Optimizing Game Performance in JavaFX
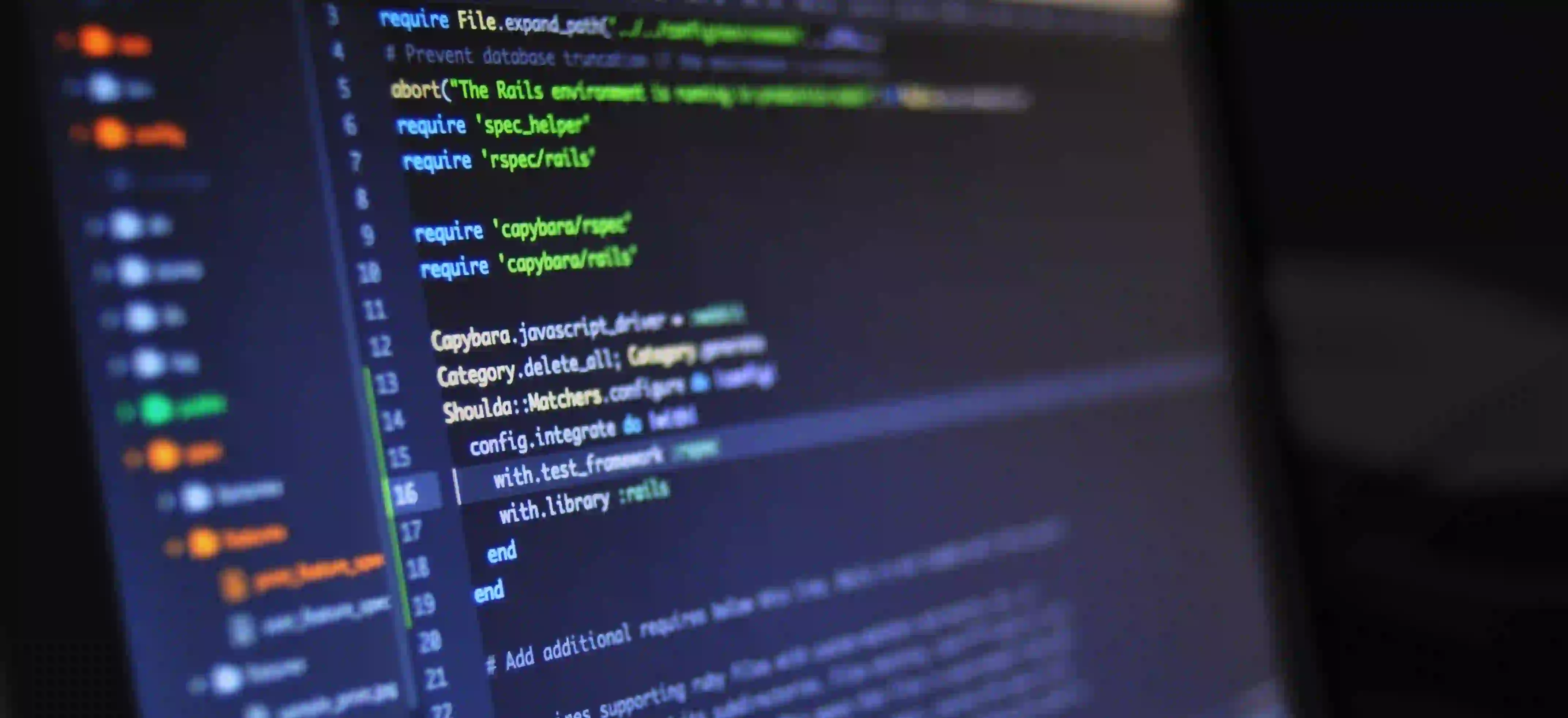
Maximizing Game Performance in JavaFX
When it comes to game development in Java, performance optimization is a pivotal aspect of creating a seamless player experience. JavaFX, with its powerful graphics and multimedia capabilities, provides a solid foundation for building games, but achieving optimal performance requires a keen understanding of its features and nuances. In this post, we'll explore various techniques and best practices for maximizing game performance in JavaFX.
Leveraging Hardware Acceleration
JavaFX leverages hardware acceleration to improve rendering performance. By default, it utilizes the graphics processing unit (GPU) for rendering, resulting in smoother animations and better overall performance. However, it's essential to ensure that hardware acceleration is enabled to fully benefit from this capability.
Enabling hardware acceleration in JavaFX is straightforward. You can do so by setting the system property -Dprism.order=sw
to d3d
for DirectX or es2
for OpenGL. This tells JavaFX to prioritize hardware-accelerated rendering over software rendering.
System.setProperty("prism.order", "d3d");
By explicitly setting the prism.order
property, you can ensure that your game takes full advantage of hardware acceleration, leading to improved performance.
Efficient Memory Management
Memory management is critical for game performance, especially in Java where automatic memory management is a core feature. Efficient memory usage can prevent issues like garbage collection pauses, which can cause noticeable stuttering in games.
One approach to efficient memory management is object pooling. By reusing objects instead of creating and discarding them frequently, you can reduce memory allocation overhead and minimize the frequency of garbage collection cycles.
Let's take a look at an example where object pooling can be beneficial:
// Object pooling for bullets
class BulletPool {
private List<Bullet> availableBullets = new ArrayList<>();
private List<Bullet> inUseBullets = new ArrayList<>();
public Bullet getBullet() {
if (availableBullets.isEmpty()) {
// Create a new bullet only if no bullets are available for reuse
return new Bullet();
} else {
Bullet bullet = availableBullets.remove(availableBullets.size() - 1);
inUseBullets.add(bullet);
return bullet;
}
}
public void returnBullet(Bullet bullet) {
inUseBullets.remove(bullet);
availableBullets.add(bullet);
}
}
In this example, instead of creating a new Bullet
object each time one is needed, the BulletPool
reuses existing bullet objects, reducing the overhead of object creation and garbage collection.
Multithreading for Performance
Multithreading can significantly enhance game performance by offloading certain tasks to separate threads, preventing the main rendering thread from being overloaded. However, it's essential to use multithreading judiciously, as improper multithreading can lead to synchronization issues and other complexities.
For computationally intensive tasks, such as pathfinding algorithms or complex simulations, using separate threads can prevent the game loop from being bogged down. Java provides robust support for multithreading through classes like Thread
and ExecutorService
.
// Example of using ExecutorService for multithreading
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.submit(() -> {
// Perform computationally intensive task
});
executor.shutdown();
In this example, the ExecutorService
is used to submit a task for concurrent execution, effectively utilizing multiple threads to improve performance without directly managing thread creation and lifecycle.
Optimized Rendering with JavaFX
JavaFX offers various tools and techniques for optimizing rendering performance. One such technique is using the Canvas
node for custom rendering operations. By directly manipulating pixels on a Canvas
, you can achieve efficient and tailored rendering for game graphics.
// Example of using Canvas for custom rendering
Canvas canvas = new Canvas(width, height);
GraphicsContext gc = canvas.getGraphicsContext2D();
gc.setFill(Color.RED);
gc.fillRect(0, 0, width, height);
In this example, the Canvas
and GraphicsContext
classes are used to perform custom rendering operations, leveraging low-level pixel manipulation for optimal performance.
Profiling and Benchmarking
Profiling and benchmarking your game is crucial for identifying performance bottlenecks and tracking improvements. Tools like Java Mission Control and VisualVM provide deep insights into memory usage, CPU utilization, and other critical performance metrics.
By profiling your game, you can pinpoint areas that require optimization and measure the impact of performance-enhancing techniques. This iterative approach allows for informed decision-making and continuous refinement of your game's performance.
Final Thoughts
Optimizing game performance in JavaFX encompasses a blend of hardware utilization, memory management, multithreading, rendering optimization, and meticulous analysis through profiling. By leveraging these techniques and best practices, you can create JavaFX games that deliver a responsive and immersive experience for players.
In the ever-evolving landscape of game development, performance optimization remains a fundamental pursuit, and in JavaFX, the synergy of efficient coding and leveraging platform capabilities is key to achieving exceptional game performance.
To explore further on JavaFX game development and additional optimization strategies, you can refer to the comprehensive JavaFX documentation, which provides detailed insights into the capabilities and best practices for JavaFX game development.