the Selenium official website and the TestNG documentation. Happy testing!